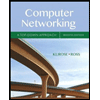
Stack:#ifndef STACKTYPE_H_INCLUDED
#define STACKTYPE_H_INCLUDED
const int MAX_ITEMS = 5;
class FullStack
// Exception class thrown
// by Push when stack is full.
{};
class EmptyStack
// Exception class thrown
// by Pop and Top when stack is emtpy.
{};
template <class ItemType>
class StackType
{
public:
StackType();
bool IsFull();
bool IsEmpty();
void Push(ItemType);
void Pop();
ItemType Top();
private:
int top;
ItemType items[MAX_ITEMS];
};
#endif // STACKTYPE_H_INCLUDED
Queue:#ifndef QUETYPE_H_INCLUDED
#define QUETYPE_H_INCLUDED
template<class T>
class QueType
{
public:
QueType();
QueType(int);
~QueType();
void MakeEmpty();
bool IsEmpty();
bool IsFull();
void Enqueue(T);
void Dequeue(T&);
T Front();
private:
int front;
int rear;
T *info;
int maxQue;
};
#endif // QUETYPE_H_INCLUDED
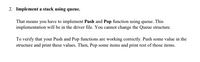

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Assume class StackType has been defined to implement a stack data structure as discussed in your textbook (you may also use the stack data structure defined in the C++ STL). Write a function that takes a string parameter and determines whether the string contains balanced parentheses. That is, for each left parenthesis (if there is any) there is exactly one matching right parenthesis later in the string and every right parenthesis is preceded by exactly one matching left parenthesis earlier in the string. e.g. abc(c(x)d(e))jh is balanced whereas abc)cd(e)(jh is not. The function algorithm must use a stack data structure to determine whether the string parameter satisfies the condition described above. Edit Format Table 12pt v Paragraph v |BIU A v ev T? WP O words ....arrow_forward#include<bits/stdc++.h>using namespace std;#define MAX 1000 //Maximum size of stack class Stack{ int top;// to store top of stack public: int elements[MAX]; //Integer array to store elements Stack(){ //class constructor for initialization top=-1; } bool push(int x) { if(top>=(MAX-1)) //Condition for top when stack because full { cout<<"Stack is full\n"; return false; } else { ++top; //Increasing top value elements[top]=x; //storing it to element in to stack return true; }}int pop(){ if(top<0)// Condition for top when stack is empty { cout<<"stack is empty\n"; return INT_MIN; } else { int x=elements[top--];//poping out the element for stack by decreasing to element return x; }}int display(){if(top<0)//Condition for top when stack is empty { cout<<"Stack is…arrow_forwardC programming fill in the following code #include "graph.h" #include <stdio.h>#include <stdlib.h> /* initialise an empty graph *//* return pointer to initialised graph */Graph *init_graph(void){} /* release memory for graph */void free_graph(Graph *graph){} /* initialise a vertex *//* return pointer to initialised vertex */Vertex *init_vertex(int id){} /* release memory for initialised vertex */void free_vertex(Vertex *vertex){} /* initialise an edge. *//* return pointer to initialised edge. */Edge *init_edge(void){} /* release memory for initialised edge. */void free_edge(Edge *edge){} /* remove all edges from vertex with id from to vertex with id to from graph. */void remove_edge(Graph *graph, int from, int to){} /* remove all edges from vertex with specified id. */void remove_edges(Graph *graph, int id){} /* output all vertices and edges in graph. *//* each vertex in the graphs should be printed on a new line *//* each vertex should be printed in the following format:…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
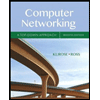
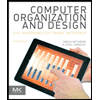
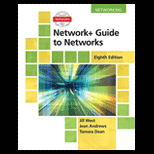
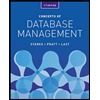
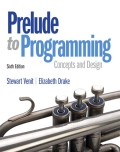
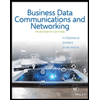