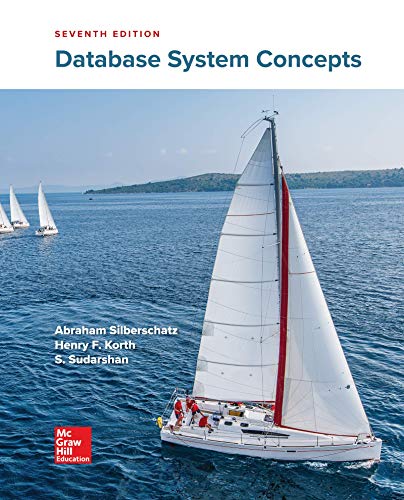
python: Write a program with a class definition 'Aircraft'. The constructor should take in arguments for tail_number, latitude, longitude, altitude, heading, and speed. These should be stored in a dictionary. The key is the parameter name (e.g. 'tail_number'), and the value will be the argument passed to the constructor (e.g. 'N51123ND'). Also have a method definition in the class called 'print_plane', which prints out all of these values for a given instantiation of the class. In the main portion of the program, have an initially-empty list called 'airplanes'. Have the user enter the arguments listed above, call the constructor, and add the new object to the list. Do this three times, so that there will be a list with three instantiations of the Aircraft class in it. Finally, use a 'for' loop to iterate over the list, calling the print_plane method from each object in the list.

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 4 images

- Which of the statements are true about the class Magazine? 01: class Magazine { 02: public: 03: Magazine (int maxSize = 100); 04: void add (Article a); 05: int numArticles() const; 06: Article getArticle (int i); 07: private: 08: Article* array; 09: int numberOfArticles; 10: }; The compiler will provide a destructor for this class. It has a default constructor It is const-correct. It has a copy constructor The programmer should provide an assignment operatorarrow_forwardJava:arrow_forwardWrite in Javaarrow_forward
- Lab 2 – Designing a class This lab requires you to think about the steps that take place in a program by writing pseudocode. Read the following program prior to completing the lab. Design a class named Computer that holds the make, model, and amount of memory of a computer. Include methods to set the values for each data field, and include a method that displays all the values for each field. For the programming problem, create the pseudocode that defines the class and enter it below. Enter pseudocode herearrow_forwardSummary In this lab, you create a derived class from a base class, and then use the derived class in a Python program. The program should create two Motorcycle objects, and then set the Motorcycle’s speed, accelerate the Motorcycle object, and check its sidecar status. Instructions Open the file named Motorcycle.py. Create the Motorcycle class by deriving it from the Vehicle class. Call the parent class __init()__ method inside the Motorcycle class's __init()__ method. In theMotorcycle class, create an attribute named sidecar. Write a public set method to set the value for sidecar. Write a public get method to retrieve the value of sidecar. Write a public accelerate method. This method overrides the accelerate method inherited from the Vehicle class. Change the message in the accelerate method so the following is displayed when the Motorcycle tries to accelerate beyond its maximum speed: "This motorcycle cannot go that fast". Open the file named MyMotorcycleClassProgram.py. In the…arrow_forward1. Square Roots Create a class with a method that, given an integer, returns an array of double-precision floating point numbers (known in Java as double), each of which is a square root of a number between 2 and the parameter to the method. For example, if the parameter is 5, the method should return an array of 4 doubles, with approximate values 1.4142135623, 1.7320508075, 2.0, and 2.2360679774. Your method should check the validity of the parameter, and take appropriate action if the parameter is invalid. 2. Reading Files Create a class with a method that, given a string representing a file name, returns an integer with the number of characters in the file. For example, if the file has 10 characters, your method must return the number 10. 3. Main Class Create a class HW1.java with a main method that does both of the following: calls the method from part 1 with a random integer between 0 and 10, and prints each of the numbers in the result. The numbers should all be printed on the…arrow_forward
