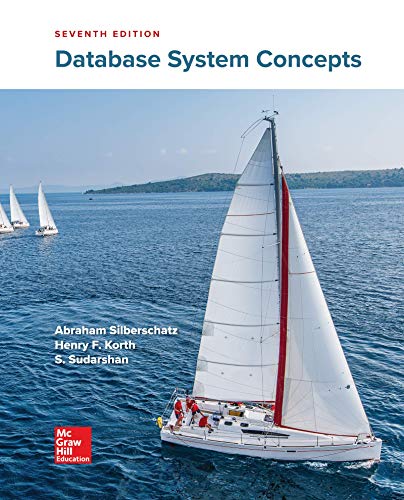
public class Geometry
{
public static void main(String[] args)
{
//asks for user's choice
Scanner in = new Scanner(System.in);
displayMenu();
selectOption(choice);
System.out.println("Enter your choice (1-3): ");
int choice1 = in.nextInt();
System.out.println("Thanks for using the Geometry Calculator - Goodbye!");
//Prints if input is a number that is not one of the choices
while(choice1 < 1 || choice1 > 3 )
{
System.out.println("Invalid choice. Please enter 1 - 3: ");
choice1 = in.nextInt();
}
}
/**
Displays the menu
*/
public static void displayMenu()
{
System.out.println("Welcome to the Geometry Calculator Application");
System.out.println("1. Calculate the Area of a Circle");
System.out.println("2. Calculate the Area of a Rectangle");
System.out.println("3. Calculate the Area of a Triangle");
}
/**
This method calculates the area of the circle
@return the area of the circle
*/
public static double circle()// calculates the area of the circle
{
Scanner in = new Scanner(System.in);
System.out.println("What is the circle's radius? ");
double radius = in.nextDouble();
double circleArea = Math.PI * radius * radius;
return circleArea;
}
/**
This method calculates the area of rectangle
@result the area of the rectangle
*/
public static double rectangle()// calculates the area of the rectangle
{
Scanner in = new Scanner(System.in);
System.out.println("What is the rectangle's length? ");
double length = in.nextDouble();
System.out.println("What is the rectangle's width? ");
double width = in.nextDouble();
double rectangleArea = length * width;
return rectangleArea;
}
//calculates the area of a triangle
/**
This method calculates the area of a triangle
@return the area of the triangle
*/
public static double triangle()
{
Scanner in = new Scanner(System.in);
System.out.println("What is the length of the triangle's base? ");
double base = in.nextDouble();
System.out.println("What is the triangle's height? ");
double height = in.nextDouble();
double triangleArea = 0.5 * base * height;
return triangleArea;
}
//prints area to two decimal places
/**
This method prints the area
@param the area's value
*/
public static void printArea(double a)
{
System.out.printf("The area is "+ "%.2f" + a);
}
//assigns each choice to a method
/**
This method assigns each choice to an area method
@param choice of the method
*/
public static void selectOption(int choice)
{
if(choice==1)
{
circle();
printArea(circleArea()); //prints circle's area if the choice is #1
}
else if(choice==2)
{
rectangle();
printArea(rectangleArea); //prints rectangle's area if the choice is #2
}
else if(choice==3)
{
triangle();
printArea(triangleArea); //prints triangle's area if the choice is #3
}
}
}
This is my code for calculating the area.
These are the errors I get.
Geometry.java:17: error: cannot find symbol
selectOption(choice);
^
symbol: variable choice
location: class Geometry
Geometry.java:109: error: cannot find symbol
printArea(circleArea); //prints circle's area if the choice is #1
^
symbol: variable circleArea
location: class Geometry
Geometry.java:114: error: cannot find symbol
printArea(rectangleArea); //prints rectangle's area if the choice is #2
^
symbol: variable rectangleArea
location: class Geometry
Geometry.java:119: error: cannot find symbol
printArea(triangleArea); //prints traingle's area if the choice is #3
^
symbol: variable triangleArea
location: class Geometry
4 errors
I would appreciate help in this!

