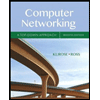
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
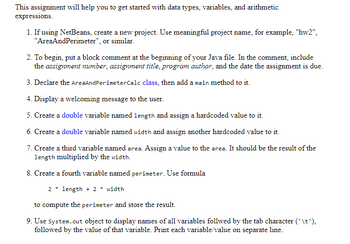
Transcribed Image Text:This assignment will help you to get started with data types, variables, and arithmetic
expressions.
1. If using NetBeans, create a new project. Use meaningful project name, for example, "hw2",
"AreaAndPerimeter", or similar.
2. To begin, put a block comment at the beginning of your Java file. In the comment, include
the assignment number, assignment title, program author, and the date the assignment is due.
3. Declare the AreaAnd PerimeterCalc class, then add a main method to it.
4. Display a welcoming message to the user.
5. Create a double variable named length and assign a hardcoded value to it.
6. Create a double variable named width and assign another hardcoded value to it.
7. Create a third variable named area. Assign a value to the area. It should be the result of the
length multiplied by the width.
8. Create a fourth variable named perimeter. Use formula
2* length + 2 * width
to compute the perimeter and store the result.
9. Use System.out object to display names of all variables follwed by the tab character ('\t').
followed by the value of that variable. Print each variable/value on separate line.
![Coding Requirements
• As mentioned above, program should contain a block comment with the description of what
it does.
• Label the end of your class and the end of the main method with appropriate "ending"
comments:
public
public class AreaAndPerimeterApp
{
public static void main (String[] args)
{
// The code here is indented between the braces.
// ...
}//main
}//class AreaAndPerimeter App
• Align each opening and closing brace { } vertically as shown, so that the code looks neat, is
readable, and easy to understand.
This is often called "the coding style" by professional programmers. Successful styles exist
for many popular programming environments, including the style presented in our textbook.
Once adopted, the style must be followed by all programmers throughout the entire duration
of the project.
Indent all code between the beginning and ending braces. Indentation of all code blocks
allow program statements and comments to be easily observed.](https://content.bartleby.com/qna-images/question/1c50bd72-23cb-4139-953a-0cc18f92fc0e/5e54b307-6eb0-4b6e-be7f-7eb83fcb2db5/v9nv7ii_thumbnail.png)
Transcribed Image Text:Coding Requirements
• As mentioned above, program should contain a block comment with the description of what
it does.
• Label the end of your class and the end of the main method with appropriate "ending"
comments:
public
public class AreaAndPerimeterApp
{
public static void main (String[] args)
{
// The code here is indented between the braces.
// ...
}//main
}//class AreaAndPerimeter App
• Align each opening and closing brace { } vertically as shown, so that the code looks neat, is
readable, and easy to understand.
This is often called "the coding style" by professional programmers. Successful styles exist
for many popular programming environments, including the style presented in our textbook.
Once adopted, the style must be followed by all programmers throughout the entire duration
of the project.
Indent all code between the beginning and ending braces. Indentation of all code blocks
allow program statements and comments to be easily observed.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Similar questions
- Instructions: For each Exercise below, write your code in an IDE and run your code within the IDE as well. Once you have satisfied the Exercise requirements, paste your code below under the corresponding Exercise. Exercise 1: The Hogwarts School of Witchcraft and Wizardry welcomes you! First-year students must go through the annual Sorting Ceremony. The Sorting Hat is a talking hat at Hogwarts that magically determines which of the four school Houses each new student belongs most to: Gryffindor ● Hufflepuff ● Ravenclaw ● Slytherin Your task is the following: Please Write a sortinghat.cpp program that asks the user some questions and places them into one of the four Houses based on their answers! ●arrow_forwardUsing Eclipse, create a New Java project named YourNameCh7Project-- for example, I would name my Project PaulaStrozierCh7Project Create a new class named YourInitialsFutureDaysApp--for example I would name my class PSFutureDaysApp Line 1 should have a comment with YourName Delete any unnecessary comments created by Eclipse. All variable names and array names must begin with your initials in lower case. Example: I would use my initials, psInput, psToday, pselapsedDays, psfutureDay, psDayNames Be sure to make comments throughout your project explaining your code. Write a program that prompts the user to enter an integer for today’s day of the week (Sunday is 0, Monday is 1, . . . , and Saturday is 6). Also prompt the user to enter the number of days after today for a future day and display the future day of the week. Use an array to store the names for days in a week.arrow_forwardThe Mid-Term Exam: A program to write (below). Note that you must submit and return directly to me with your name and the date included as an upload Canvas (file) submission. Make sure that your name is used as the file name. (You will not get credit if your exam cannot be read.) No notes, books, cells, Internet Search, computer/device, etc., may be used during this exam. Write a Java program that lets the user enter a series of integers with an input range from 2 to 199 (in random order). The user must enter a value of -1, 0, or +1 (a sentinel which must be an integer and not part of the series of numbers) in order to signal the end of the series. • After all the numbers have been entered, the program should display the smallest and largest numbers entered, and not include the sentinel. Note that this must be a Java implementatiọn, and not pseudo-code, etc. You must use a Loop and If-Else constructs as well.arrow_forward
