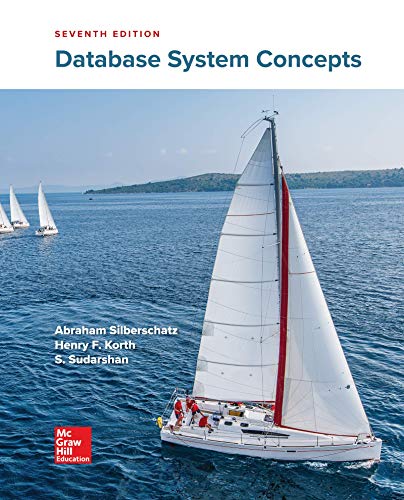
Concept explainers
(Java)
- Open up Eclipse and create a new class called ArrayListPractice.java
- Next, copy and paste the below program into your file and run the code.
- Your job is to take the given code, remove all the arrays and replace them with the identical ArrayLists. There should be no arrays in your program.
- You will need to call the ArrayList methods as defined in the lesson notes above.
- Note that you will not be able to do method overloading with ArrayLists so you should assign different names to your methods.
- Once you have made the changes, you should get identical output as the given version of the program.
- Submit your program when you are finished.
/**
* @author
* CIS 36B
* Activity 5.2
*/ import java.util.ArrayList;
import java.util.Scanner;
public class ArrayListPractice {
public static void main(String[] args) {
int scores[] = {95, 96, 97, 98, 99};
System.out.println("Integer test scores:");
print(scores);
System.out.println();
System.out.println("After adding extra credit:");
addExtraCredit(scores);
print(scores);
System.out.println();
double rainfall[] = {23.4, 16.4, 18.9, 52.7};
System.out.println("Rainfall in Inches:");
print(rainfall);
System.out.println();
String vowels[] = {"a", "e", "i", "o", "u"};
System.out.println("Vowels in the Latin Alphabet:");
print(vowels);
System.out.println(); }
/**
* Prints an array of integer values
* @param values the array of ints
*/ public static void print(int values[]) {
for (int i = 0; i < values.length - 1; i++) {
System.out.print(values[i] + ", "); }
System.out.println(values[values.length-1]); }
/**
* Prints an array of double values
* @param values the array of doubles
*/
public static void print(double values[]) {
for (int i = 0; i < values.length - 1; i++) {
System.out.print(values[i] + ", "); }
System.out.println(values[values.length-1]); }
/**
* Prints an array of String values
* @param values the array of Strings
*/ public static void print(String values[]) {
for (int i = 0; i < values.length - 1; i++) {
System.out.print(values[i] + ", "); }
System.out.println(values[values.length-1]); }
/**
* Add 5 to each element in an integer array
* @param values the array of ints
*/ public static void addExtraCredit(int values[]) {
for(int i = 0; i < values.length; i++) {
values[i]+=5;
}
}
}
Your Program Should Give the Identical Output to the Output Below:
Integer test scores: 95, 96, 97, 98, 99
After adding extra credit: 100, 101, 102, 103, 104
Rainfall in Inches: 23.4, 16.4, 18.9, 52.7
Vowels in the Latin Alphabet:
a, e, i, o, u

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- (Game: ATM machine) Use the Account class created in our previous Lab Exercise to simulate an ATM machine. Create ten accounts in an array with id 0, 1, . . ., 9, and initial balance $100. The system prompts the user to enter an id. If the id is entered incorrectly, ask the user to enter a correct id. Once an id is accepted, the main menu is displayed as shown in the sample run. You can enter a choice 1 for viewing the current balance, 2 for withdrawing money, 3 for depositing money, and 4 for exiting the main menu. Once you exit, the system will prompt for an id again. Thus, once the system starts, it will not stop.arrow_forward(With java please)arrow_forward(PLEASE USE JAVA AND JFRAME GUI) Game rules:The game consists of a two-dimensional field of size m × n (game field). Each element ofthe game field is a button with a number assigned to it.Initialization of the game:• The numbers on the buttons of the game field are set to a random digit between 0and 9• The target value is displayed above the game field• The current sum of the numbers displayed on the buttons of the game field is printedbelow the game field• The number of moves to completion is displayed in the upper right corner above thegame field.Playing:The first move is determined by the player by selecting any button (button A) on thegame field.1. The player is allowed to choose a second button (button B) located in the columnor row of the previously selected button (A).2. Upon selecting the second button (B), the value of button (A) is updated accordingto the following formula: A = (AoperationB)mod10.3. The operation for the basic game will be +.4. Decrement the number of moves…arrow_forward
