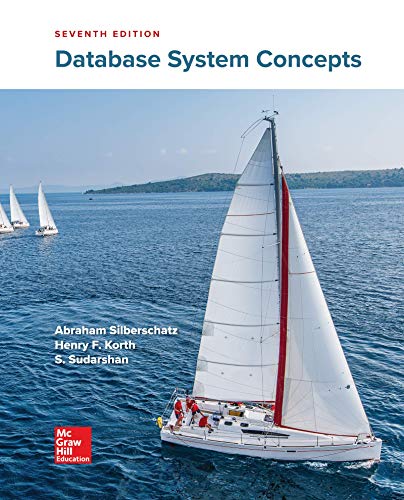
Structure chart with parameter passing for the following program
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
//structure
typedef struct
{
char Username[20];
int cents;
}value;
int *coinChange(int change);
//main function
int main(){
FILE *f;
//open the file
f = fopen("coins.txt", "r");
//check if file not opened
if(f==NULL)
{
printf("File not opened!");
return 1;
}
value arr[10];
int i, j, n, option, totalCoins;
char name[20];
int *coins; // =
//read the file
for(i=0; !feof(f) ; i++)
{
fscanf(f, "%s", arr[i].Username);
fscanf(f, "%d", &arr[i].cents);
}
n = i;
//close the file
fclose(f);
//loop
while(1)
{
//display menu
printf("1. Enter name\n");
printf("2. Exit\n\n");
//prompt and read option
printf("Enter option (1 or 2): ");
scanf("%d", &option);
//switch statement
switch(option)
{
case 1:
//read the name
scanf("%s", name);
totalCoins = 0;
//search in the list
for(i=0; i<n; i++)
{
if(!strcmp(name, arr[i].Username))
{
totalCoins = totalCoins + arr[i].cents;
}
}
//check if not found
if(totalCoins==0)
printf("Not found\n\n");
else
{
//get the change
coins = coinChange(totalCoins);
//print the change
if(coins[0])
printf("50 cents: %d\n", coins[0]);
if(coins[1])
printf("20 cents: %d\n", coins[1]);
if(coins[2])
printf("10 cents: %d\n", coins[2]);
if(coins[3])
printf("5 cents: %d\n", coins[3]);
printf("\n\n");
}
break;
case 2:
//create csv file
f = fopen("change.csv", "w");
//search the name in the list
for(i=0; i<n-1; i++)
{
totalCoins = arr[i].cents;
strcpy(name, arr[i].Username);
for(j=i+1; j<n; j++)
{
if(!strcmp(name, arr[j].Username))
{
totalCoins = totalCoins + arr[j].cents;
arr[j].cents = 0;
}
}
//check if found
if(totalCoins!=0)
{
//get change
coins = coinChange(totalCoins);
//print to the csv file
fprintf(f, "%s,%d,%d,%d,%d,%d\n", arr[i].Username, totalCoins, coins[0],
coins[1],coins[2],coins[3]);
}
}
//close the file
fclose(f);
return 0;
default:
printf("Wrong option. Try again\n\n");
}
}
return(0);
}
//function to calculate the change
int* coinChange(int change){
int *coins = (int*)calloc(4, sizeof(int));
while(change > 0){
if(change > 0 && change <=95 && change% 5 == 0){
if(change >= 50){
change -= 50;
coins[0]++;
}else if(change >= 20){
change -= 20;
coins[1]++;
}else if(change>=10){
change -= 10;
coins[2]++;
}else if(change>=5){
change -= 5;
coins[3]++;
}
}
}
return coins;
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- #include #include #include "Product.h" using namespace std; int main() { vector productList; Product currProduct; int currPrice; string currName; unsigned int i; Product resultProduct; cin>> currPrice; while (currPrice > 0) { } cin>> currPrice; main.cpp cin>> currName; currProduct.SetPriceAndName (currPrice, currName); productList.push_back(currProduct); resultProduct = productList.at (0); for (i = 0; i < productList.size(); ++i) { Type the program's output Product.h 1 CSE Scanned Product.cpp if (productList.at (i).GetPrice () < resultProduct.GetPrice ()) { resultProduct = productList.at(i); } AM cout << "$" << resultProduct.GetPrice() << " " << resultProduct. GetName() << endl; return 0; Input 10 Cheese 6 Foil 7 Socks -1 Outputarrow_forwardWrite a function getNeighbors which will accept an integer array, size of the array and an index as parameters. This function will return a new array of size 2 which stores the neighbors of the value at index in the original array. If this function would result in returning garbage values the new array should be set to values {0,0} instead of values from the array.arrow_forward#include <iostream> #include <iomanip> #include <string> using namespace std; struct Teletype { string name; string phonenum; Teletype *nextaddr; }; void populate(Teletype *); void displayrecord(Teletype *); //void insertrecord(Teletype *); // create //void removerecord(Teletype *); //create //void modifyrecord(Teletype *); // create //int find(TeleType *, string); // Extra Credit create bool check(); int main() { int location = 0; int count = 0; char answery_n; Teletype *list, *current; list = new Teletype; current = list; cout << "Please "; do { count++; populate(current); if (check() == false) { cout << " Not storage available" << endl; } else { current->nextaddr = new Teletype; current = current->nextaddr;…arrow_forward
