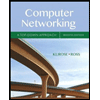
The following java code has many errors fix them so that the gui will work and output a new window with a slider!
private static void buildGui() {
JFrame frame = new JFrame();
JTextArea textArea = new JTextArea(5, 20);
// set default font size to 10 to match JSlider starting position
textArea.setFont(new Font(textArea.getFont().getName(), textArea.getFont().getStyle(), 10));
frame.add(textArea, BorderLayout.CENTER);
// set up slider
JSlider slider = new JSlider(JSlider.HORIZONTAL, 10, 30, 10);
slider.setMajorTickSpacing(5);
slider.setMinorTickSpacing(1);
slider.setPaintTicks(true);
slider.setPaintLabels(true);
frame.add(slider, BorderLayout.SOUTH);
// create and add change listener
slider.addChangeListener(new ChangeListener() {
@Override
public void stateChanged(ChangeEvent e) {
int fontSize = slider.getValue();
Font currentFont = textArea.getFont();
// get the current font and set the modified font size
textArea.setFont(new Font(currentFont.getName(), currentFont.getStyle(), fontSize));
}
});
frame.pack();
frame.setVisible(true);
}

1. Create a class give any name( I named Main)
2. Import the awt and swing and event.
3. put all the code in class
4. call the build gui method in main.
5. Done
Step by stepSolved in 4 steps with 2 images

- Write the application JFrameDisableButton that instantiates a JFrame that contains a JButton. Modify the JFrameDisableButton program so that the JButton is not disabled until the user has clicked at least eight times. At that point, display a JLabel that indicates “That’s enough!”. import java.awt.*; import javax.swing.*; import java.awt.event.*; public class JFrameDisableButton extends JFrame implements ActionListener { final int SIZE = 180; Container con = getContentPane(); JButton button = new JButton("Press Me"); int count = 0; final int MAX = 8; JLabel label = new JLabel("That's enough!"); public JFrameDisableButton() { // Write your code here } @Override public void actionPerformed(ActionEvent e) { // Write your code here } public static void main(String[] args) { JFrameDisableButton frame = new JFrameDisableButton(); } }arrow_forwardWrite JavaFX code with an intuitive GUI design that can generate a Fibonacci number sequence of a certain length (e.g., 30-50 numbers) via pressing a ‘Fib Gen’ button. The program should have at least two filtering functions on the generated number sequence.arrow_forwardPlease rewrite all of this animation code so that it performs the same functions in a java file instead of the files .css,.js, and .html (which is what I have) in visual studios. You would need to import javaFX since this would require GUI. My styles.css and Animation.html are attached as screenshots, and my script.js is below: script.js const dialogue = [ { character: 'Narrator', text: "In the dead of night, beneath a moon veiled by misty clouds, a lone figure trudged along a desolate forest path." }, { character: 'Narrator', text: "Unknown to her, she was not alone." }, { character: 'Human', text: "Who's there? Show yourself!" }, { character: 'Creature', text: "You venture into realms unknown, mortal. What brings you to these woods?" }, { character: 'Human', text: "I seek passage to the village beyond. I mean no harm." }, { character: 'Creature', text: "Harm is but a shadow in these woods, mortal." }, { character: 'Human', text: "What do you mean? Who are…arrow_forward
- can someone help me resize the picture in Java. This is my code: import java.awt.*;import java.awt.image.BufferedImage;import java.io.File;import java.io.IOException;import javax.imageio.ImageIO; class ImageDisplayGUI { public static void main(String[] args) { SwingUtilities.invokeLater(() -> { createAndShowGUI(); }); } private static void createAndShowGUI() { JFrame frame = new JFrame("Bears"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(800, 600); frame.setLayout(new BorderLayout()); // Load and display the JPG image try { BufferedImage image = ImageIO.read(new File("two bears.png")); // Replace with your image path ImageIcon icon = new ImageIcon(image); JLabel imageLabel = new JLabel(icon); frame.add(imageLabel, BorderLayout.CENTER); } catch (IOException e) { // Handle image loading error…arrow_forwardx = zeros(uint8(5,5)); for i = 1:5 for j = 1:5 a = 5/i; x(i,j) = 255/a; end end Which of the following best describes the black and white image x? 1. The columns of x will get lighter from left to right 2. The rows of x will get lighter from top to bottom 3. The rows of x will get darker from top to bottom 4. The columns of x will get darker from left to rightarrow_forwardUse a Bootstrap class to add rounded corners on the image. <img src="img_chania.jpg" alt="Chania" class=" ">arrow_forward
