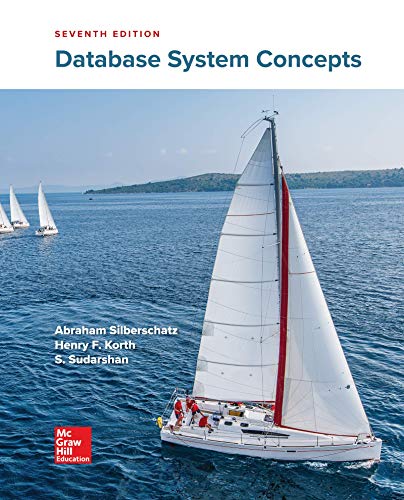
Concept explainers
My final python turtle code doesnt want to run, there is no error appearing, and there's no GUI appearing, requesting inputs for amount of lakes, mountains, and rivers, let alone opening python turtle. Help making it run?
My code:
import turtle
import random
from tkinter import Tk, Label, Entry, Button, Scale
def get_user_input():
"""
Creates a GUI using Tkinter to obtain user input for the number of lakes, mountains, and rivers.
Returns:
A tuple containing the number of lakes, mountains, and rivers (int, int, int).
"""
window = Tk()
window.title("Island Generator Settings")
lake_label = Label(window, text="Number of Lakes:")
lake_label.grid(row=0, column=0)
lake_entry = Entry(window)
lake_entry.grid(row=0, column=1)
mountain_label = Label(window, text="Number of Mountains:")
mountain_label.grid(row=1, column=0)
mountain_entry = Entry(window)
mountain_entry.grid(row=1, column=1)
river_label = Label(window, text="Number of Rivers:")
river_label.grid(row=2, column=0)
river_entry = Entry(window)
river_entry.grid(row=2, column=1)
size_scale_label = Label(window, text="Island Size (Scale)")
size_scale_label.grid(row=3, column=0)
size_scale = Scale(window, from_=1, to_=3, orient='horizontal', resolution=0.1)
size_scale.set(1) # Default size multiplier of 1
size_scale.grid(row=3, column=1, columnspan=2)
def handle_close():
window.destroy()
button = Button(window, text="Generate Island", command=handle_close)
button.grid(row=4, column=0, columnspan=3)
window.mainloop()
num_lakes = int(lake_entry.get()) if lake_entry.get() else 0
num_mountains = int(mountain_entry.get()) if mountain_entry.get() else 0
num_rivers = int(river_entry.get()) if river_entry.get() else 0
size_multiplier = size_scale.get()
return num_lakes, num_mountains, num_rivers, size_multiplier
# Define colors
ocean = "#000066"
sand = "#ffff66"
grass = "#00cc00"
lake = "#0066ff"
mountain_color = "#808080" # Gray color for mountains
def draw_circle(radius, line_color, fill_color):
"""
Draws a circle with the specified radius, line color, and fill color.
Args:
radius (int): The radius of the circle.
line_color (str): The color of the circle's outline.
fill_color (str): The color to fill the circle.
"""
my_turtle.color(line_color)
my_turtle.fillcolor(fill_color)
my_turtle.begin_fill()
my_turtle.circle(radius)
my_turtle.end_fill()
def move_turtle(x, y):
"""
Moves the turtle to the specified coordinates without drawing a line.
Args:
x (int): The x-coordinate of the destination.
y (int): The y-coordinate of the destination.
"""
my_turtle.penup()
my_turtle.goto(x, y)
my_turtle.pendown()
def draw_lake():
"""
Draws a random lake circle within the boundaries of the landmass.
Increases the size of the lake based on the size multiplier.
