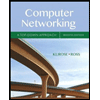
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
let list=1200 element.
Note: java programmimg, use given code.
3a. Use the binary search 3b. Use the sequential search
- Print the number of comparison in step 3(a) and 3(b). If the item is found in the list, print its position.
import java.util.*;
public class Problem5
{
staticScannerconsole = newScanner(System.in);
finalstaticintSIZE = 1000;
publicstaticvoidmain(String[] args)
{
Integer[] intList = newInteger[SIZE];
SearchSortAlgorithms<Integer> intSearchObject
= new SearchSortAlgorithms<Integer>();
//code
}
public interface SearchSortADT<T>
{
publicintseqSearch(T[] list, intstart, intlength, TsearchItem);
publicintbinarySearch(T[] list, intstart, intlength, TsearchItem);
publicvoidbubbleSort(Tlist[], intlength);
publicvoidselectionSort(T[] list, intlength);
publicvoidinsertionSort(T[] list, intlength);
publicvoidquickSort(T[] list, intlength);
publicvoidheapSort(T[] list, intlength);
}
public class SearchSortAlgorithms<T> implements SearchSortADT<T>{
privateintcomparisons;
publicintnoOfComparisons(){
// finishthis
}
publicvoidinitializeNoOfComparisons()
{
//code
}
publicintseqSearch(T[] list, intstart, intlength, TsearchItem)
{
intloc;
booleanfound = false;
for (loc = start; loc < length; loc++)
{
if (list[loc].equals(searchItem))
{
found = true;
break;
}
}
if (found)
return loc;
else
return -1;
}
publicintbinarySearch(T[] list, intstart, intlength, TsearchItem)
{
intfirst = start;
intlast = length - 1;
intmid = -1;
booleanfound = false;
while (first <= last && !found)
{
mid = (first + last) / 2;
Comparable<T> compElem = (Comparable<T>) list[mid];
if (compElem.compareTo(searchItem) == 0)
found = true;
else
{
if (compElem.compareTo(searchItem) > 0)
last = mid - 1;
else
first = mid + 1;
}
}
if (found)
return mid;
else
return -1;
}
publicintbinSeqSearch15(T[] list, intstart, intlength, TsearchItem)
{
intfirst = 0;
intlast = length - 1;
intmid = -1;
booleanfound = false;
while (last - first > 15 && !found)
{
mid = (first + last) / 2;
Comparable<T> compElem = (Comparable<T>) list[mid];
comparisons++;
if (compElem.compareTo(searchItem) ==0)
found = true;
else
{
if (compElem.compareTo(searchItem) > 0)
last = mid - 1;
else
first = mid + 1;
}
}
if (found)
return mid;
else
returnseqSearch(list, first, last, searchItem);
}
publicvoidbubbleSort(Tlist[], intlength)
{
for (intiteration = 1; iteration < length; iteration++)
{
for (intindex = 0; index < length - iteration;
index++)
{
Comparable<T> compElem =
(Comparable<T>) list[index];
if (compElem.compareTo(list[index + 1]) > 0)
{
Ttemp = list[index];
list[index] = list[index + 1];
list[index + 1] = temp;
}
}
}
}
publicvoidselectionSort(T[] list, intlength)
{
for (intindex = 0; index < length - 1; index++)
{
intminIndex = minLocation(list, index, length - 1);
swap(list, index, minIndex);
}
}
privateintminLocation(T[] list, intfirst, intlast)
{
intminIndex = first;
for (intloc = first + 1; loc <= last; loc++)
{
Comparable<T> compElem = (Comparable<T>) list[loc];
if (compElem.compareTo(list[minIndex]) < 0)
minIndex = loc;
}
return minIndex;
}
privatevoidswap(T[] list, intfirst, intsecond)
{
Ttemp;
temp = list[first];
list[first] = list[second];
list[second] = temp;
}
publicvoidinsertionSort(T[] list, intlength)
{
for (intfirstOutOfOrder = 1; firstOutOfOrder < length;
firstOutOfOrder ++)
{
Comparable<T> compElem =
(Comparable<T>) list[firstOutOfOrder];
if (compElem.compareTo(list[firstOutOfOrder - 1]) < 0)
{
Comparable<T> temp =
(Comparable<T>) list[firstOutOfOrder];
intlocation = firstOutOfOrder;
do
{
list[location] = list[location - 1];
location--;
}
while (location > 0 &&
temp.compareTo(list[location - 1]) < 0);
list[location] = (T) temp;
}
}
}
publicvoidquickSort(T[] list, intlength)
{
recQuickSort(list, 0, length - 1);
}
privateintpartition(T[] list, intfirst, intlast)
{
Tpivot;
intsmallIndex;
swap(list, first, (first + last) / 2);
pivot = list[first];
smallIndex = first;
for (intindex = first + 1; index <= last; index++)
{
Comparable<T> compElem = (Comparable<T>) list[index];
if (compElem.compareTo(pivot) < 0)
{
smallIndex++;
swap(list, smallIndex, index);
}
}
swap(list, first, smallIndex);
return smallIndex;
}
privatevoidrecQuickSort(T[] list, intfirst, intlast)
{
if (first < last)
{
intpivotLocation = partition(list, first, last);
recQuickSort(list, first, pivotLocation - 1);
recQuickSort(list, pivotLocation + 1, last);
}
}
publicvoidheapSort(T[] list, intlength)
{
buildHeap(list, length);
for (intlastOutOfOrder = length - 1; lastOutOfOrder >= 0;
lastOutOfOrder--)
{
Ttemp = list[lastOutOfOrder];
list[lastOutOfOrder] = list[0];
list[0] = temp;
heapify(list, 0, lastOutOfOrder - 1);
}
}
privatevoidheapify(T[] list, intlow, inthigh)
{
intlargeIndex;
Comparable<T> temp =
(Comparable<T>) list[low];
largeIndex = 2 * low + 1;
while (largeIndex <= high)
{
if (largeIndex < high)
{
Comparable<T> compElem =
(Comparable<T>) list[largeIndex];
