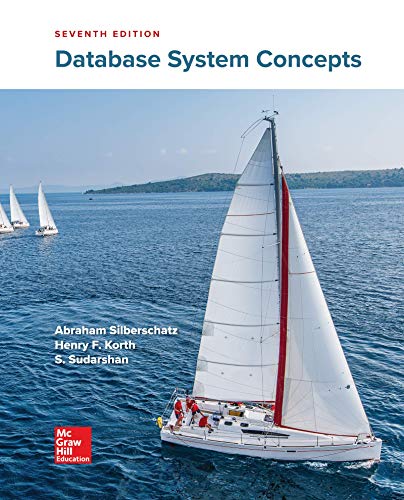
Concept explainers
java
random matches. Write a BinarySearch client that takes an int value T as
a command-line argument and runs T trials of the following experiment for N = 103, 104,
105, and 106: generate two arrays of N randomly generated positive six-digit int values,
and find the number of values that appear in both arrays. Print a table giving the average
value of this quantity over the T trials for each value of N.

The experiment aims to study the number of overlaps between two randomly generated integer arrays for varying sizes. Specifically, for each array size , we generate two arrays of random six-digit integers and count the number of integers that appear in both arrays. By repeating this experiment times, we can derive an average number of overlaps for each array size .
Algorithm:
- Parse the command-line argument to get the value of .
- Initialize a list of array sizes .
- For each size in the list, execute the following steps:
- Initialize a variable to zero.
- Repeat times:
- Generate two random arrays of size .
- Sort the first array.
- For each element in the second array, perform a binary search on the sorted first array to check for its existence.
- Increment the totalMatches by the number of elements found.
- Compute the average number of overlaps for as
.
- Print the average number for .
Step by stepSolved in 5 steps with 3 images

- Strings all_colors and updated_value are read from input. Perform the following tasks: Split all_colors into tokens using a comma (',') as the separator and assign color_list with the result. Replace the first element in color_list with updated_value.arrow_forwardWrite a program named GradeStats.java then implement the following method: public static int[] gradeStats(int[] arr) It accepts int array representing test grades for CISY432 then calculates and returns the following grade statictics: highest, lowest, average, number of grades that are greater than or equal to 70, the number of grades that are less than 70 It returns these five numbers as an int array, and the 1st element is for highest, 2nd for lowest, 3rd for average 4th element for number of grades that are greater than or equal to 70 5th for the number of grades that are less than 70 For example. O Type here to search DELLarrow_forwardYou have the following vector x. You can copy and paste this directly into Matlab or octave online. x = [614 6421361333415455411346236116625352552342 43462322433422523224355133513432333444513452 3644552454565325421324432554254213]' Use the reshape command to reshape the vector x into a square matrix, find the determinant of the resulting matrix.arrow_forward
- Exception in thread "main" java.lang.NumberFormatException: For input string: "x" for Java code public class Finder { //Write two recursive functions, both of which will parse any length string that consists of digits and numbers. Both functions //should be in the same class and have the following signatures. //use the if/else statement , Find the base case and -1 till you get to base case //recursive function that adds up the digits in the String publicstaticint sumIt(String s) { //if String length is less or equal to 1 retrun 1. if (s.length()<= 1){ return Integer.parseInt(s); }else{ //use Integer.praseInt(s) to convert string to Integer //returns the interger values //else if the CharAt(value in index at 0 = 1) is not equal to the last vaule in the string else {//return the numeric values of a char value + call the SumIt method with a substring = 1 return Character.getNumericValue(s.charAt(0) ) + sumIt(s.substring(1)); } } //write a recursion function that will find…arrow_forwardIN Java Overloaded Sorting. In class, we have primarily used integer arrays asexamples when demonstrating how to sort values. However, we can sort arrays madeof other primitive datatypes as well.In this assignment, you will create three arrays of size 8; one array will be an integerarray, one will be a char array, and one will be a float array. You will then ask the userto state what kind of data they want to sort – integers, chars, or floats.The user will then input 8 values. You will store them in the appropriate array based onwhat datatype they initially stated they would use.You will create a function called sortArray() that takes in an integer array as aparameter, and two overloaded versions of the same function that take in a char arrayand float array as parameters respectively. You will use these functions to sort theappropriate array and display the sorted values to the user.Note: You must make overloaded functions for this assignment – they must all be calledsortArray(). You…arrow_forwardWrite a Java class that creates a 3x4 array of 12 random ints between 0 and 100. Write a method to return the sum of the elements in the last column of each row in the two-dimensional array.arrow_forward
- Write the function divideArray() in script.js that has a single numbers parameter containing an array of integers. The function should divide numbers into two arrays, evenNums for even numbers and oddNums for odd numbers. Then the function should sort the two arrays and output the array values to the console. Ex: The function call: let nums = [4, 2, 9, 1, 8]; divideArray(nums); produces the console output: Even numbers: 2 4 8 Odd numbers: 1 9 The program should output "None" if no even numbers exist or no odd numbers exist. Ex: The function call: let nums = [4, 2, 8]; divideArray(nums); produces the console output: Even numbers: 2 4 8 Odd numbers: None Hints: Use the push() method to add numbers to the evenNums and oddNums arrays. Supply the array sort() method a comparison function for sorting numbers correctly. To test your code in your web browser, call divideArray() from the JavaScript console.arrow_forwardThe code in the picture takes two sorted arrays of sizes m and n, then it merges and sorts them. Whats the best-case and worst-case for this alogrithm?arrow_forwardWrite a complete Java program named Transpose that contains the following: The main method asks the user to provide n - the number of rows and m – the number of columns for a rectangular matrix of integers from 2 to 10. The main method calls the matrixSetUp() method which creates a rectangular matrix of specified dimensions and populates it with random integers from 0 to 99. Hint: You can use Math.random() to generate random numbers. The main method prints the matrix created by the matrixSetUp () method in the form of a matrix. Hint: in order to print the array in the example format, you can use Arrays.toString(matrix[row]) to print each row. The main method calls the matrixTranspose() method that creates and returns a transpose of the initial matrix. Hint: matrix transpose means: ����������[���][������]=�[������][���] The main method prints the transpose of the matrix.arrow_forward
- Write a Java program that will allow the user to play a hangman game repeatedly until theychoose to stop. The game should randomly choose a secret word from a pre-set list of wordsfor the user to guess. Store the list of words available for play in an array so you can randomlychoose them by index during game play.During a turn, the user will guess one letter at a time. Each letter in the secret word shoulddisplay as an asterisk until the letter is guessed by the user. When a correct letter is guessed,the letter should be displayed in the correct position in place of the asterisk. The user hangshimself with the 8th wrong guess and loses the game.Make a character array to hold the characters and asterisks representing the word beingguessed. You can change the contents of this array as the letters are guessed and revealed. Inother words, this array can be used to display the current state of the secret word each round.Here is the list of words the game should choose from for the secret…arrow_forwardHere is the iterative implementation of binary search: For each call to binary_search below, indicate how many times the code inside the while loop will execute. animals = ["aardvark", "cat", "dog", "elephant", "panda"] 1. binary_search("elephant", animals) 2. binary_search("dog", animals) 3. binary_search("anteater", animals)arrow_forwardJAVA: Use the "natural split" algorithm on the file split.txt and answer the following question: How many elements are in the first list? Split.txt file 200494 774 644 345 521 61 27 28 584 569 66 857 210 2 211 675 548 596 188 647 671 517 408 475 615 113 731 522 850 17 640 940 556 517 602 935 76 578 880 428 168 394 381 497 247 368 137 138 619 973 895 106 838 45 921 800 681 660 490 427 937 917 786 959 152 604 837 749 684 963 926 963 988 492 385 458 788 7 62 46 932 996 787 634 34 616 71 128 686 578 923 532 933 545 100 224 895 595 342 933 934 797 120 565 495 884 237 648 843 599 157 843 192 279 301 649 382 881 667 178 126 746 44 601 22 52 769 708 991 83 447 786 525 168 328 922 153 367 22 689 834 774 93 110 547 597 644 726 237 20 370 573 391 225 750 897 956 839 951 455 8 509 746 338 576 276 277 341 274 891 723 53 966 271 50 990 493 823 833 306 619 461 768 832 736 759 920 451 549 833 911 123 907 225 252 179 177 272 747 859arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
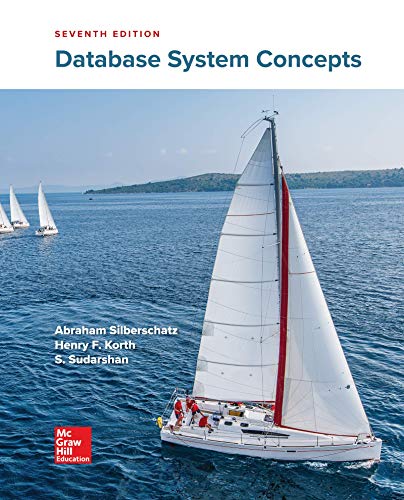
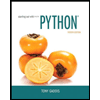
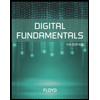
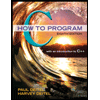
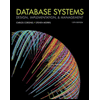
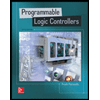