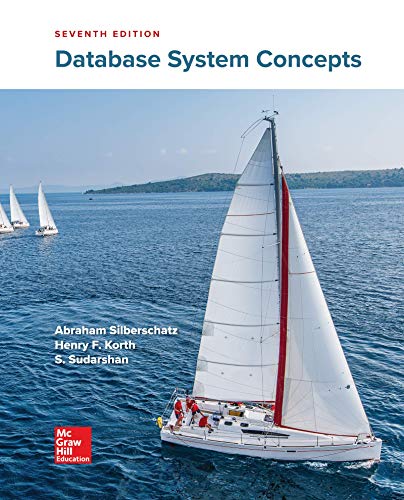
My issue:
I wrote my questions in bold in the comments of the code
Java code:
import java.util.Scanner;
public class DescendingOrder {
// TODO: Write a void method selectionSortDescendTrace() that takes
// an integer array and the number of elements in the array as arguments,
// and sorts the array into descending order.
public static void selectionSortDescendTrace(int [] numbers, int numElements) {
//my code starts here
int i;
int j;
int elementIndex;
int temp;
//loop in ascending order using textbook example
for (i=0; i < numElements-1; ++i) {
index=i;
for (j=i+1; j < numElements; ++j) {
if (numbers[j] < numbers[elementIndex]) {
elementIndex=j;
}
}
//swap using textbook example
temp=numbers[i];
numbers[i]=numbers[elementIndex];
numbers[elementIndex]=temp;
//I don't know how to put it in descending order. Is it using a loop?
//where do I put in descending order? Is it in the method definitions or main method ???
}
}
//my code ends here
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
int input, i = 0;
int numElements = 0;
int [] numbers = new int[10];
// TODO: Read in a list of up to 10 positive integers; stop when
// -1 is read. Then call selectionSortDescendTrace() method.
//my code starts here
for (i=0; i < numbers.length; ++i) {
input=scnr.nextInt();
numbers[i]=input;
if (numbers[i]==-1) {
break;
numElements++;
}
//selectionSortDescendTrace(); //what goes inside the parenthesis???
//my code ends here
}
}
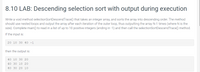
![Selection sort has the advantage of being easy to code, involving one loop nested within another loop, as shown below.
Figure 8.6.1: Selection sort algorithm.
public class SelectionSort {
public static void selectionSort (int [] numbers) {
int i;
int j;
int indexSmallest;
int temp;
// Temporary variable for swap
for (i = e; i« numbers.length
1; ++i) {
// Find index of smallest remaining element
indexSmallest = i;
for (j = i + 1; j< numbers.length; ++j) {
if (numbers[j] < numbers [indexSmallest]) {
indexSmallest - j;
}
// Swap numbers[i] and numbers[indexSmallest]
temp = numbers[i];
numbers[i] = numbers[indexSmallest];
numbers[indexSmallest] = temp;
UNSORTED: 10 2 78 4 45 32 7 11
SORTED: 2 4 7 10 11 32 45 78
public static void main(String [] args)
int numbers [] = {10, 2, 78, 4, 45, 32, 7, 11};
int i;
System.out.print ("UNSORTED: ");
for (i = e; i < numbers.length; ++i)
System.out.print (numbers[i] +
System.out
In()3B
/* initial call to selection sort with index */
selectionSort(numbers);
System.out.print("SORTED: ");
for (i = e; i < numbers.length; ++i) {
System.out.print (numbers[i] + " ");
System.out.println();
Feedback?
Selection sort may require a large number of comparisons. The selection sort algorithm runtime is O(N2). If a list has N elements, the outer
loop executes N -1 times. For each of those N -1 outer loop executions, the inner loop executes an average of * times. So the total](https://content.bartleby.com/qna-images/question/c14768d3-3359-407d-85b4-624ecac227c9/92779156-44f9-4ce0-990d-4585e12ab813/wm1css_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- import java.util.Arrays; import java.util.Random; public class Board { /** * Construct a puzzle board by beginning with a solved board and then * making a number of random moves. That some of the possible moves * don't actually change what the board looks like. * * @param moves the number of moves to make when generating the board. */ public Board(int moves) { throw new RuntimeException("Not implemented"); } The board is 5 X 5. You can add classes and imports like rand.arrow_forwardIn Java: Write a method that multiplies all elements in the array by 3 and returns it to the main method.arrow_forwardin javaarrow_forward
- Method Details: public static java.lang.String getArrayString(int[] array, char separator) Return a string where each array entry (except the last one) is followed by the specified separator. An empty string will be return if the array has no elements. Parameters: array - separator - Returns: stringThrows:java.lang.IllegalArgumentException - When a null array parameter is providedarrow_forwardHow do I make the code run properly? Code: import java.util.*; import java.util.Arrays; public class Movie { private String movieName; private int numMinutes; private boolean isKidFriendly; private int numCastMembers; private String[] castMembers; // default constructor public Movie() { this.movieName = "Flick"; this.numMinutes = 0; this.isKidFriendly = false; this.numCastMembers = 0; this.castMembers = new String[10]; } // overloaded parameterized constructor class movie{public static void main(String args[]){Movie obj=new Movie();Movie obj1=new Movie(obj.getMovieName(),obj.getNumMinutes(),obj.isKidFriendly(),obj.getCastMembers());System.out.println("Default Constructor: "+obj.getNumCastMembers());System.out.println("Overloaded Constructor: "+obj1.getNumCastMembers());}} // set the number of minutes public void setNumMinutes(int numMinutes) { this.numMinutes = numMinutes; } // set the movie name public void setMovieName(String movieName) { this.movieName = movieName; } // set if the…arrow_forwardWrite a Java method (do not write a class, only the method) with the following signature: Return type: void Parameters: 2D integer array Name: printDiagonal Implement the method code that will print out the values of the 2D array along the Diagonal from left to right. For example, if the array had the following values: 56 78 98 21 33 55 98 44 11 The method should print to the console: 56,33,11 The above 2D array example is only given for you to understand the problem Do not hard code the values in your solution or assume the array is the same as the given data. Your method should work for all 2D integer arrays.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
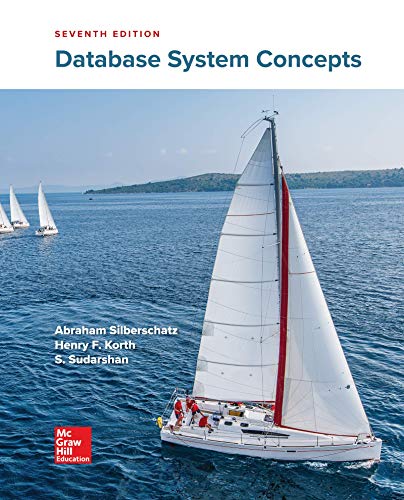
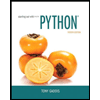
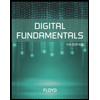
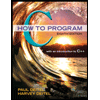
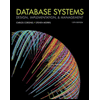
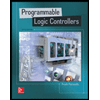