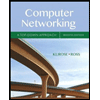
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
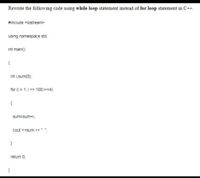
Transcribed Image Text:Rewrite the following code using while loop statement instead of for loop statement in C++.
#include <iostream>
using namespace std;
int main()
{
int i,sum(0);
for (i = 1; i <= 100;i+=4)
{
sum=sum+i;
cout <<sum <<"
}
retum 0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Similar questions
- Overview In this assignment, the student will write a C++ program that identifies a non-negative integer as prime, composite, or neither and provides the unique prime factorization for the composite case. When completing this assignment, the student should demonstrate mastery of the following concepts: · Decision Making (if, else) · Iteration (for, while) · Number Theory (Prime Factors) · Advanced Loop Variants Assignment Write a C++ program that prompts the user for a single integer value. After receiving the value, perform an analysis to determine if the integer is prime, composite or neither. If you are not sure what this means, you need to research the appropriate topics in number theory and consider what the algorithmic approach to determining if these properties are present would be from a programming perspective. In the event that you determine the provided integer is composite, provide a listing of the unique prime factorization for that integer as illustrated in the…arrow_forwardASSIGNMENT: Write a program to use the capability of Recursion to calculate factorials. For example, 5 factorial is normally written as 5! 5! = 5*4*3*2*1 5! = 120 Use recursive function calling to multiply. 5*4*3*2*1 And then print the result. 120 Your output should resemble the image below. >sh -c jav d. -type f > java -cla 5 4 2 5! = 120 } Note: 5! is use in this example but your program should calculate the factorial for any number entered.arrow_forwardC++ Programming 1arrow_forward
- C++ This is what i have so far. Can you use a for loop for the code. #include <iostream> // for cin and cout#include <iomanip> // for setw() and setfill()using namespace std; // so that we don't need to preface every cin and cout with std:: int main(){int menuOption = 0; cout << "Choose from among the following options:\n"<< "1. Exit the program\n"<< "2. Display building\n"<< "Your choice -> ";cin >> menuOption;cout << endl; // Leave a blank line after getting the user input for the menu option. // See if exit was chosenif (menuOption == 1) {exit(0);} // Menu 2 if (menuOption == 2){cout << " /\\ " << endl;cout << " || " << endl;cout << " || " << endl;cout << " -- " << endl;cout << "|++|" << endl;cout << "====" << endl; } cout << endl;return 0;}arrow_forwardThis is the C code I have so far #include <stdio.h> #include <stdlib.h> struct employees { char name[20]; int ssn[9]; int yearBorn, salary; }; struct employees **emps = new employees()[10]; //Added new statement ---- bartleby // function to read the employee data from the user void readEmployee(struct employees *emp) { printf("Enter name: "); gets(emp->name); printf("Enter ssn: "); for(int i =0; i <9; i++) scanf("%d", &emp->ssn[i]); printf("Enter birth year: "); scanf("%d", &emp->yearBorn); printf("Enter salary: "); scanf("%d", &emp->salary); } // function to create a pointer of employee type struct employees *createEmployee() { // creating the pointer struct employees *emp = malloc(sizeof(struct employees)); // function to read the data readEmployee(emp); // returning the data return emp; } // function to print the employee data to console void display(struct employees…arrow_forward>> IN C PROGRAMMING LANGUAGE ONLY << COPY OF DEFAULT CODE, ADD SOLUTION INTO CODE IN C #include <stdio.h>#include <stdlib.h>#include <string.h> #include "GVDie.h" int RollSpecificNumber(GVDie die, int num, int goal) {/* Type your code here. */} int main() {GVDie die = InitGVDie(); // Create a GVDie variabledie = SetSeed(15, die); // Set the GVDie variable with seed value 15int num;int goal;int rolls; scanf("%d", &num);scanf("%d", &goal);rolls = RollSpecificNumber(die, num, goal); // Should return the number of rolls to reach total.printf("It took %d rolls to get a \"%d\" %d times.\n", rolls, num, goal); return 0;}arrow_forward