- Background Information This assignment tests your understanding of and ability to apply the programming concepts we have covered in the unit so far, including the usage of variables, input and output, data types, selection, iteration, functions and data structures. Above all else, it tests your ability to design and then implement a solution to a problem using these concepts. Assignment Overview You are required to design and implement a "Word Game" program in which the user must identify a randomly selected "password" from a list of 8 words. The 8 words are selected at random from the list of 100 words in the starter file (word_game.py) provided to you with this assignment brief. Please use the starter file as the basis of your assignment code. The user has 4 attempts in which to guess the password. Whenever they guess incorrectly, they are told how many of the letters are the same between the word they guessed and the password. For example, if the password is "COMEDY" and…arrow_forwardThis is the C code I have so far #include <stdio.h> #include <stdlib.h> struct employees { char name[20]; int ssn[9]; int yearBorn, salary; }; struct employees **emps = new employees()[10]; //Added new statement ---- bartleby // function to read the employee data from the user void readEmployee(struct employees *emp) { printf("Enter name: "); gets(emp->name); printf("Enter ssn: "); for(int i =0; i <9; i++) scanf("%d", &emp->ssn[i]); printf("Enter birth year: "); scanf("%d", &emp->yearBorn); printf("Enter salary: "); scanf("%d", &emp->salary); } // function to create a pointer of employee type struct employees *createEmployee() { // creating the pointer struct employees *emp = malloc(sizeof(struct employees)); // function to read the data readEmployee(emp); // returning the data return emp; } // function to print the employee data to console void display(struct employees…arrow_forwardIn C++Using the code provided belowDo the Following: Modify the Insert Tool Function to ask the user if they want to expand the tool holder to accommodate additional tools Add the code to the insert tool function to increase the capacity of the toolbox (Dynamic Array) USE THE FOLLOWING CODE and MODIFY IT: #define _SECURE_SCL_DEPRECATE 0 #include <iostream> #include <string> #include <cstdlib> using namespace std; class GChar { public: static const int DEFAULT_CAPACITY = 5; //constructor GChar(string name = "john", int capacity = DEFAULT_CAPACITY); //copy constructor GChar(const GChar& source); //Overload Assignment GChar& operator=(const GChar& source); //Destructor ~GChar(); //Insert a New Tool void insert(const std::string& toolName); private: //data members string name; int capacity; int used; string* toolHolder; }; //constructor GChar::GChar(string n, int cap) { name = n; capacity = cap; used = 0; toolHolder = new…arrow_forward
