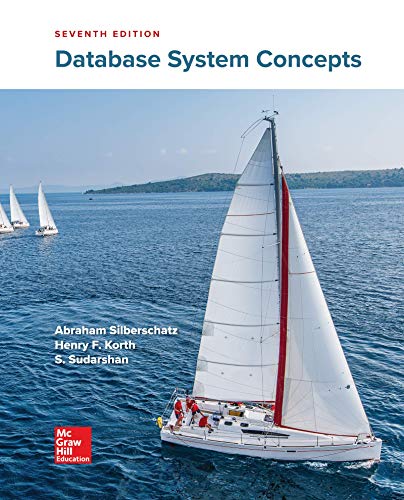
import java.util.Scanner;
public class PalindromeChecker {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.print("Please enter a string to test for palindrome or type QUIT to exit:\n");
String input = scanner.nextLine().toLowerCase();
if (input.equals("quit")) {
break;
}
if (isPalindrome(input)) {
System.out.println("The input is a palindrome.");
} else {
System.out.println("The input is not a palindrome.");
}
}
}
private static boolean isPalindrome(String str) {
str = str.replaceAll("[^a-zA-Z0-9]", "");
int left = 0;
int right = str.length() - 1;
while (left < right) {
if (str.charAt(left) != str.charAt(right)) {
return false;
}
left++;
right--;
}
return true;
}
}
Test Case 1
Desserts, I stressedENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
KayakENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quitENTER
Test Case 2
dadENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quitENTER
Test Case 3
momENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
QuitENTER
Test Case 4
helloENTER
The input is not a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quiTENTER
b,a,dENTER
The input is not a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quitENTER
Test Case 6
Able was I, ere I saw ElbaENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quitENTER
Test Case 7
A man, a plan, a canal, PanamaENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quitENTER
Test Case 8
@abENTER
The input is not a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
ab@ENTER
The input is not a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
@aaENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
aa@ENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quitENTER
Test Case 9
abbaENTER
The input is a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
abcaENTER
The input is not a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
aabcaaENTER
The input is not a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
abaceedabaENTER
The input is not a palindrome.\n
Please enter a string to test for palindrome or type QUIT to exit:\n
quitENTER

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 11 images

- Create a Flowchart //Java Source Code :- import java.util.Scanner; public class bExpert { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.println("\nVALUES"); System.out.print("Value 1: "); int v1 = sc.nextInt(); System.out.print("Value 2: "); int v2 = sc.nextInt(); System.out.print("Value 3: "); int v3 = sc.nextInt(); System.out.println("\nPROCESS"); System.out.println("\n1. Summation"); System.out.println("2. Product"); System.out.println("3. Min / Max"); System.out.println("4. Odd / Even"); char st = 'y'; while(st != 'n'){ System.out.print("\nCHOICE: "); int ch = sc.nextInt(); switch(ch) { case 1: int sum = v1 + v2 + v3; System.out.println("\nSum = "+sum); break; case 2: int prod =…arrow_forwardIf integer numberOfYears is 50, output "Equal to 1 half-century". Otherwise, output "Not equal to 1 half-century". End with a newline. ▶ Click here for examples 1 import java.util.Scanner; HEM & in to00 L 2 3 public class IfElse { 4 5 6 7 8 9 10 11 12 public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int numberOfYears; numberOf Years = scnr.nextInt(); V* Your code goes here */arrow_forwardHi, I am struggling a bit with this problem. (This is in Java by the way.) Provided code: import java.util.Scanner; public class LabProgram { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String userText; // Add more variables as needed userText = scnr.nextLine(); // Gets entire line, including spaces. /* Type your code here. */ }}arrow_forward
