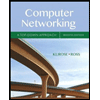
Objective: Write a Java program practicing the use of arrays in addition to the other programming practices you have learned, such as program decomposition and the use of Java class (static) methods. Monolithic solutions that do not exhibit good design practices and appropriate class methods will not receive full credit.
Use arrays for this. Do not use ArrayLists.
Material Provided: You will need the following file to complete this lab:
- info.txt (A sample input file, which is available on Carmen.)
Set up
You will need to create your own implementation of the Student class. It will look much like the Student class we saw in the class PowerPoint slides. You may take some shortcuts though. Knowing that the data will always be sets of four lines of text which contain a student name and three scores, you can get away with a constructor that takes the name and three scores and creates the instance of the Student object. (You will still need to get the data out of it though, so don’t forget those methods.)
Also, given that you will be reading the number of students in the “class group” as the first item of data read from the file, you can allocate the array to hold the students in the program. After that you can read the data in groups of four lines, to get the complete set of data for each student, and allocate new memory for that data in your array.
Hints:
- for loops will be helpful in this assignment.
- Read all input as Strings. Use Integer.parseInt(String integer_value) to get the numeric value from it.
- To create each new element in the array of students, use the new keyword and the Student constructor. I.e., studentList[i] = new Student(line, Integer.parseInt(line1),…
- Don’t worry about methods in the main program. The objective here is to write a Student class, which will have a few instance methods in it. (My solution has two for loops in it with the necessary input and output statements to read from the file and print to the screen,
- The Student class file will look a lot like the Student class file in the set of notes on classes and objects. Put it under the same package as the class with main in it, so they are effectively side by side. Remember that it won’t have a main (or much of anything else except the class instance variables and methods themselves.)
- Don’t forget to include the “throws IOException” in the proper place.
- Remember that the backslash is a special character, so when you use it to enter a path name (such as “C:\\info.txt”) in Eclipse, you must enter a pair of them. Otherwise Windows will drop a single backslash and you get an incorrect path. (Later versions of Eclipse actually interpret the single backslash properly, it appears.) Other programming environments may handle this differently, and the path for a Macintosh is also different from this. Macintoshes use something like “users/user_name/documents/file_name”.
Description
Here is a sample interaction between a user and the program you will write in the exercise. (User input is in bold.)
Enter file name: c:\\info.txt
Name Score1 Score2 Score3 Total
-----------------------------------------------------
Andy Borders 200 250 400 850
John Smith 120 220 330 670
John Borell 250 250 500 1000
Robert Fennel 200 150 350 700
Craig Fenner 230 220 480 930
Bill Johnson 120 150 220 490
Brent Garland 220 240 350 810
-----------------------------------------------------
The total number of student in this class is: 7
The average total score of the class is: 778
John Borell got the maximum score of: 1000
Bill Johnson got the minimum score of: 490
-----------------------------------------------------
Note that the filename supplied includes the full path. Specify a full path or the IDE will not find the file.
The program performs the following actions:
- Ask the user for the name of a file containing information about student scores and input the file name. (See “Input file format” for a description of the format of the file.)
- Input the student information from the file and store in an appropriate array of Student objects. See “Setup” for a description of this.
- Output a well-formatted table containing the name, individual scores and total score for each student.
- Output the number of students in the class, the average total score, the maximum total score and the minimum total score in the class, including the names of the students with the maximum and minimum scores.
