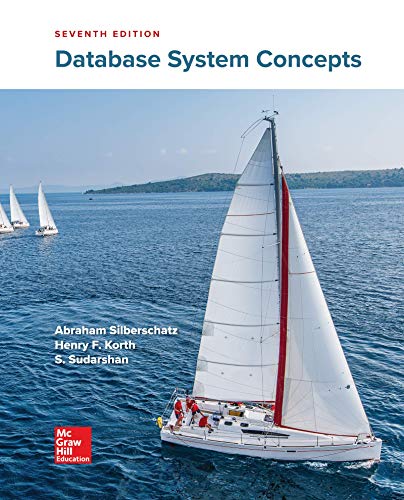
Concept explainers
PYTHON QUESTION
This assignment requires you to create a dictionary by reading the text file created for the Chapter 6 assignment. The dictionary should have player names for the keys. The value for each key must be a two-element list holding the player's goals and assists, respectively. See page 472! Start with an empty dictionary. Then, use a loop to cycle through the text file and add key-value pairs to the dictionary. Close the text file and process the dictionary to print the stats and determine the top scorer as before. In fact, much of the code used in program6_2.py can be copied and used for this program. Printing the stats for each player is the most challenging part of this program. To master this, refer to the examples in the zip file that can be downloaded from the "Dictionary values can be lists" link in the "Learn Here" part of this module. NOTE: you do not need to submit the text file. Submit just this program. The required output should be the same well-formatted table as was generated by program_62.py.
- PLAYER COLUMN: 10 characters wide and left-aligned.
- GOALS COLUMN: 6 characters wide and centered.
- ASSISTS COLUMN: 8 characters wide and centered.
- TOTAL COLUMN: 6 characters wide and centered.
Sample Output
(See Attached Photo)
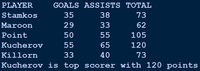

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- You are to write a short program using dictionaries. Create a dictionary object that will store key value pairs of cars and prices. The list of items are as follows. Tesla M3 = 67,000, Lexus RX400 = 53,000, Infinity G12= 14,000, Ford F150= 60,000, Honda Civic= 31,500. 1) First step is to save the data in the dictionary called cars. 2) Second step is for your program to ask the user to enter which car do they want to buy using the input method. The user may enter "Ford 150". 3) Third step is locating the car in the cars dictionary object. 4) Next step is to get the price from the dictionary to show to the user in the following format. "The car you selected Ford 150 is for $60,000 and with tax the total cost will be $64,200" You should plan to use 7% for tax calculation. It could easily be calculated as 60000*.07 + 60000 = 64200arrow_forwardCounting numbers are the numbers 1, 2, 3, and so on. Create a dictionary in Python that maps the first n counting numbers to their squares. Assign the dictionary to the variable squares.arrow_forwardCreate a program that asks the user to enter a series of city names and populations. Each name and population should be added to a dictionary as a key/value pair. Once they are finished entering these values, use a dictionary comprehension to create a new dictionary featuring only the cities whose populations are over 2 million. Print the key/value pairs in the resulting dictionary out.arrow_forward
- Perform the following tasks: Create a dictionary named color_dict containing key-value pairs 'red': 222, 'green': 184, and 'blue': 135. Read strings key_read and value_read from input. Add key_read and value_read as a new key-value pair to color_dict with key_read as the key and value_read as the value.arrow_forwardCreate a dictionary (order_dict) that the key is the burger number, and the value is a list of the quantities. For example: order_dict which is {'1': [10, 3, 78], '2': [35, 0, 65], '3': [9, 23, 0], '4': [0, 19, 43], '5': [43, 0, 21]} Create a dictionary (total_order_dict) that the key is the burger number, and the value is the total of the quantities for that burger. For example: total_order_dict which is {'1': 91, '2': 100, '3': 32, '4': 62, '5': 64} Use "assert to test the values of the total_order_dict