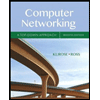
(python)
1. Create a function that converts minutes into seconds.
INPUT: the value in minutes
OUTPUT: Nothing is output
RETURN: the value in seconds.
2. Create a function that converts hours to seconds.
INPUT: the value in hours
OUTPUT: Nothing is output
RETURN: the value in seconds.
Bonus 1 Point: Use the method from #1 to help convert the value.
3. Create a function that calculates the points based on the teams record. This function will accept three parameters (wins, loss, ot loss). The team is awarded 2 points for a win, 0 for a loss and 1 for an ot loss.
INPUT: the win, loss, and ot loss values
OUTPUT: Nothing is output
RETURN: the value in points.
4. Create a function that only outputs the menu options.
INPUT: Nothing to input
OUTPUT: the menu of options
RETURN: Nothing is returned
5. Create a main method/main if statement.
6. Call the function from #4. Then allow the user to enter a value to select one of the options.
7. Based on the input, check that value and create if statements to check the input.
8. Based on the input, get the needed additional input from the user and call the function
Bonus Point: Add a 4th option to quit and loop until they select that option.

Step by stepSolved in 4 steps with 6 images

- Fill in the Blank Word GameThis exercise involves creating a fill in the blank word game. Try prompting the user to enter a noun,verb, and an adjective. Use the provided responses to fill in the blanks and then display the story.First, write a short story. Remove a noun, verb, and an adjective.Create a function that asks for input from the user.Create another function that will fill the blanks in the story you’ve just created.Ensure that each function contains a docstring.After the noun, verb, and adjective have been collected from the user, display the story that has beencreated using their input. use python to ode.arrow_forwardAlert ⚠️ You guys use AI tool to answer. Last time I found plagiarism and AI detection in my answer. Now If you will use these things I'll surely give multiple downvotes and will report sure.arrow_forward# Exercise 8: Create a function that takes the sum of three numbers and returns the value # Make sure to use the function and display the result # When you are done, get 3 numbers from a user and then print out the sum using your functionarrow_forward
- # NumberFun.py # using multiple functions. # copy/paste this program to run it first, you are supposed to use Google Chrome as Internet Browser for this course. # 1. calculate sum of the first n natural numbers, e.g.:1,2,3,... def sumN(n): sumN = 0 for i in range(1,n+1): sumN = sumN + i return sumN # 2. calculate sum of the square of the first m natural numbers, e.g.:1,4,9,... ### After you define/write/complete the function sumMSquare(m), remove the # sign as below to ENABLE next line of code ####def sumMSquare(m):### YOUR TURN TO DEFINE/WRITE/COMPLETE function sumMSquare(m) as below, based on sumN(n) # 3. call two functions defined previously within main() function def main(): print("This program computes the total and total of squares of the first") print("N/M natural numbers.\n") n,m = input("Please enter a value for N and M: ").split(",") print("The total of the first", n, "natural numbers is", sumN(int(n)))### After you define/write/complete the function sumMSquare(m), remove…arrow_forwardPython Programming Only (PLEASE INCLUDE INPUT VALIDATION IF NEEDED) Write a function named maximum that accepts two integer values as arguments and returns the value that is the greater of the two. For example, if two numbers are one big and one small the function should return the bigger number. Use the function in a program that prompts the user to enter two integer values. The program should display the value that is the greater of the two.arrow_forwardProgram Unit Score Calculator Console App Write a Python Console Application program that allows the user to enter the marks for different assessments in a unit, and computes the total mark and grade for the unit. Here is the program logic specification: There are six assessment activities Quiz1, Quiz2, Quiz3, Quiz4, Lab Journal, Major Assignment and Final Exam. The four quizzes are worth 5 marks each, the Lab Journal is worth 10 marks, the Major Assignment is worth 30 marks and the Final Exam is worth 40 marks. The algorithm for computing the total mark for the unit is: Total Mark = Quiz1+Quiz2+Quiz3+Quiz4+Major Assignment+ Lab Journal + Final Exam The following screenshot shows a successful test run:arrow_forward
- The code is not printing the correct result. Please explain to me step by step.arrow_forwardpython module must define four functions:a. add(a, b)b. subtract(a, b)c. multiply(a, b)d. divide(a, b)i. Divide must check for division by zero and return an error message if itencounters it.3. Each function should take two arguments and perform the corresponding mathematicaloperation. Return the result, don’t print it in the function.4. Include a main() function that tests these functions with some example inputs. Ensurethat you print the results of the function calls here in the main function and provide theappropriate labels.5. The main() function must only run automatically if the module is being run directly, notwhen imported.arrow_forwardQuestion 6 # This function checks whether a given number is even or not def isEven(number): """ what it takes? a number what it does? determines if a number is even what it returns? if even, return 1 if odd, return -1 """ # your code goes in here defisEven(number): #check if the number is divisible by 2 by checking the remainder. if number % 2==0: #If remainder is 0, then its divisble by 2 and an even number #hence return-1 return1 else: #if remainder is not 0, it'll be 1, so its NOT divisible by 2 and its an odd number #hence return -1 return-1 Test case for question 6 try: if isEven(5) == -1: score['question 6'] = 'pass' else: score['question 6'] = 'fail' except: score['question 6'] = 'fail' isEven(5)arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
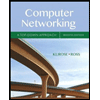
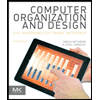
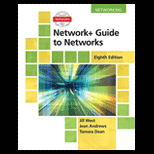
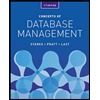
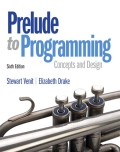
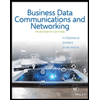