if (compElem.compareTo(list[largeIndex + 1]) < 0)
largeIndex = largeIndex + 1;
}
if (temp.compareTo(list[largeIndex]) > 0)
break;
else
{
list[low] = list[largeIndex];
low = largeIndex;
largeIndex = 2 * low + 1;
}
}
list[low] = (T) temp;
}
privatevoidbuildHeap(T[] list, intlength)
{
for (intindex = length / 2 - 1; index >= 0; index--)
heapify(list, index, length - 1);
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Similar questions
- White a Java Function to find unique number in a list ls = [ 2, 3, 4, 10, 5, 6, 6, 4, 10, 3 ] , unique number is number that doesn't repeat this case unique list will be = [ 2, 5 ]arrow_forwardPythonarrow_forwardComplete this codeYou can write a recursive helper method that takes any number of arguments and then call it inside the method, but you cannot use any loops. /** * Decide if it is possible to divide the integers in a list into two sets, * so that the sum of one set is odd, and the sum of the other set is a multiple of 10. * Every integer must be in one set or the other. * For example, oddAndTen([5, 5, 3]) → true, * and oddAndTen([5, 5, 4]) → false. * @param list is a list of integers. * @return true iff there is one odd partition and the other multiple of 10. */public static boolean oddAndTen(List<Integer> list) { // call your recursive helper method return ...} private static boolean oddAndTenHelper(...) { // add any parameters // base case // recursive step}arrow_forward
- The chapter is nested lists Create code in python using for loops Thanks!arrow_forwardSelect problem below. Your post must include: The problem statement. A description of your solution highlighting the use of the split(), join(), lists and the different list operations involved in solving the problems. Include line numbers from your program dont use JAVA Make Username. Create a program that reads a full name as a single string, and and uses the split creates a username with the following rules: The first character of the username is the first character of the first name. The second character of the username is the first letter of the middle name, if one was provided. The rest of the characters will be a prefix of the last name, long enough to meet the length requirement. The username can have up to 8 characters only, and in lowercase. Here is a sample execution:arrow_forwardBinary Search of Strings1. Write a version of the selection sort algorithm presented in the unit, which is usedto search a list of strings.2. Write a version of the binary search algorithm presented in the unit, which isused to search a list of strings. (Use the selection sort that you designed aboveto sort the list of strings.)3. Create a test program that primes the list with a set of strings, sorts the list, andthen prompts the user to enter a search string. Your program should then searchthe list using your binary search algorithm to determine if the string is in the list.Allow the user to continue to search for strings until they choose to exit theprogramarrow_forward
- For any element in keysList with a value greater than 50, print the corresponding value in itemsList, followed by a comma (no spaces). Ex: If the input is: 32 105 101 35 10 20 30 40 the output is: 20,30, 1 #include 2 3 int main(void) { const int SIZE_LIST = 4; int keysList[SIZE_LIST]; int itemsList[SIZE_LIST]; int i; 4 6 7 8 scanf("%d", &keysList[0]); scanf ("%d", &keysList[1]); scanf("%d", &keysList[2]); scanf("%d", &keysList[3]); 10 11 12 13 scanf ("%d", &itemsList[0]); scanf ("%d", &itemsList[1]); scanf("%d", &itemsList[2]); scanf ("%d", &itemsList[3]); 14 15 16 17 18 19 /* Your code goes here */ 20 21 printf("\n"); 22 23 return 0; 24 }arrow_forwardJava/Data Structures: The Java Class Library implementation of the interface list return null when an index is out of range. True or Falsearrow_forward6. the grade is under 20 which is outlier, remove it from the array list. 7. Print array list using System.out.println() 8. Use indexOf to print index of 80. 9. Use get function. 10. What is the difference between get and index of? 11. Print the values of the array list using Iterator class. 12.. Delete all the values of the array list using clear function. 13. Print all the values of the array after you execute clear using System.out.println(). what is the result of using clear function? 14. What is the shortcoming of using array List?arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
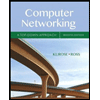
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
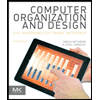
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
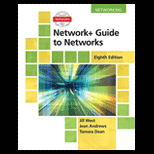
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
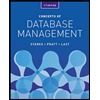
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
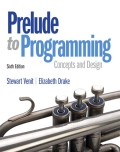
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
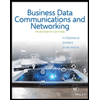
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY