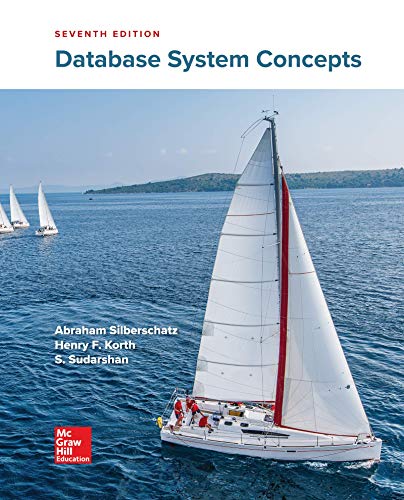
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
![### Pseudocode for Finding the Kth Smallest Element in a Binary Search Tree (BST)
The task is to implement the `FindKth` function on a Binary Search Tree (BST). This function should accept a pointer to the root of a BST and an integer K. The function returns the Kth smallest value in the BST if one exists or -99999 otherwise. Assume that K is indexed starting at 0. Do not assume that height information is stored at each node.
#### Example
For example, `FindKth(a, 3)` would return the value `13` for the following BST:
#### Diagram Explanation
The BST is represented as follows:
- The root of the tree is `13`.
- The left child of `13` is `11`.
- The right child of `13` is `15`.
- The left child of `11` is `10`.
- The right child of `11` is `12`.
- The left child of `15` is `14`.
```
13
/ \
11 15
/ \ /
10 12 14
```
### Sample Pseudocode
To find the Kth smallest element in the BST, follow these steps:
1. Perform an in-order traversal of the BST.
2. Keep track of the number of nodes visited.
3. When K nodes have been visited, return the value of the current node.
#### Pseudocode Implementation
```
function FindKth(node, K):
# This list will hold the current count of visited nodes and the value of the Kth node
result = [-99999, -1] # result[0] will be updated with the value of the Kth smallest element
# Helper function to perform in-order traversal
function in_order_traversal(node, K, result):
if node is None:
return
# Traverse the left subtree
in_order_traversal(node.left, K, result)
# Visit the current node
result[1] += 1
if result[1] == K:
result[0] = node.value
return
# Traverse the right subtree
in_order_traversal(node.right, K, result)
# Start in-order traversal from the root node
in_order_traversal(node, K, result)](https://content.bartleby.com/qna-images/question/c9d2e553-8b23-4042-8a74-de309176cab2/4762c8a6-7a27-40cc-86c1-b87360a8b725/42npyad_thumbnail.png)
Transcribed Image Text:### Pseudocode for Finding the Kth Smallest Element in a Binary Search Tree (BST)
The task is to implement the `FindKth` function on a Binary Search Tree (BST). This function should accept a pointer to the root of a BST and an integer K. The function returns the Kth smallest value in the BST if one exists or -99999 otherwise. Assume that K is indexed starting at 0. Do not assume that height information is stored at each node.
#### Example
For example, `FindKth(a, 3)` would return the value `13` for the following BST:
#### Diagram Explanation
The BST is represented as follows:
- The root of the tree is `13`.
- The left child of `13` is `11`.
- The right child of `13` is `15`.
- The left child of `11` is `10`.
- The right child of `11` is `12`.
- The left child of `15` is `14`.
```
13
/ \
11 15
/ \ /
10 12 14
```
### Sample Pseudocode
To find the Kth smallest element in the BST, follow these steps:
1. Perform an in-order traversal of the BST.
2. Keep track of the number of nodes visited.
3. When K nodes have been visited, return the value of the current node.
#### Pseudocode Implementation
```
function FindKth(node, K):
# This list will hold the current count of visited nodes and the value of the Kth node
result = [-99999, -1] # result[0] will be updated with the value of the Kth smallest element
# Helper function to perform in-order traversal
function in_order_traversal(node, K, result):
if node is None:
return
# Traverse the left subtree
in_order_traversal(node.left, K, result)
# Visit the current node
result[1] += 1
if result[1] == K:
result[0] = node.value
return
# Traverse the right subtree
in_order_traversal(node.right, K, result)
# Start in-order traversal from the root node
in_order_traversal(node, K, result)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- A singly linked list contains n - 1 strings that are binary representations of numbers from the set {0, 1,.…, n – 1} where n is an exact power of 2. However, the string corresponding to one of the numbers is missing. For example, if n = 4, the list will contain any three strings from 00, 01,10 and 11. Note that the strings in the list may not appear in any specific order. Also note that the length of each string is lgn, hence the time to compare two strings in O(lgn). Write an algorithm that generates the missing string in O(n).arrow_forwardPlease use the alphabet shown in the screenshot below to code a Depth-first search (DFS) algorithm in C++.arrow_forward
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
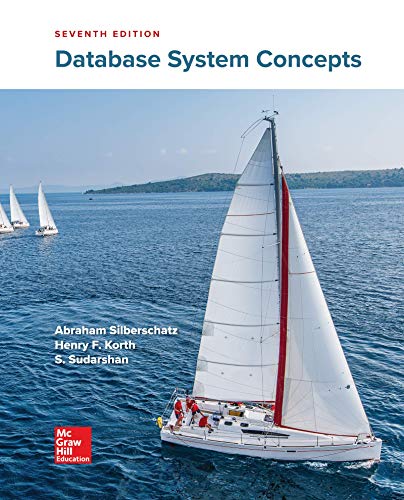
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
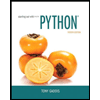
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
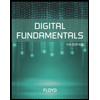
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
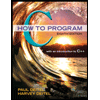
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
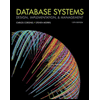
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
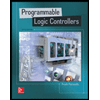
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education