- Write a main program that displays a single frame with the title “My First Frame”. Set the size to 800 by 800. Make the frame visible. Create a panel by using the JPanel constructor and add it to the frame. Use Color.RED (a constant in the java.awt package) along with the setBackground() method in JPanel, to set the color of the panel. Add a JButton and a JLabel to the panel before adding the panel to the frame. Display the resultsarrow_forwardSave the Word document and close them. Go to D2L and submit it by attaching the document file.You mayarrow_forwardPlease help me fix the errors in the java program below. There are two class AnimatedBall and BouncingBall import javax.swing.*;import java.awt.*;import java.awt.event.ActionEvent;import java.awt.event.ActionListener;public class AnimatedBall extends JFrame implements ActionListener{private Button btnBounce; // declare a button to playprivate TextField txtSpeed; // declare a text field to enterprivate Label lblDelay;private Panel controls; // generic panel for controlsprivate BouncingBall display; // drawing panel for ballContainer frame;public AnimatedBall (){// Set up the controls on the appletframe = getContentPane();btnBounce = new Button ("Bounce Ball");//create the objectstxtSpeed = new TextField("10000", 10);lblDelay = new Label("Enter Delay");display = new BouncingBall();//create the panel objectscontrols = new Panel();setLayout(new BorderLayout()); // set the frame layoutcontrols.add (btnBounce); // add controls to panelcontrols.add (lblDelay);controls.add…arrow_forward
- def edge_highlight(self, image): """ Returns a new image with the edges highlighted # Check output >>> img_proc = PremiumImageProcessing() >>> img = img_read_helper('img/test_image_32x32.png') >>> img_edge = img_proc.edge_highlight(img) >>> img_exp = img_read_helper('img/exp/test_image_32x32_edge.png') >>> img_exp.pixels == img_edge.pixels # Check edge_highlight output True >>> img_save_helper('img/out/test_image_32x32_edge.png', img_edge) """ # YOUR CODE GOES HERE # kernel = [[-1, -2, -1], [0, 0, 0], [1, 2, 1] ] pixels = image.pixels new_pixels = [] for y in range(len(pixels)): new_row = [] for x in range(len(pixels[y])): neighbors = [] for dy in range(-1, 2): for dx in range(-1, 2): nx = x + dx…arrow_forwardPlease rewrite this animation code below so that it performs the same functions in a java file instead of the files .css,.js, and .html in visual studios. You would need to import javaFX and include your images for creature.png,forestbackground.png,human.png, and human2.png. Also attach a screenshot of the running animation in java. script.js const dialogue = [ { character: 'Narrator', text: "In the dead of night, beneath a moon veiled by misty clouds, a lone figure trudged along a desolate forest path." }, { character: 'Narrator', text: "Unknown to her, she was not alone." }, { character: 'Human', text: "Who's there? Show yourself!" }, { character: 'Creature', text: "You venture into realms unknown, mortal. What brings you to these woods?" }, { character: 'Human', text: "I seek passage to the village beyond. I mean no harm." }, { character: 'Creature', text: "Harm is but a shadow in these woods, mortal." }, { character: 'Human', text: "What do you mean?…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
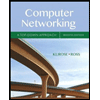
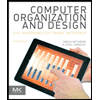
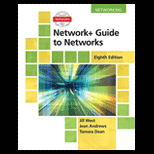
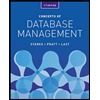
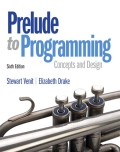
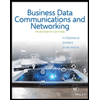