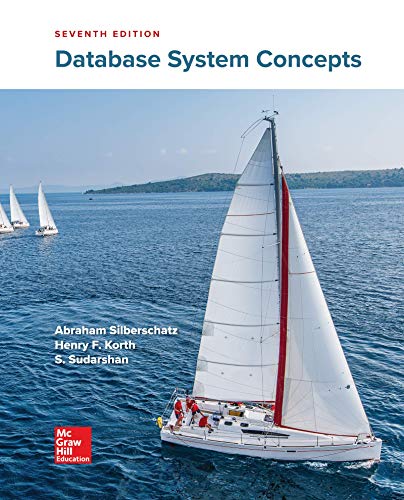
Python Programming (code)
Write a class named "Fraction" that represents a rational number (a number that can be expressed as the quotient of two integers). Implement the following methods:
- The __init__(self, numerator, denominator) method should accept integer values for the numerator and denominator arguments and set instance attributes of the same name. If the denominator is 0, raise a ZeroDivisionErrorexception. Use the [math.gcd](https://docs.python.org/3/library/ math.html#math.gcd) function to find the greatest common divisor (GCD) of the numerator and de- nominator and then divide each of them by it to "normalize" the fraction. For example, the fraction 28/20 will get normalized to 7/5 since the GCD of 28 and 20 is 4:
>>> x = Fraction(28, 20)
>>> x
Fraction(7, 5)
- Implement the basic binary operators (+,-,*,/) so that a Fraction can be combined with either another fraction or an integer. All methods should return a new Fraction. Note that you may need to implement "reversed" operators for arithmetic with integers to fully work.
- The __neg__ method should return a new Fraction instance with the numerator negated.
- The __repr__ method should return a string of the form Fraction(a, b) where a and b are the numerator and denominator, respectively.
--------------------------------------------------------------------------
Few test cases:
>>> from fraction import Fraction
>>> frac_1 = Fraction(28,20)
>>> frac_
Fraction(7, 5)
>>> frac_2 = Fraction(1,0)
Traceback (most recent call last):
raise ZeroDivisionError
fraction.ZeroDivisionError: Denominator cannot be zero
>>> frac_2 = Fraction(1,5)
>>> frac_1 + frac_
Fraction(8, 5)
>>> frac_1 * frac_
Fraction(7, 25)
>>> frac_2 - frac_
Fraction(-6, 5)
>>> frac_2 / frac_
Fraction(1, 7)
>>> frac_2 / 2
Fraction(1, 10)
>>> frac_1 / 2
Fraction(7, 10)
>>> frac_1 * 2
Fraction(14, 5)
>>> -frac_
Fraction(-1, 5)
>>> 2 - frac_
Fraction(9, 5)
>>> 2 - (-frac_2)
Fraction(11, 5)
--------------------------------------------------------------------------
Required Output:
class Fraction:
def __init__(self, numerator. denominator):
pass
def __neg__():
pass
def __repr__():
pass

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 6 images

- Question: Submit document with methods for your automobile class, and pseudo code indicating functionality of each method. Example: public String RemoveVehicle(String autoMake, String autoModel, String autoColor, int autoYear) If values entered match values stored in private variables remove vehicle information else return message indicating mismatch Assignment details/background Create an automobile class that will be used by a dealership as a vehicle inventory program. The following attributes should be present in your automobile class: private string makeprivate string modelprivate string colorprivate int yearprivate int mileage Your program should have appropriate methods such as: default constructorparameterized constructoradd a new vehicle methodlist vehicle information (return string array)remove a vehicle methodupdate vehicle attributes method. All methods should include try..catch constructs. Except as noted all methods should return a…arrow_forwardIn Java Write a Fraction class that implements these methods:• add ─ This method receives a Fraction parameter and adds the parameter fraction to thecalling object fraction.• multiply ─ This method receives a Fraction parameter and multiplies the parameterfraction by the calling object fraction.• print ─ This method prints the fraction using fraction notation (1/4, 21/14, etc.)• printAsDouble ─ This method prints the fraction as a double (0.25, 1.5, etc.)• Separate accessor methods for each instance variable (numerator , denominator ) in theFraction classProvide a driver class (FractionDemo) that demonstrates this Fraction class. The driver class shouldcontain this main method : public static void main(String[] args) { Scanner stdIn = new Scanner(System.in); Fraction c, d, x; // Fraction objects System.out.println("Enter numerator; then denominator."); c = new Fraction(stdIn.nextInt(), stdIn.nextInt()); c.print(); System.out.println("Enter numerator; then denominator."); d = new…arrow_forwardIn python and include doctring: First, write a class named Movie that has four data members: title, genre, director, and year. It should have: an init method that takes as arguments the title, genre, director, and year (in that order) and assigns them to the data members. The year is an integer and the others are strings. get methods for each of the data members (get_title, get_genre, get_director, and get_year). Next write a class named StreamingService that has two data members: name and catalog. the catalog is a dictionary of Movies, with the titles as the keys and the Movie objects as the corresponding values (you can assume there aren't any Movies with the same title). The StreamingService class should have: an init method that takes the name as an argument, and assigns it to the name data member. The catalog data member should be initialized to an empty dictionary. get methods for each of the data members (get_name and get_catalog). a method named add_movie that takes a Movie…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
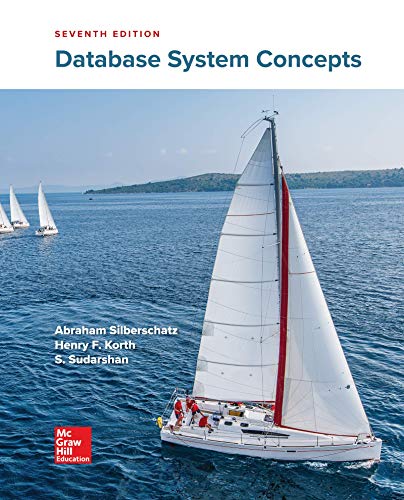
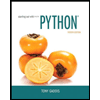
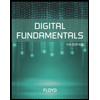
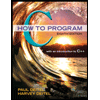
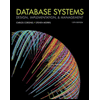
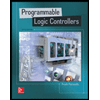