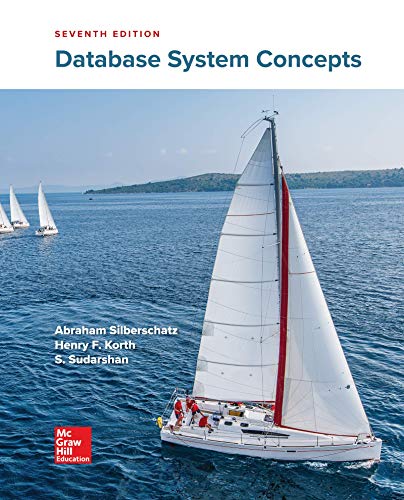
Java
See attached
The given program reads a list of single-word first names and ages (ending with -1), and outputs that list with the age incremented. The program fails and throws an exception if the second input on a line is a String rather than an Integer. At FIXME in the code, add a try/catch statement to catch java.util.InputMismatchException, and output 0 for the age.
import java.util.Scanner;
import java.util.InputMismatchException;
public class NameAgeChecker {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
String inputName;
int age;
inputName = scnr.next();
while (!inputName.equals("-1")) {
// FIXME: The following line will throw an InputMismatchException.
// Insert a try/catch statement to catch the exception.
age = scnr.nextInt();
System.out.println(inputName + " " + (age + 1));
inputName = scnr.next();
}
}
}
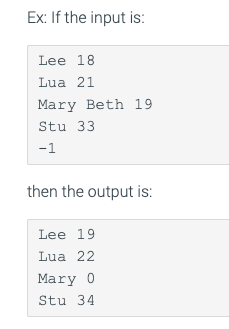

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- In Java Only: My program is not working correctly, The history teacher at your school needs help grading a true/false test.The student's IDs and test answers are stored ina file, The first entry in the file contains the answers to the test in the following form: TFFTFFTTTTFFTFTFTFTT Every other entry in the file is the student;s ID, followed by a blank, followed by the student;s response. For example, the entry: ABC54301 TFTFTFTT TFTFTFFTTFT indicstes that the student;s ID is ABC54301 and the answer to question 1 is True, the answer to question 2 is false, and so on. This student did not answer question 9. he exam has 20 questions, and the class has more than 150 students. Each correct answer is awarded 2 points, each wrong answer gets -1 point, and no answer gets 0 points. Write a program that processes the test data. The output should be the student's ID, followed by the student's answers, followed by the test score, and then followed by the test grade. Here is my code, I keep…arrow_forwardWhen reading data from a text file, it is common to see numbers such as 12,345, $20, or 195*. Write a method that cleans a string containing digits by removing any characters that are not a digit or a - sign. Then convert to an integer and return the result. Numbers.java 1 public class Numbers 2 { /** Cleans a string containing an integer and converts it. @param number a string containing an integer and possibly extra characters @return the value of the number inside the string * / blic static int clean(String number) { 4 5 7 8 9 10 11 } 12 }arrow_forwardModify catchsignal.c to only allow user to break the program when they use Ctrl-C twice. Modify this file to allow user to break the program when they use Ctrl-C twice.*/ #include <stdio.h>#include <unistd.h>#include <signal.h> void sig_handler(){ printf("I got an INT signal\n");} int main(void){ signal(SIGINT, sig_handler); int i; while(1){ printf("%d\n",i); i++; sleep(1); } return 0;}arrow_forward
- Write a program called Guests.java that prompts a user to enter how many guests he will host. Next, reads a series of the names into an array of Strings using Scanner, and then prints back "Welcome" to all of those guests.arrow_forwardEx: Use getline() to get a line of user input into a string. Output the line. Enter text: IDK if I'll go. It's my BFF's birthday. You entered: IDK if I'll go. It's my BFF's birthday. Search the string (using find()) for common abbreviations and print a list of each found abbreviation along with its decoded meaning. Ex: Enter text: IDK if I'll go. It's my BFF's birthday. You entered: IDK if I'll go. It's my BFF's birthday. BFF: best friend forever IDK: I don't know Support these abbreviations: BFF -- best friend forever IDK -- I don't know . JK -- just kidding TMI -- too much information TTYL-talk to you later 1 #include 2 // FIXME include the string Library 3 using namespace std; 4 5 int main() { 6 7 8 9 10 } /* Type your code here. */ return 0; main.cpparrow_forwardJAVA PPROGRAM Please Modify this program with further modifications as listed below: ALSO, take out and change the following in the program: System.out.println("Program terminated."); because the test case does not need it And change this in the program: Please enter the file name or type QUIT to exit:\n so it reperats once for every test case because it repeats twice in every case as shown in the screenshot. I only need it once. I have provided the failed the test cases and the inputs as a screenshot. The program must pass the test case when uploaded to Hypergrade. import java.io.*;import java.util.ArrayList;import java.util.Scanner;public class SymmetricalNameMatcher { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); String fileName; do { // Prompt the user to enter a file name or 'QUIT' to exit. System.out.print("Please enter the file name or type QUIT to exit:\n"); fileName =…arrow_forward
- Write a complete Python program that opens a file called sample.txt for reading, and opens a file called out.txt for writing.arrow_forwardCan someone please help me with this project for my Java Programming class? If you could correct my mistakes for me that would be great.arrow_forwardWrite a program using while loop and for loops to print first 50 prime numbers in 5 lines, each line containing 10 numbers. 1. Create a program called CountCharacters.java. 2. At the top of your java file add the following documentation comments. Replace the italicized text with the appropriate content. /** @author Your Name CS 110 Section 012 Lab 9 Final Today's Date 3. Import the Scanner class and ask user to enter a line. Implement the while loop so that the user can input any number of lines and one line at a time. To stop entering lines, the user can write "quit. (Hint: Use the string method <str>.equals() in the while condition to stop the while loop OR a break keyword). 4. Implement the for loop inside the while loop to iterate through the line one character at a time to count and output the number of blank spaces, alphabetic characters, digit characters, and other characters in all the lines. You need to use different methods of character class like charAt(), isDigit(),…arrow_forward
- For Java Write code for an if-else block that checks separates integers into the following categories: An integer less than 0, An integer greater than 0 and less than or equal to 10, An integer greater than 10 that is even, and an integer greater than 10 that is odd. Have each category print out a different statement. What are some ways you can make your code more efficient when using if-else blocks?arrow_forwardComputer Programming Java Write a method named FirstHalf that receives a String parameter named word and then returns the first half of the string. You are guaranteed as a precondition that word has an even length greater than 1.arrow_forwardThis is a python file Fix this code and the output is the attachment #filename: modValidateEmail.py def isValidMSUMEmail(email): if email.count('@') != 1: return False tmp= email.split('@') if len(tmp) == 0: return False userName = tmp[0] if not userName[0].isalpha(): return False for c in userName: if not c.isalpha() and c != '.': return False inst= tmp[1] if inst != 'mnstate.edu': return False else: return True #filename: UnitTestValidEmail.py from unittest import * from modValidateEmail import * class TestValidEmail(TestCase): #create a subclass of unittest.TestCase def testUserName(self): self.assertEqual(isValidMSUMEmail('.joe@mnstate.edu'),False) self.assertEqual(isValidMSUMEmail('joe4@mnstate.edu'),False) self.assertEqual(isValidMSUMEmail('joe$smith@mnstate.edu'),False) self.assertEqual(isValidMSUMEmail('joe.smith@mnstate.edu'),True) def…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
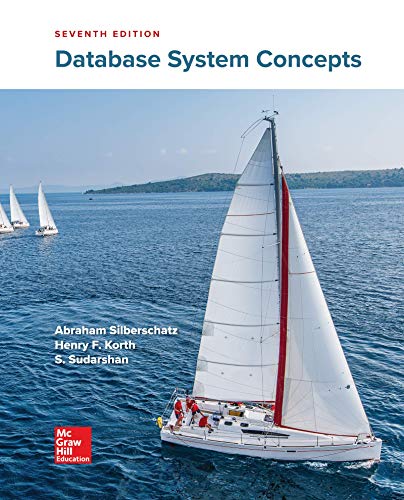
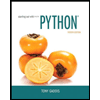
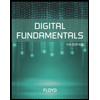
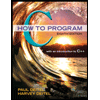
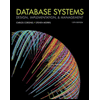
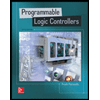