For example: expected_result_ototal_order_dict = {'1': 91, '2': 100, '3': 32, '4': 62, '5': 64} assert actual_result_ototal_order_dict['1'] == expected_result_ototal_order_dict['1'] , "The actual result is not the \same as expected result for the order number 1!" (and test the other elements) The function can be like: def test_sum(): #Testing assert actual_result_ototal_order_dict['1'] == 91, "The actual result is not the \ same as expected result for the…arrow_forwardWhich of the following statements DOES NOT delete the last value in the list lst? a) 1st.pop( b) 1st.remove(-1) c) 1st = lst[:-1] d) 1st.pop(len(lst)-1)arrow_forward
- Word Puzzle GameIn this assignment is only for individual. You are going to decode the scrambled word into correct orderin this game. One round of play is as follows:1. Computer reads a text file named words.txt2. Computer randomly picks one word in the file and randomly scrambles characters in the wordmany times to hide the word3. Computer displays the scrambled word to user with indexes and gives the user options to1. Swap two letters in word based on index given2. Solve the puzzle directly3. Quit the game4. If a player chooses to swap letters, the computer reads two indexes and swaps the letters. Thegame resume to step 3 with the newly guessed word. If the resulted word after swapping is thesecret word, the game is over.5. If a user chooses to solve directly, computer prompts the user to enter the guessed word. If it’scorrect, the game is over, otherwise goes to step 3.6. After game is over, display how many times the player has tried to solve the puzzle.The following is sample…arrow_forwardCreates and returns a mutable dictionary, initially giving it enough allocated memory to hold a given number ofentries.arrow_forwardThis is question 5 on the same chapter 9arrow_forward
- Python question please include all steps and screenshot of code. Also please provide a docstring, and comments throughout the code, and test the given examples below. Thanks. Write a function called honorRoll(). Its only input parameter is a list that contains listsconsisting of a name followed by a series of grades (integers). All grades will be in therange 0-100. All students will have at least one grade, but not all students will have thesame number of grades. Your function should return a list of students whose averagegrade is above 92.0.>>> honorRoll([['Alice', 95, 92, 98], ['Carlos', 85, 87, 95, 91], ['Betty', 81, 74, 89]])['Alice']>>> honorRoll([['Alice', 92, 92, 92], ['Bob', 81, 46]])[]arrow_forwardMake new code pleasearrow_forwardWrite a prgram that creates a dictionary containing course numbers and room numbers of the room where the courses meet. The dictionary should have the following key value pairs: Course Number Room Number CS101 3004 CS102 4501 CS103 6755 NT110 1244 CM241 1411 This program should also create a dictionary containing course numbers and the names of the instructors that teach each course. The dictionary should have the following key-value pairs: Course Number Instructor CS101 Haynes CS102 Alvarado CS103 Rich NT110 Burke CM241 Lee This program should also create a dictionary containing the course numbers and the meeting times of each course. The dictionary should have the following key values Course number Meeting Time CS101 8:00 a.m. CS102 9:00 a.m. CS103 10:00 a.m. NT110 11:00 a.m. CM241 1:00 p.m. If…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
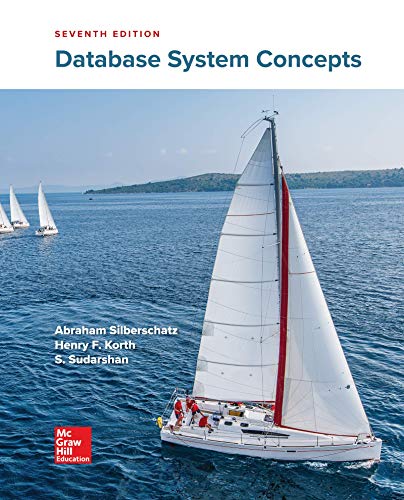
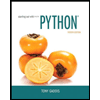
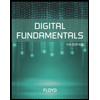
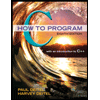
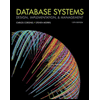
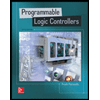