- Create pseudocode for the following #include <iostream> #include <string> #include <cstdlib> #include <ctime> using namespace std; char water[10][10] = {{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'},{'~','~','~','~','~','~','~','~','~','~'}}; void createBoard(int &numShip); void promptCoords(int& userX, int &userY); void shipGen(int shipX[] ,int shipY[], int &numShip); void testCoords(int &userX, int &userY, int shipX[], int shipY[], int &numShip, int& victory); void updateBoard();arrow_forward>> IN C PROGRAMMING LANGUAGE ONLY << COPY OF DEFAULT CODE, ADD SOLUTION INTO CODE IN C #include <stdio.h>#include <stdlib.h>#include <string.h> #include "GVDie.h" int RollSpecificNumber(GVDie die, int num, int goal) {/* Type your code here. */} int main() {GVDie die = InitGVDie(); // Create a GVDie variabledie = SetSeed(15, die); // Set the GVDie variable with seed value 15int num;int goal;int rolls; scanf("%d", &num);scanf("%d", &goal);rolls = RollSpecificNumber(die, num, goal); // Should return the number of rolls to reach total.printf("It took %d rolls to get a \"%d\" %d times.\n", rolls, num, goal); return 0;}arrow_forwardAlgorithm for the below program #include<stdio.h>#include<stdlib.h>#include<string.h> //structuretypedef struct{char Username [20];int cents;} value; int *coin Change (int change); //main functionint main () { FILE *f; //open the filef = f open ("coins.txt", "r"); //check if file not openedif(f==NULL){printf ("File not opened!");return 1;} value arr [10];int i, j, n, option, totalCoins;char name [20];int *coins; // = //read the filefor (i=0;!feof(f) ; i++){fscanf(f, "%s", arr[i].Username);fscanf(f, "%d", &arr[i].cents);} n = i; //close the filefclose(f); //loopwhile (1){//display menuprintf ("1. Enter name\n");printf ("2. Exit\n\n"); //prompt and read optionprintf ("Enter option (1 or 2): ");scanf ("%d", &option); //switch statementswitch(option){case 1://read the namescanf ("%s", name);totalCoins = 0;//search in the listfor(i=0; i<n; i++){if(!strcmp(name, arr[i].Username)){totalCoins = totalCoins + arr[i].cents;}}//check if not…arrow_forward
- C++ This program does not compile! Spot the error and give the line number. Presume that the header file is error free and // Implementation file for the Rectangle class.1. #include "Rectangle.h" // Needed for the Rectangle class2. #include <iostream> // Needed for cout3. #include <cstdlib> // Needed for the exit function4. using namespace std; //***********************************************************// setWidth sets the value of the member variable width. *//*********************************************************** 5. void Rectangle::setWidth(double w){6. if (w >= 0)7. width = w;8. else{9. cout << "Invalid width\n";10. exit(EXIT_FAILURE);}} //***********************************************************// setLength sets the value of the member variable length. *//*********************************************************** 11. void Rectangle::setLength(double len){12. if (len >= 0)13. length = len;14. else{15. cout << "Invalid length\n";16.…arrow_forwardIn C++ struct myGrades { string class; char grade; }; Declare myGrades as an array that can hold 5 sets of data and then set a class string in each position as follows: “Math” “Computers” “Science” “English” “History” and give each class a letter gradearrow_forward/* Programming concept: structures Program should: be a C program, define a structure to store the year, month, day, and high temperature. write a function get_data that has a parameter that is a pointer to a struct and reads from the user into the struct pointed to by the parameter. write a function print_data that takes the struct pointer as a parameter and prints the data. in main, declare a struct, call the get_data function, call the print_data function. */ #include <stdio.h> int main(int argc, char *argv[]) { return 0;}arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
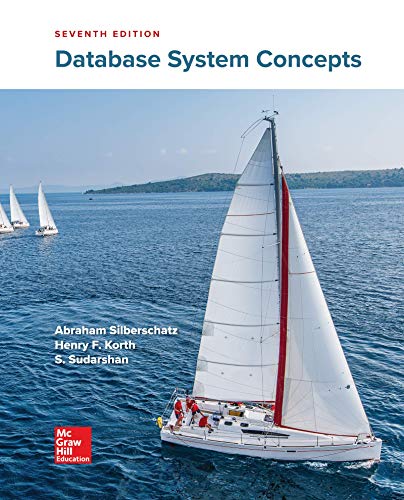
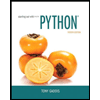
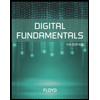
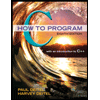
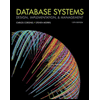
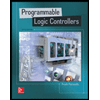