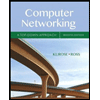
(Java)
- Open up Eclipse and create a new class called ArrayListPractice.java
- Next, copy and paste the below program into your file and run the code.
- Your job is to take the given code, remove all the arrays and replace them with the identical ArrayLists. There should be no arrays in your program.
- You will need to call the ArrayList methods as defined in the lesson notes above.
- Note that you will not be able to do method overloading with ArrayLists so you should assign different names to your methods.
- Once you have made the changes, you should get identical output as the given version of the program.
- Submit your program when you are finished.
/**
* @author
* CIS 36B
* Activity 5.2
*/
import java.util.ArrayList;
import java.util.Scanner;
public class ArrayListPractice {
public static void main(String[] args) {
int scores[] = {95, 96, 97, 98, 99};
System.out.println("Integer exam scores:");
print(scores);
System.out.println();
System.out.println("After adding extra credit:");
addExtraCredit(scores);
print(scores);
System.out.println();
double rainfall[] = {23.4, 16.4, 18.9, 52.7};
System.out.println("Rainfall in Inches:");
print(rainfall);
System.out.println();
String vowels[] = {"a", "e", "i", "o", "u"};
System.out.println("Vowels in the Latin Alphabet:");
print(vowels);
System.out.println();
}
/**
* Prints an array of integer values
* @param values the array of ints
*/
public static void print(int values[]) {
for (int i = 0; i < values.length - 1; i++) {
System.out.print(values[i] + ", ");
}
System.out.println(values[values.length-1]);
}
/**
* Prints an array of double values
* @param values the array of doubles
*/
public static void print(double values[]) {
for (int i = 0; i < values.length - 1; i++) {
System.out.print(values[i] + ", ");
}
System.out.println(values[values.length-1]);
}
/**
* Prints an array of String values
* @param values the array of Strings
*/
public static void print(String values[]) {
for (int i = 0; i < values.length - 1; i++) {
System.out.print(values[i] + ", ");
}
System.out.println(values[values.length-1]);
}
/**
* Add 5 to each element in an integer array
* @param values the array of ints
*/
public static void addExtraCredit(int values[]) {
for(int i = 0; i < values.length; i++) {
values[i]+=5;
}
}
}
95, 96, 97, 98, 99
After adding extra credit:
100, 101, 102, 103, 104
Rainfall in Inches:
23.4, 16.4, 18.9, 52.7
Vowels in the Latin Alphabet:
a, e, i, o, u

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

- Using Java: (Sum the areas of geometric objects) Write a method that sums the areas of all thegeometric objects in an array. The method signature is:public static double sumArea(GeometricObject[] a) Write a test program that creates an array of four objects (two circles and tworectangles) and computes their total area using the sumArea methodarrow_forward(Flattening arrays with flatten vs. ravel) Create a 2-by-3 array containing the first six powers of 2 beginning with 2^0. Flatten the array first with method flatten, then with ravel. In each case, display the result then display the original array to show that it was unmodified. Please use Python and keep it simple.arrow_forward(Java) Q5arrow_forward
- (Java in Eclipse) Write a method that returns the sum of all the elements in a specified column in a 2-D array using the following header: public static int sumCol(int[][] m, int colIdx) Included is a test class and a sample runarrow_forward(Intro to Java) Open a new Java file called ArrayMethods.java. Read the Javadoc comment for each method below and write the method according to the description in the comment Once you are getting the correct output for all tests inside of main, upload your source file to Canvas public class ArrayMethods { /** * Displays the contents of an array on the console * with each element separated by a blank space * Prints a new line character last * @param nums the array to print to the console */ public static void printArray(int[] nums) { return; } /** * Assuming an array of integers, return true if 1 * is the first element or 1 is the last element * Note: you may assume that you will be given an array * of at least length 1 * is1FirstLast([1, 2, 10]) → true * is1FirstLast([10, 1, 2, 1]) → true * is1FirstLast([13, 10, 1, 2, 3]) → false * @param numbers the array of int numbers * @return whether or not 1 is the first or…arrow_forward(in java) Add the following class methods to the program below A method that asks for the username and password of the user. Note: Because we do not have any database, just assume all username/password combinations are valid, so set the isAuthenticated variable to true and print a login successful message inside this method with the value of isAuthenticated variable. A method that prints Top 5 Trending items in ascending order. (Use Arrays.sort() library method to quickly sort arrays). A method that prints logged in username, remaining balance and subscriber since information. A method (like updateContinueWatching(String strValue) which takes a String parameter and updates the Continue Watching array for the user. So in NetflixDemo class, take a movie or TV show name as input from the user and pass it to this updateContinueWatching(value) method. Print the final Continue Watching array. Note: New value should take first position in the Continue Watching array (index 0).…arrow_forward