"""
x_limit = int(150 * size_multiplier) // 2 - 30 * size_multiplier # Adjusted for lake size
y_limit = int(120 * size_multiplier) // 2 - 30 * size_multiplier # Adjusted for lake size
x = random.randint(-x_limit, x_limit)
y = random.randint(-y_limit, y_limit)
move_turtle(x, y)

Step by stepSolved in 2 steps

- The second picture is the code. I want the output of the code to look like picture one. What part do I have to fix? THX!arrow_forwardIN PYTHON PLEASE, THANK YOU!!!arrow_forwardSelect all the statements that are true about Python's unittest module and Django's test framework: a. Django's test framework builds on Python's unittest module b. Django's TestCase class is a sub-class of unittest.TestCase c. A collection of unit tests is called a "test suite" d. Unit tests are run using the same database that the programmer uses for the development server e. HTML assert methods (such as assertHTMLEqual) are already part of Python's unittest.TestCasearrow_forward
- In Python: So this is part of a large project I am working on but I'm having a hard time finding out how to read from one text file and then correct that information to another or same txt file as well as to do it alphabetically. Any help is appreciated. The code I've added was from a previous project that was similar as to what this one wants. I can't use custom classes and I believe I'm supposed to be opening the bad information file, writing it and corrections to a temp file and then creating a new file with the right information. I'm in a beginner class and we haven't covered anything past lists. Problem #1: How much should I study outside of class? Issue: Your fellow students liked the 2nd version of study hour’s application and want to expand it again by adding the features listed below. Minimum Study Hours per Week per Class Grade 15 A 12…arrow_forwardI have a python programming question Create a package called geometry. Inside that package create two modules: rectangle.py and triangle.py. Also create an empty file with name __init__.py in the geometry package.Inside rectangle.py define three functions:1. rectArea(): this function takes two parameters length and width of a rectangle, calculates and returns the area.2. rectCircumference(): this function takes two parameters length and width of a rectangle, calculates and returns the circumference.3. isSquare(): this function takes two parameters length and width of a rectangle and returns true if they are equal otherwise returns false.Inside triangle.py define two functions:1. triArea(): this function takes two parameters base and height of a triangle, calculates and returns the area.2. triCircumference(): this function takes three parameters, three sides of a triangle and returns the circumference.Create main.py in the same directory as the geometry package. Import your modules.…arrow_forwardi am trying to write code for c#. the gui is set up. i have 3 list boxes each with 3 items and each item has a different price. customer should select one from each list box and then be presented with a total price. i'm struggling to know what is the first thing i do. i have the using information, namespace......do i begin after Initialize Component?arrow_forward
- Here is step one in my python project In this step we'll be translating a single letter to Morse Code and a single Morse Code to a letter. The only input validation will be on the menu selection to handle the case where the user has entered something other than 0, 1 or 2. We'll import a module of our own (provided for you) and we'll use the dictionaries in that module to do the translation. 1. Download the morse module to your folder. 2. Create your step_1.py program and import morse --> morse.py Download morse.py 3. Create your menu driven interface using a while loop with the following menu: Morse Code Translator 0: Exit 1: Translate a word into Morse Code 2: Translate Morse Code to text. 4. Use an if-elif-else construct, with 'else' explaining to the user that the selection was invalid and that only 0, 1 and 2 are acceptable inputs. 5. If the user enters "0", exit the program. 6. If the user enters "1": Prompt the user for a letter with the input() command and…arrow_forwardPython code and output screenshot is mustarrow_forwardI need a code that demonstrates the use of the below items (Templates, overloading, and vectors). It doesn't need to be special and you can choose the example for the code. Maybe 30 - 50 lines of code that I can run in Visual Basic 2019. I just want to be able to compile it so that I understand what I am reading next week. Working examples are better than what I can pull from the single code examples in the book. Please label to help me identify. Thanks 1. Write C++ code (perhaps around 30-50 lines of code) and make it compile and run showing how to use: a. Templates b. Overloading c. Vectorsarrow_forward
- This is the given code. I can't pass the unit test. Please help me recall that using python.arrow_forwardFor c++. Need help designing class, no namespsace. FoodWastageRecord NOTE: You need to design this class. It represents each food wastage entry recorded by the user through the form on the webpage (frontend). If you notice the form on the webpage, you’ll see that each FoodWastageRecord will have the following as the data members aka member variables. Date (as string) Meal (as string) Food name (as string) Quantity in ounces (as double) Wastage reason (as string) Disposal mechanism (as string) Cost (as double) Each member variable comes with its accessor/mutator functions. --------------------------------------------------------------------------------- FoodWastageReport NOTE: You need to design this class. It represents the report generated on the basis of the records entered by the user. This class will be constructed with all the records entered by the user as a parameter. It will then apply the logic to go over all the records and compute the following: Names of most commonly…arrow_forwardAttached below is a task for coding in Python. Please assist in writing out the code, thank you. Comments are also provided indicating where each step goes.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
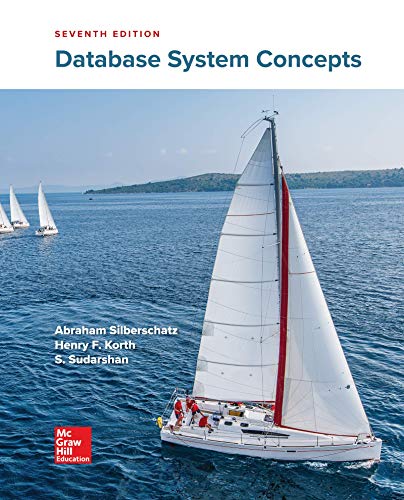
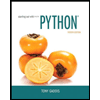
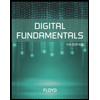
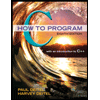
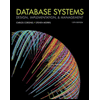
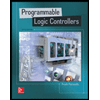