- Write a program that will contain an array of person class objects. The program should include two classes: 1) One class will be the person class. 2) The second class will be the team class, which contain the main method and the array or array list. The person class should include the following data fields; Name Phone number Birth Date Jersey Number Be sure to include get and set methods for each data field.The team class should contain the data fields for the team, such as: Team name Coach name Conference name The program should include the following functionality. Add person objects to the array; Find a specific person object in the array; (find a person using any data field you choose such as name or jersey number) Output the contents of the array, including all data fields of each person object. (display roster)arrow_forwardPart 3. arange Method Define a method in simpy named arange. Its purpose is to fill in the values attribute with range of values, like the range built-in function, but in terms of floats. It has three parameters in addition to self, the last being optional: 1. start - a float indicating the first value in the range 2. stop - a float that is not included in the produced range values 3. step - a float whose default value is 1.0 that indicates how to increase (or decrease) each subsequent item in the generated range. Unlike the built- in range function, this can be a fractional, float value. Step cannot be 0.0. Think carefully about what the value of step tells you about how to design your loop(s) for this method. Before any looping, you should assert step != 0.0 to be sure you avoid an infinite loop with an invalid argument. positive = Simpy([]) positive.arange(1.0, 5.0) print("Actual: ", positive, " - Expected: Simpy([1.0, 2.0, 3.0, 4.0])") fractional = Simpy([]) positive.arange (0.0,…arrow_forwardMake Album in c++ You are going to develop a new class named Album. This class will has (at a minimum) teh following attributes and features: Attributes: Album Title Artist Name Array of song names (Track Listing) Array of track lengths (decide on a unit) At most there can be 20 songs on an album. Behaviors: Default and at least one argumented constructor Appropriate getters for attributes bool addTrack(string name, type duration) - adds track to album. You decide type on duration. Make sure you document what dis is. getAlbumLength() - method dat returns total length of album matching watever units / type you decide. getAlbumLength_string() - method dat returns total length of album in mm:ss format string. I would suggest using you're other getLength function here. string shuffleAlbum(int n) - method dat returns a formatted string made up of n random tracks from teh album. The string will be in the format [1] track_name (mm:ss), [2] track_name (mm:ss)... Well formatted print()…arrow_forward
- Write a code for a banking program.a) In this question, first, you need to create a Customer class, this class should have:• 2 private attributes: name (String) and balance (double)• Parametrized constructor to initialize the attributes• Methods:i. public String toString() that gives back the name and balanceii. public void addPercentage; this method will take a percentage value andadd it to the balanceb) Second, you will create a driver class and ask the user to enter 6 customers’ informationand then you will create an array of Customer objects.c) Then you use this array used for various operations as shown in the output.• Using the array of customer objects, you need to search for all customers whohave less than $150• Using the array of customer objects, you need to get the average balance of thebalances in this array• Using the array of customer objects, you need to get the customer with thehighest balance and lowest balance• Using the array of customer objects, you need to show all…arrow_forwardQuadratic equations The form of a quadratic equation is ax2 + bx + c = 0. Write a program that solves a quadratic equation in all cases, including when both roots are complex numbers. Remember that the solutions are x = (-b + sqrt(b2 -4ac))/2a and (-b - sqrt(b2 -4ac))/2a For this you will need to set up the following classes: Complex: Encapsulates a complex number. ComplexPair: Encapsulates a pair of complex numbers. Quadratic: Encapsulates a quadratic equation. – Assume the coefficients are all of type int for this example. SolveEquation: Contains the main method. (I give this to you it is shown below) Along with the usual constructors, accessors and mutators you will need the following additional methods: In the Complex class a method called isReal to determine whether a complex object is real or not returning a boolean value. In the ComplexPair class a method bothIdentical that determines if both complex numbers are identical, returning a boolean value. In the…arrow_forwardWritten in Python It should have an init method that takes two values and uses them to initialize the data members. It should have a get_age method. Docstrings for modules, functions, classes, and methodsarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
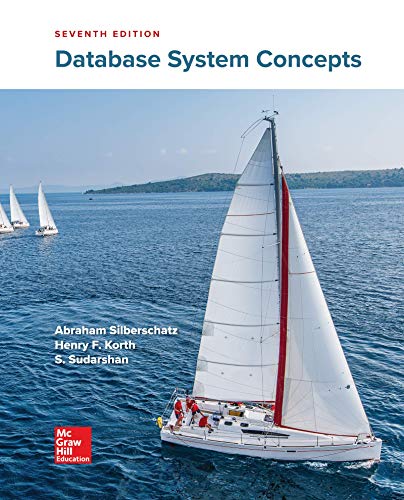
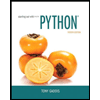
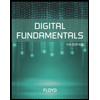
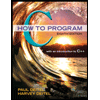
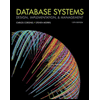
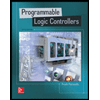