To tabulate the output, you can count spaces or use tab characters. A tab character can be printed by using the ’\t’ escape sequence. For example, System.out.print(”1\t2\t3”) will output something like “1 2 3” where 1, 2 and 3 are separated by tabs.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Hello! I need some help with my Java homework. Please use Eclipse Please add comments to the to program so I can understand what the code is doing and learn Create a new Eclipse project named so as to include your name (eg smith15 or jones15). In this project, create a new package with the same name as the project. In this package, write a solution to the exercise noted below. Implement the following method that returns the maximum element in an array: public static <E extends Comparable<E>> E max(E[] list) Write a test program that generates 10 random integers, invokes this method to find the max, and then displays the random integers sorted smallest to largest and then prints the value returned from the method. Max sure the the last sorted and returned value as the same!arrow_forwardNon dynamic.arrays are allocated at runtime where dynamic arrays are allocated at compile time. True False Question 4 There are other ways to end a loop other than just the loop condition evaluating to false. True Falsearrow_forwardIn this assignment, you will decide how to keep the inventory in the text file. Then your program must read the inventory from the file into the array. Each product must have a record in the file with the name, regular price, and type. There are several options for storing records in the file. For example, • each value takes one line in the file (i.e., three lines for one product). Then you must take care of correct handling of the ends of the lines; • all values are in one line that can be read as a string. Then you must handle the parsing of the string; • all values are in one line separated by a delimiter. Then you must handle a line with delimiters. Assume that the inventory does not have more than 100 products. But the actual number is known only after the reading of the file. Once you can read data from the file into the array, you must add a new property to the product class – a static variable that holds the number of products in the inventory. Its value must grow as reading…arrow_forward
- What transpires when a parameter for an array is sent in with either the ref or out keyword, and the results are observed?arrow_forwardI NEED HELP PLEASE NO REGEX OR ANYTHING COMPLICATED PLEASE.. USE BASIC APPROACH Create a program in Python that reads data from Breakfast Menu (https://www.w3schools.com/xml/simple.xml) and builds parallel arrays for the menu items, with each array containing the menu item name, description, calories, and price, respectively. After reading the data and building the arrays, display the menu items similar to the following: name - description - calories - priceAt the bottom, display the total number of items on the menu, the average number of calories per item, and the average price per item similar to: 0 items - 0 average calories - $0.00 average price You may either read the page using Internet processing methods, or you may download and save the page and then read the data from the saved file. You must process the data using string functions (no XML libraries). must use separate subroutines/functions/methods to implement each type of processing, and include error handling for…arrow_forwardStep 1: Read your files! You should now have 3 files. Read each of these files, in the order listed below. staticarray.h -- contains the class definition for StaticArray, which makes arrays behave a little more like Python lists O In particular, pay attention to the private variables. Note that the array has been defined with a MAX capacity but that is not the same as having MAX elements. Which variable indicates the actual number of elements in the array? staticarray.cpp -- contains function definitions for StaticArray. Right now, that's just the print() member function. 0 Pay attention to the print() function. How many elements is it printing? main.cpp -- client code to test your staticarray class. Note that the multi-line comment format (/* ... */) has been used to comment out everything in main below the first print statement. As you proceed through the lab, you will need to move the starting comment (/*) to a different location. Other than that, no changes ever need to be made to…arrow_forward
- I NEED HELP PLEASE NO REGEX OR ANYTHING COMPLICATED PLEASE.. USE BASIC APPROACH Create a program in Python that reads data from Breakfast Menu (https://www.w3schools.com/xml/simple.xml) and builds parallel arrays for the menu items, with each array containing the menu item name, description, calories, and price, respectively. After reading the data and building the arrays, display the menu items similar to the following: name - description - calories - priceAt the bottom, display the total number of items on the menu, the average number of calories per item, and the average price per item similar to: 0 items - 0 average calories - $0.00 average price You may either read the page using Internet processing methods, or you may download and save the page and then read the data from the saved file. You must process the data using string functions (no XML libraries). must use separate subroutines/functions/methods to implement each type of processing, and include error handling for…arrow_forwardTASK 1. Prior to writing your code, you will create an input file for this assignment yourself and name it input.txt. Open the CreateInput.java file, attached to this assignment in Blackboard and analyze its code. Find the 2D array of items and add three more grocery items and their type of your choice into this array. In the same code, find an array of countries and add two more countries of your choice to this 1D array.arrow_forwarduse the array built to shuffle and deal two poker hands. Change the corresponding values for all Jacks through Aces to 11, 12, 13, 14 respectively. Shuffle the deck, then deal two hands. Comment your code and submit the Python code. i wrote a code for it but its not displaying properly, can someone tell me why? (black and white is the array given/ the picture of the python app is what i wrote) its only displaying the array given and not the bottom code i put, why?arrow_forward
- Section A. Declare and initialize an array myChars containing the following characters: B, C, D, A, M, S and F.Remember to choose the correct data type for the array elements.Section B. Using a WHILE loop, output the elements of the array myChars so that they are displayed on thesame line and separated by a space. Use .length to get the length of the array.Section C. Change the last value of the array myChars to the letter X. Use .length in your code.Section D. Using a FOR loop, output the elements of the myChars array so that they are displayed on the sameline and separated by a dash (an extra dash at the end is okay). Use .length in your code.Section E. Declare an array of int of capacity 10 named thisArray.Section F. Use a FOR loop to populate the array thisArray with the following numbers 5, 10, 15, 20, 25. Thinkof a way to insert these numbers without actually writing “5” or “10” and so on, but by using the variable i ofyour FOR loop. NOTE that this is a partially-filled array and…arrow_forwardDue in C languagearrow_forwardWhich of the following are true about arrays? Arrays are reference types. Array indexing is bounds‐checked. Arrays sometimes can be created without using new. The entire contents of an array can be copied via =.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
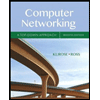
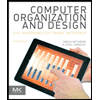
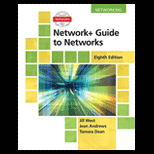
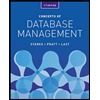
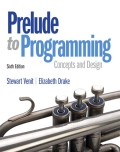
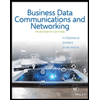