- JAVA CODE: check outputarrow_forwardimport java.util.Scanner; public class DebugSix4 { public static void main(String[] args) { int high, low, count = 0; final int NUM = 5; Scanner input = new Scanner(System.in); System.out.print("This application displays " + NUM + " random numbers" + "\nbetween the low and high values you enter" + "\nEnter low value now... "); low = input.nextInt(); // Inserted the missing semicolon System.out.print("Enter high value... "); high = input.nextInt(); // Added the missing dot to the method call. while (low >= high) { // For proper input validation, changed "" to ">=" System.out.println("The number you entered for a high number, " + high + ", is not more than " + low); System.out.print("Enter a number higher than " + low + "... "); high =…arrow_forwardBesides main() this program has one method that computes the amount of raise and the new salary for an employee. The current salary and a performance rating (a String: "Excellent", "Good" or "Poor") are input. import java.util.Scanner; public class Salary { public static void main (String[] args) { Declare variables Prompt for and read current salary and rating For an excellent rating compute raise as 0.06 of current salary For a good rating compute raise as 0.04 of current salary For a poor rating compute raise as 0.015 of current salary You can use abc.equals(“xyz”) to verify the rating read For an invalid rating display an appropriate message and display the salary without change; otherwise, compute and print new salary and raise amount main() must display the message } } Note:- Please type and execute this java program and also need an output for this java program as soon as possible.arrow_forward
- Java Questions - (Has 2 Parts). Based on each code, which answer out of the choices "A, B, C, D, E" is correct. Each question has one correct answer. Thank you. Part 1 - Given the following code, the output is __. class Sample{ public static void main (String[] args){ try{ System.out.println(5/0);}catch(ArithmeticException e){ System.out.print("Divide by zero????");}finally{ System.out.println("5/0"); } }} A. Divide by zero????B. 5/0C. Divide by zero????5/0D. 5/0Divide by zero????E. "5/0" Part 2 - Given the following code, the output is __. try{ Integer number = new Integer("1"); System.out.println("An Integer instance.");}catch (Exception e){ System.out.println("An Exception.");} A. An Integer instance.B. An Exception.C. 1D. Error: ExceptionE. None of the optionsarrow_forwardI have this code: import java.util.Scanner; public class CalcTax { public static void main(String[] args) { Scanner sc = new Scanner(System.in); // Welcome message System.out.println("Welcome to my tax calculation program."); // Declare the variables int i, pinCode, maxRetries = 3; String choice; // Get the PIN code for (i = 0; i < maxRetries; i++) { System.out.print("Please enter the PIN code: "); pinCode = sc.nextInt(); if (pinCode == 5678) { break; } System.out.println("Invalid pin code. Please try again."); } if (i == maxRetries) { System.out.println("Invalid pin code. You have reached the maximum number of retries."); System.exit(0); } // Get the income, interest, deduction, and paid tax amount double income, interest, deduction, paidTax; do { System.out.print("Please…arrow_forwardimport java.util.Scanner; class Program41 {//PSEUDOCODE //Write a program that prompts the user to enter a full name consisting of three names //first //middle //last // into one String variable //Then use methods of class String to: //print the number of characters in the full name, excluding spaces //print just the middle name and the number of characters in it //print the three initials of the name //print the last name in all lower case //print the full name in the usual alphabetical format (Last, First Middle) and proper case public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter full name:"); String fullName = sc.nextLine(); System.out.println("\nNumber of Charatcers in your name " + fullName + "':" + fullName.replace("","").length()); String[] subNames = fullName.split(" "); System.out.println("\nMiddle Name: " +…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
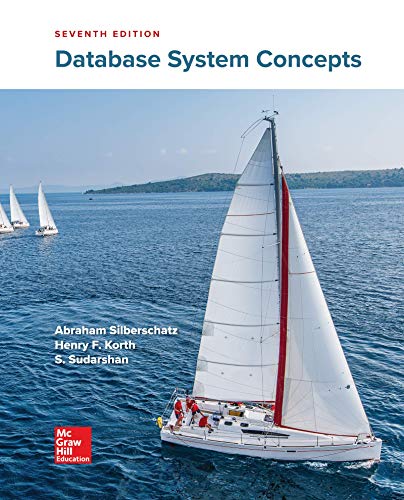
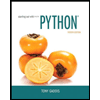
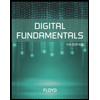
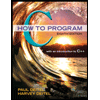
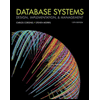
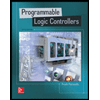