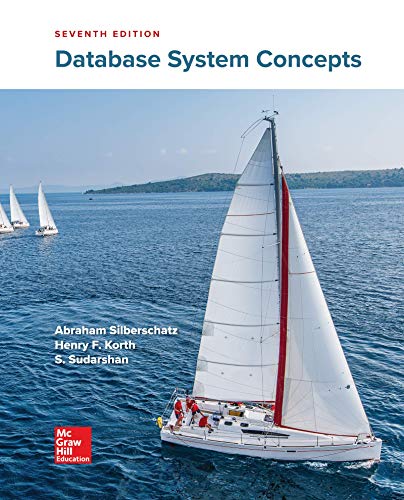
Multiple integers, representing the number of babies, are read from input and inserted into a linked list of TurtleNodes. Find the sum of all the integers in the linked list of TurtleNodes.
Ex: If the input is 3 38 18 23, then the output is:
82
import java.util.Scanner;
public class TurtleLinkedList {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
TurtleNode headTurtle = null;
TurtleNode currTurtle = null;
TurtleNode lastTurtle = null;
int count;
int inputValue;
int i;
int sum;
count = scnr.nextInt();
headTurtle = new TurtleNode(count);
lastTurtle = headTurtle;
for (i = 0; i < count; ++i) {
inputValue = scnr.nextInt();
currTurtle = new TurtleNode(inputValue);
lastTurtle.insertAfter(currTurtle);
lastTurtle = currTurtle;
}
/* Your code goes here */
System.out.println(sum);
}
}
class TurtleNode {
private int babiesVal;
private TurtleNode nextNodeRef;
public TurtleNode(int babiesInit) {
this.babiesVal = babiesInit;
this.nextNodeRef = null;
}
public void insertAfter(TurtleNode nodeLoc) {
TurtleNode tmpNext = null;
tmpNext = this.nextNodeRef;
this.nextNodeRef = nodeLoc;
nodeLoc.nextNodeRef = tmpNext;
}
public TurtleNode getNext() {
return this.nextNodeRef;
}
public int getNodeData() {
return this.babiesVal;
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- JAVA please Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts Code provided in the assignment ItemNode.java:arrow_forwardJAVA plese Implement the indexOf method in the LinkedIntegerList class public int indexOf(int value); /** * Returns whether the given value exists in the list. * @param value - value to be searched. * @return true if specified value is present in the list, false otherwise. */ } public static void main(String[] args) { // TODO Auto-generated method stub SimpleIntegerListADT myList = null; System.out.println(myList); for(int i=2; i<8; i+=2) { myList.add(i); } System.out.println(myList.indexOf(44));arrow_forwardJavaarrow_forward
- Given main() and an IntNode class, complete the IntList class (a linked list of IntNodes) by writing the insertInDescendingOrder() method to insert new IntNodes into the IntList in descending order. Ex. If the input is: 3 4 2 5 1 6 7 9 8 -1 the output is: 9 8 7 6 5 4 3 2 1 import java.util.Scanner; public class SortedList { public static void main (String[] args) {Scanner scnr = new Scanner(System.in);IntList intList = new IntList();IntNode curNode;int num; num = scnr.nextInt(); while (num != -1) {// Insert into linked list in descending order curNode = new IntNode(num);intList.insertInDescendingOrder(curNode);num = scnr.nextInt();}intList.printIntList();}}arrow_forwardCreate class Test in a file named Test.java. This class contains a main program that performs the following actions: Instantiate a doubly linked list. Insert strings “a”, “b”, and “c” at the head of the list using three Insert() operations. The state of the list is now [“c”, “b”, “a”]. Set the current element to the second-to-last element with a call to Tail() followed by a call to Previous()Then insert string “d”. The state of the list is now [“c”, “d”, “b”, “a”]. Set the current element to past-the-end with a call to Tail() followed by a call to Next(). Then insert string “e”. The state of the list is now [“c”, “d”, “b”, “a”, “e”] . Print the list with a call to Print() and verify that the state of the list is correct.arrow_forwardJava Design and draw a method called check() to check if characters in a linked list is a palindrome or not e.g "mom" or "radar" or "racecar. spaces are ignored, we can call the spaces the “separator”. The method should receive the separator as a variable which should be equal to “null” when no separator is used.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
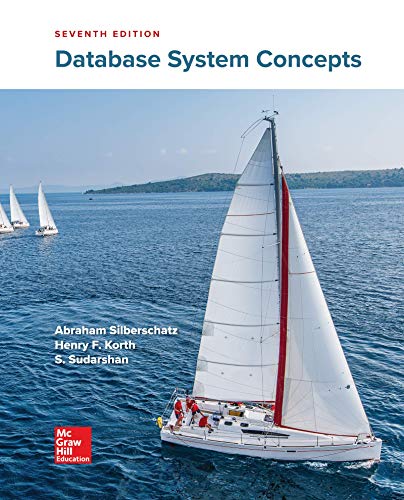
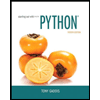
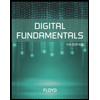
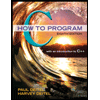
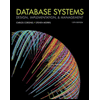
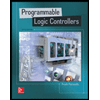