- C++ complete magic Square #include <iostream> using namespace std; /*int f( int x, int y, int* p, int* q ){if ( y == 0 ){p = 0, q = 0;return 404; // status: Error 404}*p = x * y; // product*q = x / y; // quotient return 200; // status: OK 200} int main(){int p, q;int status = f(10, 2, &p, &q);if ( status == 404 ){cout << "[ERR] / by zero!" << endl;return 0;}cout << p << endl;cout << q << endl; return 0;}*/ /*struct F_Return{int p;int q;int status;}; F_Return f( int x, int y ){F_Return r;if ( y == 0 ){r.p = 0, r.q = 0;r.status = 404;return r;}r.p = x * y;r.q = x / y;r.status = 200;return r;} int main(){F_Return r = f(10, 0);if ( r.status == 404 ){cout << "[ERR] / by zero" << endl;return 0;}cout << r.p << endl;cout << r.q << endl;return 0;}*/ int sumByRow(int *m, int nrow, int ncol, int row){ int total = 0;for ( int j = 0; j < ncol; j++ ){total += m[row * ncol + j]; //m[row][j];}return total; } /*…arrow_forwardC++ languagearrow_forwardInstructions for both assignments can be found in the picture linked to this post. The programs must be written in c++ and requires that the random number generator be seeded like this program: Program 3.25 // This program demonstrates random numbers. #include <iostream> #include <cstdlib> // For rand and srand #include <ctime> // For the time function using namespace std; int main() { // Get the system time. unsigned seed = time(0); // Seed the random number generator. srand(seed); // Display three random numbers. cout << rand() << endl; cout << rand() << endl; cout << rand() << endl; return 0; }arrow_forward
- Instructions for both assignments can be found in the picture linked to this post. The programs must be written in c++ and requires that the random number generator be seeded like this program: Program 3.25 // This program demonstrates random numbers. #include <iostream> #include <cstdlib> // For rand and srand #include <ctime> // For the time function using namespace std; int main() { // Get the system time. unsigned seed = time(0); // Seed the random number generator. srand(seed); // Display three random numbers. cout << rand() << endl; cout << rand() << endl; cout << rand() << endl; return 0; }arrow_forwardThis is the C code I have so far #include <stdio.h> #include <stdlib.h> struct employees { char name[20]; int ssn[9]; int yearBorn, salary; }; struct employees **emps = new employees()[10]; //Added new statement ---- bartleby // function to read the employee data from the user void readEmployee(struct employees *emp) { printf("Enter name: "); gets(emp->name); printf("Enter ssn: "); for(int i =0; i <9; i++) scanf("%d", &emp->ssn[i]); printf("Enter birth year: "); scanf("%d", &emp->yearBorn); printf("Enter salary: "); scanf("%d", &emp->salary); } // function to create a pointer of employee type struct employees *createEmployee() { // creating the pointer struct employees *emp = malloc(sizeof(struct employees)); // function to read the data readEmployee(emp); // returning the data return emp; } // function to print the employee data to console void display(struct employees…arrow_forwardLook at the following C++ code and comment each line about how it works with pointer. int i = 33; double d = 12.88; int * iPtr = &i; double * dPtr = &d; // iPtr = &d; // dPtr = &i; // iPtr = i; // int j = 99; iPtr = &j; //arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
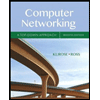
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
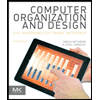
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
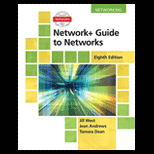
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
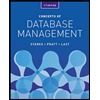
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
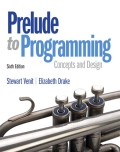
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
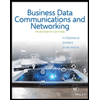
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY