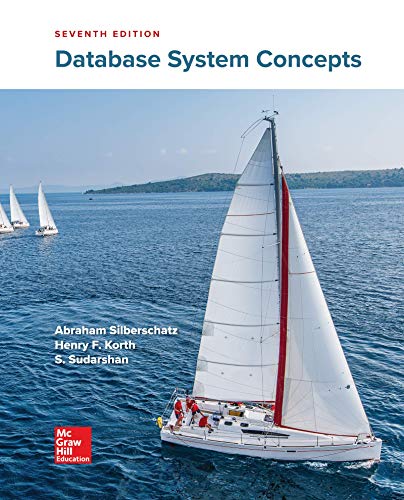
C++ compile a program to answer question in picture included.
Code to edit:
#include <iostream>
#include <string>
class DayOfYear
{
private:
mutable int day; // Make 'day' mutable
// Static arrays to hold month names and the number of days in each month
static const std::string monthNames[];
static const int daysInMonth[];
public:
// Constructor
DayOfYear (int day) : day (day) {}
// Member function to print the day in month-day format
void
print () const
{
int tempDay = day; // Create a temporary variable
int month = 0;
// Find the month for the given day
while (tempDay > daysInMonth[month])
{
tempDay -= daysInMonth[month];
month++;
}
// Print the result
std::cout << monthNames[month] << " " << tempDay << std::endl;
}
};
// Static member variable initialization
const std::string DayOfYear::monthNames[] = {
"January", "February", "March", "April", "May", "June",
"July", "August", "September", "October", "November", "December"
};
const int DayOfYear::daysInMonth[] = {
31, 28, 31, 30, 31, 30,
31, 31, 30, 31, 30, 31
};
int
main ()
{
int userDay;
// Get user input for the day of the year
std::cout << "Enter the day of the year: ";
std::cin >> userDay;
// Validate user input
if (userDay < 1 || userDay > 365)
{
std::cerr << "Invalid input. Day of the year should be between 1 and 365."
<< std::endl;
return 1;
}
// Create an instance of the DayOfYear class with user input
DayOfYear userDayOfYear (userDay);
// Print the representation in month-day format
std::cout << "Day " << userDay << ": ";
userDayOfYear.print ();
return 0;
}
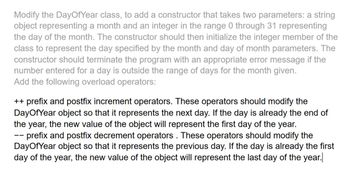

Step by stepSolved in 4 steps with 7 images

- Ajva.arrow_forwardC PROGRAMMING HELP I need help with an old script of mine. I'm having trouble getting math involving accessing an array to work properly, I'm getting an error message that's puzzling me. I'm pretty sure the solution is simple and right in front of me but it's stumping me. Below is the script: #include <stdio.h>#include <math.h>void value(float x){ printf("what is the value of x?"); scanf("%f",&x); }void power(float i,int p,int x,float arr[100]){ float equation,butt; printf("what is the highest power in the series?"); scanf("%d",&p); for (i = 0; i < p; ++i) equation = pow(x,i); //how the hell do i get the counter to do what i want butt = 1+equation^arr[i];}void shortcut(int x){ shortcut = 1/(1-x)}int main(){ printf("Here is the result!:") printf("%d %f", p, f); printf("If we had used shortcut, value would be: %d", shortcut); int diff; diff = shortcut - butt; printf("The difference is only %0.2f!",diff);…arrow_forwardThis assignment is not graded, I just need to understand how to do it. Please help, thank you! Language: C++ Given: Main.cpp #include #include "Shape.h" using namespace std; void main() { /////// Untouchable Block #1 ////////// Shape* shape; /////// End of Untouchable Block #1 ////////// /////// Untouchable Block #2 ////////// if (shape == nullptr) { cout << "What shape is this?! Good bye!"; return; } cout << "The perimeter of your " << shape->getShapeName() << ": " << shape->getPerimeter() << endl; cout << "The area of your " << shape->getShapeName() << ": " << shape->getArea() << endl; /////// End of Untouchable Block #2 //////////} Shape.cpp string Shape::getShapeName() { switch (mShapeType) { case ShapeType::CIRCLE: return "circle"; case ShapeType::SQUARE: return "square"; case ShapeType::RECTANGLE: return "rectangle"; case…arrow_forward
- class Main { // this function will return the number elements in the given range public static int getCountInRange(int[] array, int lower, int upper) { int count = 0; // to count the numbers // this loop will count the numbers in the range for (int i = 0; i < array.length; i++) { // if element is in the range if (array[i] >= lower && array[i] <= upper) count++; } return count; } public static void main(String[] args) { // array int array[] = {1,2,3,4,5,6,7,8,9,0}; // ower and upper range int lower = 1, upper = 9; // throwing an exception…arrow_forwardTrace through the following program and show the output. Show your work for partial credit. public class Employee { private static int empID = 1111l; private String name , position; double salary; public Employee(String name) { empID ++; this.name 3 пате; } public Employee(Employee obj) { empID = obj.empĪD; пате %3D оbj.naте; position = obj.position; %3D public void empPosition(String empPosition) {position = empPosition;} public void empSalary(double empSalary) { salary = empSalary;} public String toString() { return name + " "+empID + " "+ position +" $"+salary; public void setName(String empName){ name = empName;} public static void main(String args[]) { Employee empOne = new Employee("James Smith"), empTwo; %3D empOne.empPosition("Senior Software Engineer"); етрOпе.етpSalary(1000); System.out.println(empOne); еmpTwo empTwo.empPosition("CEO"); System.out.println(empOne); System.out.println(empTwo); %3D етpОпе empOne ;arrow_forwardin C code not c++arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
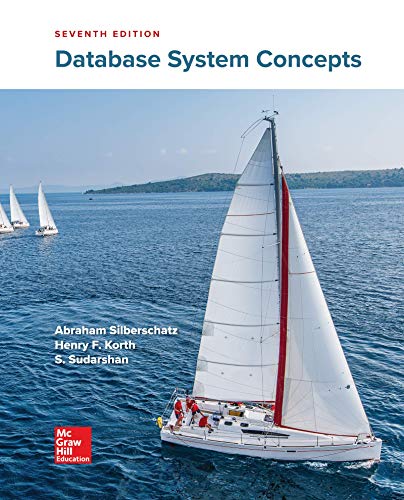
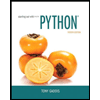
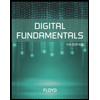
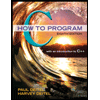
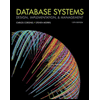
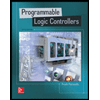