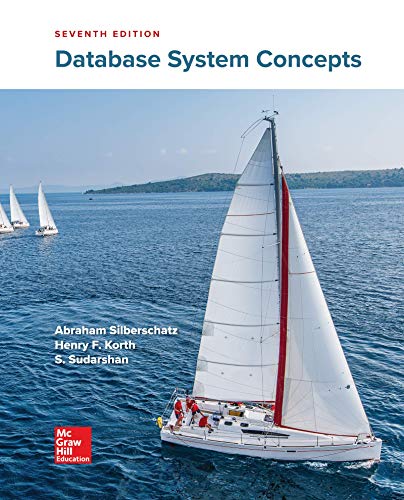
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
![**Step 1**: The following code copies a string from source to target:
```assembly
.data
source BYTE "This is the source string",0
target BYTE SIZEOF source DUP(0)
.code
mov esi,0 ; index register
mov ecx,SIZEOF source ; loop counter
L1:
mov al,source[esi] ; get char from source
mov target[esi],al ; store it in the target
inc esi ; move to next character
loop L1 ; repeat for entire string
mov edx, OFFSET source
call WriteString
mov edx, OFFSET target
call WriteString
```
**Explanation**:
- The code initializes a `source` string and a `target` location to store the copied string.
- The `esi` register is used as an index to iterate through each character of the `source`.
- For each iteration, the current character from `source` is stored in the `target`.
- A loop (`L1`) ensures this operation continues until the entire string is copied.
- After copying, a subroutine `WriteString` is called to output both `source` and `target`.
**Task**: Rewrite the program using indirect addressing rather than indexed addressing.](https://content.bartleby.com/qna-images/question/a6698ae8-6c01-4434-828e-21af6fc513df/82b27a8e-0e78-439f-b17b-c50e1964fcdf/5ob5tit_thumbnail.jpeg)
Transcribed Image Text:**Step 1**: The following code copies a string from source to target:
```assembly
.data
source BYTE "This is the source string",0
target BYTE SIZEOF source DUP(0)
.code
mov esi,0 ; index register
mov ecx,SIZEOF source ; loop counter
L1:
mov al,source[esi] ; get char from source
mov target[esi],al ; store it in the target
inc esi ; move to next character
loop L1 ; repeat for entire string
mov edx, OFFSET source
call WriteString
mov edx, OFFSET target
call WriteString
```
**Explanation**:
- The code initializes a `source` string and a `target` location to store the copied string.
- The `esi` register is used as an index to iterate through each character of the `source`.
- For each iteration, the current character from `source` is stored in the `target`.
- A loop (`L1`) ensures this operation continues until the entire string is copied.
- After copying, a subroutine `WriteString` is called to output both `source` and `target`.
**Task**: Rewrite the program using indirect addressing rather than indexed addressing.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Find a line with an error in the following code segment: 1: void displayFile(fstream file) {2: string line;3: while (file >> line) 4: cout << line << endl;5: } Group of answer choices 1 2 3 4arrow_forwardGiven: string s; int first, last; Write code to repeatedly validate that first is less than last and both first and last are valid indexes of the string s. Then print all characters of the string s from index first to last inclusive. Example Output 1 Enter a string and two indexes: Queensborough 25 eens Example Output 2 Enter a string and two indexes: Science 2 10 Enter a string and two indexes: Science -15 Enter a string and two indexes: Science 2 4 ienarrow_forwardDefine the following data structures: Record File Array Stringarrow_forward
- // Flowers.cpp - This program reads names of flowers and whether they are grown in shade or sun from an input // file and prints the information to the user's screen. // Input: flowers.dat. // Output: Names of flowers and the words sun or shade. #include <fstream> #include <iostream> #include <string> using namespace std; int main() { // Declare variables here // Open input file // Write while loop that reads records from file. fin >> flowerName; // Print flower name using the following format //cout << var << " grows in the " << var2 << endl; fin.close(); return 0; } // End of main functionarrow_forwardJava codearrow_forwardWhat happens if you enter a string greater than eight characters? How can a buffer overflow be avoided?arrow_forward
- Given string userString on one line, character newChar on a second line, and integer strIndex on a third line, change the character at index strIndex of userString to newChar. Ex: If the input is: garlic Z 0 then the output is: Zarlic Note: Assume the length of string userString is greater than strIndex. #include <iostream> #include <string> using namespace std; int main() { string userString; char newChar; int strIndex; getline(cin, userString); cin >> newChar; cin >> strIndex; /* Your code goes here */ cout << userString << endl; return 0; }arrow_forwardFile redirection allows you to redirect standard input (keyboard) and instead read from a file specified at the command prompt. It requires the use of the < operator (e.g. java MyProgram < input_file.txt) True or Falsearrow_forwardThanks for the code, can you help me fix this two errors, i dont know how to do it.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
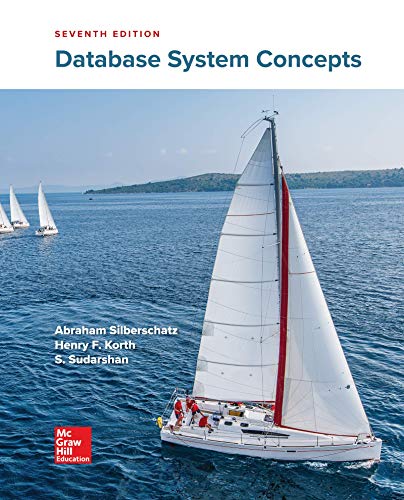
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
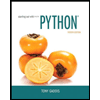
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
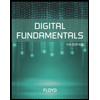
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
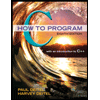
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
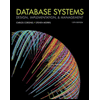
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
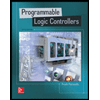
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education