- Imagine we were creating a Textbased Adventure program and our Rooms in the assignment above could hold Items. We are not writing the complete game, but lets write a program that will build the Item.txt file. Create a new project. Add a class called Item that stores information about an item that can be placed in a room. An item has a name, a description and the room number in which the item is initially is stored. LAMP, a brightly shining lamp, 3 is an example of data that an Item might contain. Using the UML model below, create the private data members and methods for constructing an item. Create an ItemFileWriter class with a static method to write the items from the given ArrayList to a text file.. The items text file will hold the item name, item description and the initial room that the item is to be loaded into, on seperate lines followed by a blank line. An example of the file that is to be constructed is attached. Construct a class with main() in it. Create an…arrow_forwardYour module should be named “loc.py”. Include instance variables for all data about a location (name, summary, details.) The constructor should allow creating a location with whatever name, summary, and details you want, but a newly create Locale has not yet been visited. The string representation of a Locale should be its summary if it has not yet been visited, otherwise it should be a brief message including its name, such as “You are at Sandy Beach.” Include other observer and mutator methods as needed.arrow_forwardin java but don't write as a GUI (Graphical User Interface) Write a program that reads a file named input.txt and writes a file that contains the same contents, but is named output.txt. The input file will contain more than one line when I test this and so should your output file. Do not use a path name when opening these files. This means the files should be located in the top level folder of the project. This would be the folder that contains the src folder, probably named FileCopy depending on what name you gave the project. Do not use a copy method that is supplied by Java. Your program must read the file line by line and write the file itself. Class should be named FileCopy do not write to input.txt!arrow_forward
- Create a new project named lab7_1. You will be implementing a Tacos class. For the class attributes, let’s use to type of taco, number of tacos, and price. Note that the price per taco of any type is 99 cents. Therefore, allow the taco type and number of tacos to be set directly, but not the price! The price should be set automatically based on the number of tacos entered. So a good idea may be to call the setter for the price inside the function that sets the number of tacos. Some other requirements: A default constructor and a constructor with parameters for your taco type and number of tacos member variables. You should also include setters and getters for these private member variables. However, make sure that the setter for price is private! You don’t want anyone outside the class modifying price. This setter should be called anytime you change the number of tacos. Your constructor needs to call a set method that allows you to set the two member variables, thus takes two…arrow_forwardQ2: Create a book modelDraw a class diagram to depict a book as specified by the following statement: "A book is made up of several parts, each of which is made up of several chapters."Sections are made up of chapters." To begin, concentrate just on classes and associations.Multiplicity should be added to the class diagram you created.Improve the class diagram by adding the following features:• The book has a publisher, a publishing date, and an ISBN number.• A title and a number are included in a part; a title, a number, and an abstract are included in a chapter; and a title, a number, and an abstract are included in a section.Take a look at the refined class diagram. A title and a number characteristic are included in the Part, Chapter, and Section classes. To factor out these two traits, use inheritance.arrow_forward*with psuedo code First, launch NetBeans and close any previous projects that may be open (at the top menu go to File ==> Close All Projects). Then create a new Java application called "WeightedAvgDataAnalyzer" (without the quotation marks), that does the following: This program is similar to the previous assignment - "WeightedAvgDropSmallest" - with a major difference that the inputs for the program will be read from a file and the output for this assignment should be written to a new file. The concept of application is as follows: The program will read numbers from a file - data.txt - that you will create, where the weight is a double (greater than 0.0 and less than or equal to 1.0) and the other numbers are the number, n, of lowest values to drop and then the numbers to be averaged after dropping the lowest n values. Example data.txt file would display: 0.5 3 10 70 90 80 20 The program then calculates the weighted average of all those numbers except the lowest n numbers, where…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
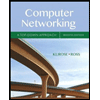
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
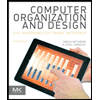
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
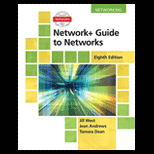
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
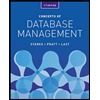
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
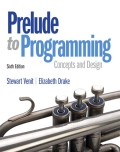
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
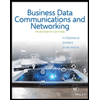
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY