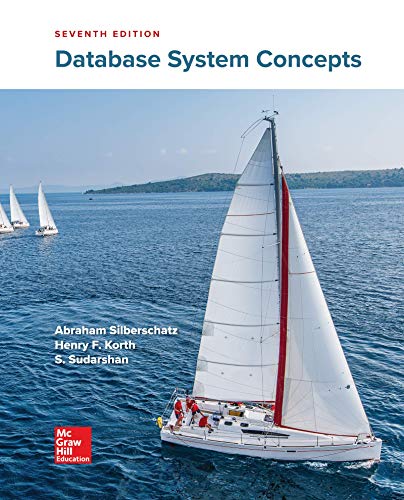
Concept explainers
It is a minimum binary heap. The main goal is to implement a minimum binary using a python list. Please help with the bold codes. All the not bold parts shouldn't be modified.
Only the bold parts should be modified.
I've said that no other methods should be added and no (pos) shouldn't be used. Please help me with not adding the other methods(def) to the code, it needs to work only by modifying the code provided above... I need to have an expected output just by having added the code below the sentence #YOUR CODE STARTS HERE. No other added methods(def).
Also, I haven't learned the (pos) function in python, so can you help me without using that function? Not allowed to use index() method as well.
getMin(self) needs to return the minimum value in the heap
_parent(self, index) it needs to return value of the parent of the element at the specific index
_leftChild(self, index) and _right Child(self,index) return value of the left/right child of the element at the specific index
insert(self, item) needs to insert an item into the heap while maintaining the minimum heap property. It must use the _parent method.
deleteMin(self) it needs to remove the minimum element of the heap and returns the value of the element that was just removed. The special cases where the heap is empty or contains only one element has been implemented for you already, your task is to implement the general case. According to the minimum heap property, the minimum element is the root node. When percolating down, if both children have the same value, swap with the left child. It must use the _leftChild and _rightChild methods.
heapSort(numList) It takes a list of numbers and uses the heap sort
Image is for input and output description.
Starter Code
class MinBinaryHeap:
def __init__(self):
self._heap=[]
def __str__(self):
return f'{self._heap}'
__repr__=__str__
def __len__(self):
return len(self._heap)
@property
def getMin(self):
# YOUR CODE STARTS HERE
pass
def _parent(self,index):
# YOUR CODE STARTS HERE
pass
def _leftChild(self,index):
# YOUR CODE STARTS HERE
pass
def _rightChild(self,index):
# YOUR CODE STARTS HERE
pass
def insert(self,item):
# YOUR CODE STARTS HERE
pass
def deleteMin(self):
# Remove from an empty heap or a heap of size 1
if len(self)==0:
return None
elif len(self)==1:
deleted=self._heap[0]
self._heap=[]
return deleted
else:
# YOUR CODE STARTS HERE
pass
def heapSort(numList):
'''
>>> heapSort([9,1,7,4,1,2,4,8,7,0,-1,0])
[-1, 0, 0, 1, 1, 2, 4, 4, 7, 7, 8, 9]
>>> heapSort([-15, 1, 0, -15, -15, 8 , 4, 3.1, 2, 5])
[-15, -15, -15, 0, 1, 2, 3.1, 4, 5, 8]
'''
# YOUR CODE STARTS HERE
The image is for a note.
Expected output
>>> h = MinBinaryHeap()
>>> h.insert(10)
>>> h.insert(5)
>>> h
[5, 10]
>>> h.insert(14)
>>> h._heap
[5, 10, 14]
>>> h.insert(9)
>>> h
[5, 9, 14, 10]
>>> h.insert(2)
>>> h
[2, 5, 14, 10, 9]
>>> h.insert(11)
>>> h
[2, 5, 11, 10, 9, 14]
>>> h.insert(14)
>>> h
[2, 5, 11, 10, 9, 14, 14]
>>> h.insert(20)
>>> h
[2, 5, 11, 10, 9, 14, 14, 20]
>>> h.insert(20)
>>> h
[2, 5, 11, 10, 9, 14, 14, 20, 20]
>>> h.getMin
2
>>> h._leftChild(1)
5
>>> h._rightChild(1)
11
>>> h._parent(1)
>>> h._parent(6)
11
>>> h._leftChild(6)
>>> h._rightChild(9)
>>> h.deleteMin()
2
>>> h._heap
[5, 9, 11, 10, 20, 14, 14, 20]
>>> h.deleteMin()
5
>>> h
[9, 10, 11, 20, 20, 14, 14]
>>> len(h)
7
>>> h.getMin
9
'''
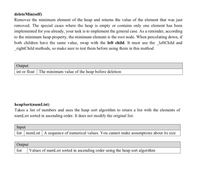
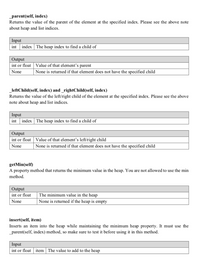

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- In python. Write a LinkedList class that has recursive implementations of the add and remove methods. It should also have recursive implementations of the contains, insert, and reverse methods. The reverse method should not change the data value each node holds - it must rearrange the order of the nodes in the linked list (by changing the next value each node holds). It should have a recursive method named to_plain_list that takes no parameters (unless they have default arguments) and returns a regular Python list that has the same values (from the data attribute of the Node objects), in the same order, as the current state of the linked list. The head data member of the LinkedList class must be private and have a get method defined (named get_head). It should return the first Node in the list (not the value inside it). As in the iterative LinkedList in the exploration, the data members of the Node class don't have to be private. The reason for that is because Node is a trivial class…arrow_forwardIn this project you will implement a Set class which represents a general collection of values. For this assignment, a set is generally defined as a list of values that is sorted and does not contain any duplicate values. More specifically, a set shall contain no pair of elements e1 and e2 such that e1.equals(e2) and no null elements. Requirements To ensure consistency among all implementations there are some requirements that all implementations must maintain. • Your implementation should reflect the definition of a set at all times. • For simplicity, your set will be used to store Integer objects. • An ArrayList object must be used to represent the set. • All methods that have an object parameter must be able to handle an input of null. • Methods such as Collections.sort that automatically sort a list may not be used. • The Set class shall reside in the default package. Recommendations There are deviations in program implementation that are acceptable and will not impact the overall…arrow_forwardWithout using the java collections interface (i.e. do not import java.util.List, LinkedList, etc. ) Write a java program that inserts a new String element (String newItem) into a linked list before another specified item (String itemToInsertBefore). For example if items "A", "B", "C" and "D" are in a linked list in that order and the below method is called, insertBefore("E", "C"), then "E" would be inserted before "C", making the final list to be "A", "B", "E", "C" and "D" with no nulls or blank elements or any elements missing or anything. It should work for all lenghths of linkedlists of Strings. public Boolean insertBefore(String newItem, String itemToInsertBefore) { // returns true if done successfully, else returns false if itemToInsertBefore cannot be found or some other error }arrow_forward
- you will create a simple heap data structure with several methods includingmaxHeapify and buildMaxHeap. To get started, import the starter file (Heap.java) into the heappackage you create in a new Java Project. Please do not change any of the method signatures inthe Heap class. Implement the methods described below. You are free to test your code however youprefer. Remember that arrays are indexed starting at 0 in Java. This will change the math in thepseudocode.public int parent(int i)This method should return the index of the parent of the ith element of the data array. If the ithelement is the root, then the method should return -1.public int left(int i)This method should return the index of the left child of the ith element of the data array. If the ithelement is a leaf, then the index will potentially be greater than the size of the data array. That’sfine.public int right(int i)This method should return the index of the right child of the ith element of the data array. If the…arrow_forwardCan you write a program in python for the dining philosopher problems that do not have deadlock? You have to use semaphores and use the strategy that a philosopher only picks the fork if both (left and right) are available. def philosopher(id: int, fork: list): The main is if __name__ == "__main__": semaphoreList = list() #this list will hold one semaphore per fork Philosophers = 5 for i in range(Philosophers): semaphoreList.append(multiprocessing.Semaphore(1)) philosopherList = list() for i in range(Philosophers): #instantiate all processes representing philosophers philosopherList.append(multiprocessing.Process(target=philosopher, args=(i, semaphoreList))) for j in range(Philosophers): #start all child processes philosopherList[j].start() for k in range(Philosophers): #join all child processes philosopherList[k].join() sample output: DEBUG: philosopher2 has chopstick2 DEBUG: philosopher2 has chopstick3 DEBUG: philosopher2 eating DEBUG: philosopher1 has chopstick1 DEBUG: philosopher0…arrow_forwardUsing Java to design and implement the class PascalTriangle that will generate a Pascal Triangle from a given number of rows. Represent each row in a triangle as a list and the entire triangle as a list of these lists. Please implement the class ArrayList for these lists. Please do not use the binomial coeffiient formula { C(n,k)= n! / (k!*(n-k)!) to create the triangle. The triangle has to be generate using in this way: each row of the triangle begins and ends with 1, value at (x,y) equals to sum of value at (x-1, y-1) & (x-1,y), whereas x is the row number and y is the columm.arrow_forward
- Your crazy boss has assigned you to write a linear array-based implementation of the IQueue interface for use in all the company's software. You've tried explaining why that's a bad idea with logic and math, but to no avail. So - suppress your inner turmoil and complete the add() and remove() methods of the AQueue class and identify the runtime order of each of your methods. /** A minimal queue interface */ public interface IQueue { /** Add element to the end of the queue */ public void add(E element); /** Remove and return element from the front of the queue * @returns fırst element * @throws NoSuchElementException if queue is empty */ public E remove(); /** Not an ideal Queue */ public class AQueue implements IQueue{ private El) array: private int rear; public AQueue(){ array = (E[)(new Object[10]): rear = 0: private void expandCapacity) { if (rear == array.length) { array = Arrays.copyOf(array, array.length 2): @Override public void add(E element) { / TODO @Override public E…arrow_forwardHello!I'm currently in the process of developing an algorithm for a reverse method for a singly-linked list--not an array, which is a much easier algorithm and I have done before. I'm writing in Java. I'm working from a pre-written SinglyLinkedList class with certain methods (intentionally) empty, for us to fill.I have, unbelievably, spent somewhere around 5 hours going through drafts. The easiest implementation I've conceived of would be to create a second list with which to import the nodes and elements starting from the tail. public void reverse() {<Integer> list2 = new SinglyLinkedList();for (size) { list2.addLast(tail); list1.remove(tail); }}This is the rough draft pseudocode for the aforementioned implementation.If we were to work within the same list, instead of creating a new one we would have to go through, for a size of 10, a hundred iterations. Obviously, there are a number of problems.First, reverse() has been defined as a void method. It's not supposed to return…arrow_forwardThink about how you search a dictionary: you start in themiddle either go left or go right until you find the word you’re looking foror can’t narrow the word list down any further. It’s possible to do thesame in a list of numbers. Write an algorithm that, given a list of numbersand a secret number to find, tells you where in the list (index) you canfind that number or tells you the number isn’t in the list.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
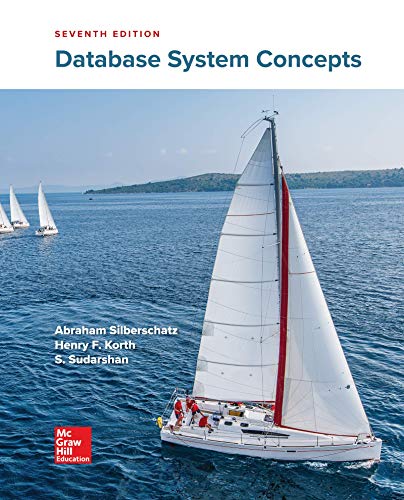
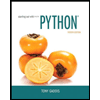
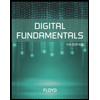
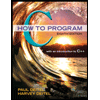
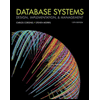
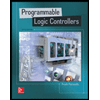