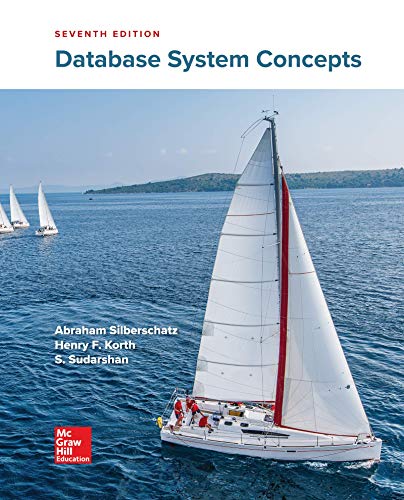
Task - Using pointers to process arrays (C Language)
Example #5 below expected output is 45, but from the
In a TV show, each minute can be either interesting or boring. Assume that if 7 consecutive minutes are boring, then an average viewer will stop watching the show. Write a C program that calculates how many minutes that an average viewer will watch a TV show, given the interesting minutes. Assume the TV shows are 45 minutes long.
Requirements
- Name your program project4_minutes.c.
- Follow the format of the examples below.
- The program will read in the number of interesting minutes, then read in the interesting minutes.
- The program should include the following function. Do not modify the function prototype.
int find_minute(int *minutes, int n);
-
- minutes represents the input array for interesting minutes, n is the length of the array (the number of interesting minutes). The function returns the how many minutes an average viewer will watch the show.
- This function should use pointer arithmetic– not subscripting – to visit array elements. In other words, eliminate the loop index variables and all use of the [] operator in the function.
- In the main function, call the find_minute function and display the result.
- Pointer arithmetic is NOT required in the main function.
Examples (your program must follow this format precisely)
Example #1
Enter the number of interesting minutes: 3
Enter the interesting minutes: 7 13 28
Output: 20
Note: In the first example, minutes 14, 15, 16, 17, 18, 19, 20 are all boring and thus an average viewer will stop watching after the 20th minute. So, the average viewer will watch for 20 minutes.
Example #2
Enter the number of interesting minutes: 3
Enter the interesting minutes: 7 13 18
Output: 25
Note: In the second example, minutes 19, 20, 21, 22, 23, 24, 25 are all boring and thus an average viewer will stop watching after the 25th minute. So, the average viewer will watch for 25 minutes.
Example #3
Enter the number of interesting minutes: 6
Enter the interesting minutes: 9 13 28 32 38 42
Output: 7
Note: In the third example, minutes 1, 2, 3, 4, 5, 6, 7 are all boring and thus an average viewer will stop watching after the 7th minute. So, the average viewer will watch for 7 minutes.
Example #4
Enter the number of interesting minutes: 6
Enter the interesting minutes: 5 12 18 24 31 38
Output: 45
Note: In the fourth example, there are no consecutive 7 boring minutes So, the average viewer will watch for the whole 45 minutes.
Example #5
Enter the number of interesting minutes: 8
Enter the interesting minutes: 2 9 13 20 25 29 33 39
Output: 45
Program-
#include <stdio.h>
//Function prototype
int find_minute(int *minutes, int n);
int main(){
int minutes[100];
int n;
//Ask user to enter the value
printf("Enter the number of interesting minutes: ");
scanf("%d", &n);
printf("Enter the interesting minutes: ");
//Read the interesting minutes
for(int i = 0; i < n; i++){
scanf("%d", &minutes[i]);
}
//Call the function
int result = find_minute(minutes, n);
//Print the result
printf("Output: %d\n", result);
return 0;
}
//Function definition
int find_minute(int *minutes, int n){
//start of the tv show at 0
int currTime = 0;
//Iterating using pointer arithmetic
// minutes+i is equivalent to &minutes[i]
for(int i = 0; i < n; i++){
if(*(minutes+i) <= currTime+7){
currTime = *(minutes+i);
}
else{
break;
}
}
int result;
if(currTime < 45){
result = currTime + 7;
}
else{
//Show stops at 45 minutes, result should not be greater than 45
result = 45;
}
return result;
}

Introduction
The given question is asking for a program that calculates the maximum number of minutes an average viewer will watch a TV show given the number of interesting minutes. The program assumes that if there are 7 consecutive minutes of boring content, then the average viewer will stop watching the show. The user is prompted to enter the number of interesting minutes, followed by the interesting minutes themselves.