- Using Java: (Sum the areas of geometric objects) Write a method that sums the areas of all thegeometric objects in an array. The method signature is:public static double sumArea(GeometricObject[] a) Write a test program that creates an array of four objects (two circles and tworectangles) and computes their total area using the sumArea methodarrow_forward(Intro to Java) Explain the answers to the below questions. include a written answer to the question using step-by-step explanation 1. Write a method called arrayTimesFive The method takes one array of doubles as a parameter It multiplies each element in the array by 5 and stores the result It returns nothingarrow_forwardHello! I need some help with my Java homework. Please use Eclipse Please add comments to the to program so I can understand what the code is doing and learn Create a new Eclipse project named so as to include your name (eg smith15 or jones15). In this project, create a new package with the same name as the project. In this package, write a solution to the exercise noted below. Implement the following method that returns the maximum element in an array: public static <E extends Comparable<E>> E max(E[] list) Write a test program that generates 10 random integers, invokes this method to find the max, and then displays the random integers sorted smallest to largest and then prints the value returned from the method. Max sure the the last sorted and returned value as the same!arrow_forward
- task-5 Change Summation Integers problem to Finding the minimumproblem. Make sure you properly wrote/updated all text messages, method names,and math calculations.Hint: You can use java.lang.Math.min() method.Example: System.out.printf("The min of the integers %4d and %4d and %4dis %7d\n", a, b, c, MinTest(a, b, c));Submit the source code files (.java files) and the console output screenshotsarrow_forward(Java) Q5arrow_forwardWrite a simple cash register class. (Use Java) Each cash register should keep track of the number of items checked out and the total cash in the register. We are only concerned with the number of items to check out and their prices. No need to handle item names, SKUs, taxes, etc... It should have the following methods (use static when appropriate): checkout( ) Takes two forms. If there is only one parameter that is the cash received and there is only one item checked out. If it has two parameters, the first parameter should have the number of items checked out and the second parameter is the price per item. cashout( ) Reset the number of items and total cash to zero for a cash register getItems( ) Returns the number of items in the cash register getTotalCash( ) Returns the total cash in the cash register getAveragePricePerItem( ) Returns the average price per item checked out by the cash register getRegisterCount( ) Returns the number of cash registers created getAllItems(…arrow_forward
- (IllegalArgumentException)Programming defined the Triangle class with three sides. In the a triangle the sum of any two sides is greater then than the other side. Create IllegaTriangleException class, and modify the constructor of Triangle classto throw an IllegaTriangleException object if a triangle is created with sides that violate the rule, as follows: /** Construct a tringle the specified sides*/ public Triangle(double side1, double side2, double side3) throws IllegaTriangleException{ //Implementarrow_forwardtask_6 Change Summation Integers problem to Finding the minimumproblem. Make sure you properly wrote/updated all text messages, method names,and math calculations.Hint: You can use java.lang.Math.min() method.Example: System.out.printf("The min of the integers %4d and %4d and %4dis %7d\n", a, b, c, MinTest(a, b, c));Submit the source code files (.java files) and the console output screenshotsarrow_forward(in java) Add the following class methods to the program below A method that asks for the username and password of the user. Note: Because we do not have any database, just assume all username/password combinations are valid, so set the isAuthenticated variable to true and print a login successful message inside this method with the value of isAuthenticated variable. A method that prints Top 5 Trending items in ascending order. (Use Arrays.sort() library method to quickly sort arrays). A method that prints logged in username, remaining balance and subscriber since information. A method (like updateContinueWatching(String strValue) which takes a String parameter and updates the Continue Watching array for the user. So in NetflixDemo class, take a movie or TV show name as input from the user and pass it to this updateContinueWatching(value) method. Print the final Continue Watching array. Note: New value should take first position in the Continue Watching array (index 0).…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
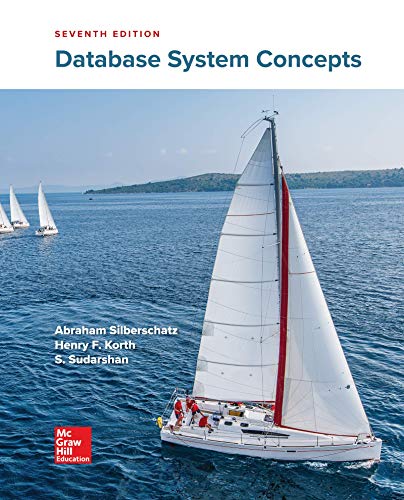
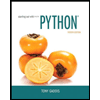
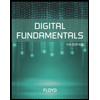
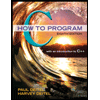
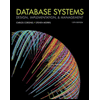
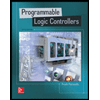