- (True/False): The ArraySum procedure (Section 5.2.5) receives a pointer to any array ofdoublewords.arrow_forward(Convert decimals to fractions)Write a program that prompts the user to enter a decimal number and displays the number in a fraction.Hint: read the decimal number as a string, extract the integer part and fractional part from the string, and use the Rational class in LiveExample 13.13 to obtain a rational number for the decimal number. Use the template athttps://liveexample.pearsoncmg.com/test/Exercise13_19.txt The problem can not use BigInteger //Below is the Rational LiveExample 13.13 that goes with the problem/question; notice "long" instead of "BigInteger" class Rational extends Number implements Comparable<Rational> { // Data fields for numerator and denominator private long numerator = 0; private long denominator = 1; /** Construct a rational with default properties */ public Rational() { this(0, 1); } /** Construct a rational with specified numerator and denominator */ public Rational(long numerator, long denominator) { long gcd = gcd(numerator,…arrow_forward(Car Class) Create a class called Car that includes three instance variables-a model (type String), a year (type String), and a price (double). Provide a constructor that initializes the three instance variables. Provide a set and a get method for each instance variable. If the price is not positive, do not set its value. Write a test application named CarApplication that demonstrates class Car's ca- pabilities. Create two Car objects and display each object's price. Then apply a 5% discount on the price of the first car and a 7% discount on the price of the second. Display each Car's price again. 3.13arrow_forward
- (Data exploration and Mining Method Proposal): Here you will explore your data both visually and/or statistically then propose methods for mining it. I- Present a simple statistical exploration of your data (correlations, standard deviations, means, medians). Also explore your data visually using methods such as histograms, scatterplots ,heat maps, box and whisker plots...) II- Propose a data mining method covered in the class to apply on your data.arrow_forward(In Java language) Create a class Called NetflixDemo Create two objects “user1” and “user2” of the Netflix class pictured below) Set various class variables for the Netflix class using these two objects. Call various methods of Netflix class using these two objects. public class Netflix {public String movies [] = new String[10];public String trendingTop5 [] = new String[5];public String continueWatching [] = new String[5];public String username;public String password;private boolean isAuthenticated;public double balance;public int subscriberSinceYear;public static void logininfo() {Scanner input = new Scanner(System.in);System.out.println("Enter the username");String username = input.nextLine();System.out.println("Enter the password");String password = input.nextLine();boolean isAuthenticated = true;System.out.println(isAuthenticated + " , You have successfully logged in ");System.out.println();}public static void trendingList(){Scanner input = new…arrow_forward(Java) Open up Eclipse and create a new class called ArrayListPractice.java Next, copy and paste the below program into your file and run the code. Your job is to take the given code, remove all the arrays and replace them with the identical ArrayLists. There should be no arrays in your program. You will need to call the ArrayList methods as defined in the lesson notes above. Note that you will not be able to do method overloading with ArrayLists so you should assign different names to your methods. Once you have made the changes, you should get identical output as the given version of the program. Submit your program when you are finished. /** * @author * CIS 36B * Activity 5.2 */ import java.util.ArrayList; import java.util.Scanner; public class ArrayListPractice { public static void main(String[] args) { int scores[] = {95, 96, 97, 98, 99}; System.out.println("Integer test scores:"); print(scores); System.out.println();…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
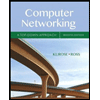
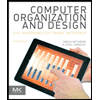
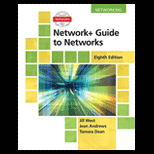
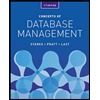
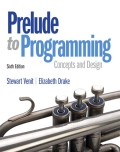
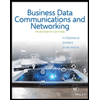