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 4 images

- python only** define the following function: This function must set the value of a task in a checklist to True. It will accept two parameters: the checklist object to add to, and the name of the task to mark as completed. In addition to changing the task value, it must return the (now modified) checklist object that it was given. if the task name provided is not in the checklist, this function must print a specific message and must return None. Define completeTask with 2 parameters Use def to define completeTask with 2 parameters Use a return statement Within the definition of completeTask with 2 parameters, use return _ in at least one place. Do not use any kind of loop Within the definition of completeTask with 2 parameters, do not use any kind of loop.arrow_forwardData Structures the hasBalancedParentheses () method. Note: this can be done both iteratively or recursively, but I believe most people will find the iterative version much easier. C++: We consider the empty string to have balanced parentheses, as there is no imbalance. Your program should accept as input a single string containing only the characters ) and (, and output a single line stating true or false. The functionality for reading and printing answers is written in the file parentheses.checker.cpp; your task is to complete the has balanced.parentheses () function. **Hint: There's a pattern for how to determine if parentheses are balanced. Try to see if you can find that pattern first before coding this method up.arrow_forwardTrying to write a gravity function in C languagearrow_forward
- C PROGRAM Strictly use the following structures and function (arrays and strings)arrow_forwardC++ Code: This function will print out the information that has been previously read (using the function readData) in a format that aligns an individual's STR counts along a column. For example, the output for the above input will be: name Alice Bob Charlie ---------------------------------------- AGAT 5 3 6 AATG 2 7 1 TATC 8 4 5 This output uses text manipulators to left-align each name and counts within 10 characters. The row of dashes is set to 40 characters. /** * Computes the longest consecutive occurrences of several STRs in a DNA sequence * * @param sequence a DNA sequence of an individual * @param nameSTRs the STRs (eg. AGAT, AATG, TATC) * @returns the count of the longest consecutive occurrences of each STR in nameSTRs **/ vector<int> getSTRcounts(string& sequence, vector<string>& nameSTRs) For example, if the sequence is AACCCTGCGCGCGCGCGATCTATCTATCTATCTATCCAGCATTAGCTAGCATCAAGATAGATAGATGAATTTCGAAATGAATGAATGAATGAATGAATGAATG and the vector namesSTRs is…arrow_forwardMemory Management Programming Assignment Please if this can be coded in Java or C++ i would appreciate implement and test the GET-MEMORY algorithm This algorithm uses the Next-Fit(First-Fit-With-A-Roving-Pointer) technique. implement and test the FREE-MOMORY algorithm Implement the “GET_MEMORY” and “FREE_MEMORY” algorithms. Comprehensive testing must be done for each algorithm. Following are sample run results for each: GET_MEMORY IS RUNNING……… Initial FSB list FSB# Location Size 1 7 4 2 14 10 3 30 20 . . . . . . Rover is 14 ---------------------------------------------------------------------------- Allocation request for 5 words Allocation was successful Allocation was in location 14 FSB# Location Size 1 7 4 2 19 5 3 30 20 . . . . . . Rover is 30 ---------------------------------------------------------------------------- Allocation request for 150 words Allocation was not successful . . . __________________________________________________________ FREE_MEMORY…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
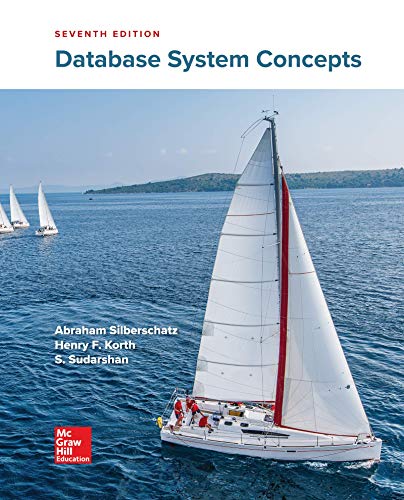
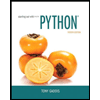
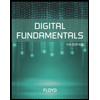
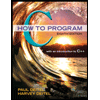
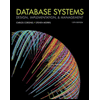
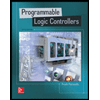