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- public class KnowledgeCheckTrek { public static final String TNG = "The Next Generation"; public static final String DS9 = "Deep Space Nine"; public static final String VOYAGER = "Voyager"; public static String trek(String character) { if (character != null && (character.equalsIgnoreCase("Picard") || character.equalsIgnoreCase("Data"))) { // IF ONE return TNG; } else if (character != null && character.contains("7")) { // IF TWO return VOYAGER; } else if ((character.contains("Quark") || character.contains("Odo"))) { // IF THREE return DS9; } return null; } public static void main(String[] args) { System.out.println(trek("Captain Picard")); System.out.println(trek("7 of Nine")); System.out.println(trek("Odo")); System.out.println(trek("Quark")); System.out.println(trek("Data").equalsIgnoreCase(TNG));…arrow_forwardpublic static void printIt(int value) { if(value 0) { } System.out.println("Play a paladin"); System.out.println("Play a white mage"); }else { } }else if (value < 15) { System.out.println("Play a black mage"); System.out.println("Play a monk"); } else { public static void main(String[] args) { printit (5); printit (10); printit (15); Play a black mage Play a white mage (nothing/they are all printed) Play a paladin Play a monkarrow_forwardComplete the isExact Reverse() method in Reverse.java as follows: The method takes two Strings x and y as parameters and returns a boolean. The method determines if the String x is the exact reverse of the String y. If x is the exact reverse of y, the method returns true, otherwise, the method returns false. Other than uncommenting the code, do not modify the main method in Reverse.java. Sample runs provided below. Argument String x "ba" "desserts" "apple" "regal" "war" "pal" Argument String y "stressed" "apple" "lager" "raw" "slap" Return Value false true false true true falsearrow_forward
- int stop = 6; int num =6; int count=0; for(int i = stop; i >0; i-=2) { num += i; count++; } System.out.println("num = "+ num); System.out.println("count = "+ count); } }arrow_forwarddef area(side1, side2): return side1 * side2s1 = 12s2 = 6Identify the statements that correctly call the area function. Select ALL that apply. Question options: area(s1,s2) answer = area(s1,s2) print(f'The area is {area(s1,s2)}') result = area(side1,side2)arrow_forwardprivate Vector location;private Vector velocity;private Vector acceleration;private int lifespan;private static final int MAX_LIFESPAN = 25;private static final int RADIUS = 7; public boolean isAlive() { } public void update() {//code here} Using java, if particle is alive then add velocity vector to location vector, add acceleration vector to velocity vector, and decrement lifespan by 1. Thank you.arrow_forward
- complete the missing code. public class Exercise09_04Extra { public static void main(String[] args) { SimpleTime time = new SimpleTime(); time.hour = 2; time.minute = 3; time.second = 4; System.out.println("Hour: " + time.hour + " Minute: " + time.minute + " Second: " + time.second); } } class SimpleTime { // Complete the code to declare the data fields hour, // minute, and second of the int type }arrow_forwardpublic class Course { private String courseNumber, courseTitle; public Course() { } public Course(String courseNumber, String courseTitle) { this.courseNumber = courseNumber; this.courseTitle = courseTitle; } public void setCourseNumber(String courseNumber) { this.courseNumber = courseNumber; } public void setCourseTitle(String courseTitle) { this.courseTitle = courseTitle; } public String getCourseNumber() { return courseNumber; } public String getCourseTitle() { return courseTitle; } public void printInfo() { System.out.println("Course Information: "); System.out.println(" Course Number: " + courseNumber); System.out.println(" Course Title: " +…arrow_forwardFind the error(s) in the following class definition: public class Circle private double radius; public double setRadius(double a) { radius = a; public void getRadius() { return radius;arrow_forward
- public class Ex08FanTest { public static void main (String[] args){ Ex08Fan fan1= new Ex08Fan(); Ex08Fan fan2= new Ex08Fan(); fan1.setOn(true); fan1.setSpeed(3); fan1.setRadius(10); fan1.setColor("yellow"); fan2.setOn(true); fan2.setSpeed(2); fan2.setRadius(5); fan2.setColor("blue"); fan2.setOn(false); System.out.println("Fan 1:\n" + fan1.toString()); System.out.println("\nFan 2:\n" + fan2.toString()); }}arrow_forwardclass Bug { private final int orgPosition = 0; private int currentPosition; private boolean direction; public Bug() { direction = true; currentPosition = orgPosition; } public void move() { if (direction) { ++currentPosition; } else { --currentPosition; } } public void turn() { direction = !direction; } public int getPosition() { return currentPosition; } public boolean getDirection() { return direction; } public String toString() { StringBuffer sb = new StringBuffer(); sb.append("Position = " + currentPosition + ", Direction = "); sb.append(direction ? "RIGHT" : "LEFT"); return sb.toString(); } } -------------------------------------TestDriver.java------------------------------------- import java.util.Scanner; class TestDriver { public static void main(String args[]) { Scanner input = new Scanner(System.in); Bug b…arrow_forwardpublic class Test { } public static void main(String[] args) { int a = 5; a += 5; } switch(a) { case 5: } System.out.print("5"); break; case 10: System.out.print("10"); System.out.print("0"); default:arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
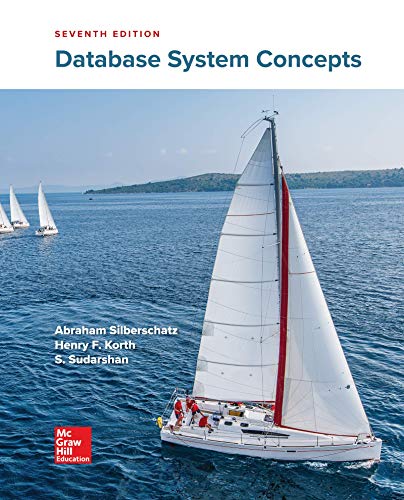
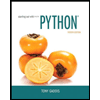
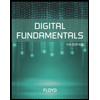
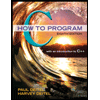
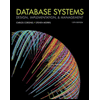
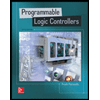