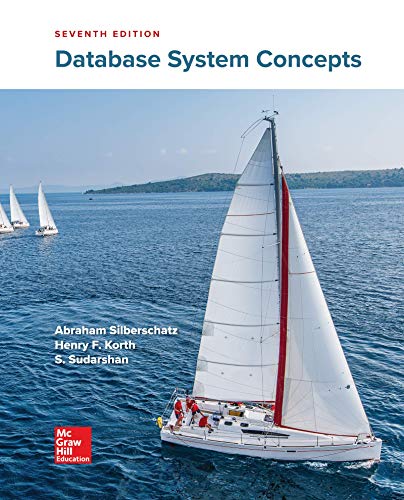
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
![This problem has you write a nested loop to process a list of list of int, and accumulate a list of list of int. The starter code
provides an accumulator for the result and a loop over the lists, and you need to write the code that checks whether sublist contains only
even ints.
You'll probably want a one-way flag: a Boolean variable that starts out as True and is set to False if you find an odd int in the sublist. You'll
need to check the value of this variable to figure out whether to append sublist to the even_lists accumulator.
1 def only_evens (1st: list[list[int]]) -> list[list[int]]:
"""Return a list of the lists in 1st that contain only even integers.
4
>>> only_evens ([[1, 2, 4], [4, 0, 6], [22, 4, 3], [2]])
[[4, 0, 6], [2]]
6.
8.
even_lists =
[]
10
for sublist in 1st:
11
12
# write your code here (please read above for a suggested approach)
13
14
return even_lists](https://content.bartleby.com/qna-images/question/2e9e339e-2841-4d7f-bb32-e8e5b2ddcf58/5c90a53a-8faa-4794-8d61-1dd0b9b4d69e/ljvgi8r_thumbnail.jpeg)
Transcribed Image Text:This problem has you write a nested loop to process a list of list of int, and accumulate a list of list of int. The starter code
provides an accumulator for the result and a loop over the lists, and you need to write the code that checks whether sublist contains only
even ints.
You'll probably want a one-way flag: a Boolean variable that starts out as True and is set to False if you find an odd int in the sublist. You'll
need to check the value of this variable to figure out whether to append sublist to the even_lists accumulator.
1 def only_evens (1st: list[list[int]]) -> list[list[int]]:
"""Return a list of the lists in 1st that contain only even integers.
4
>>> only_evens ([[1, 2, 4], [4, 0, 6], [22, 4, 3], [2]])
[[4, 0, 6], [2]]
6.
8.
even_lists =
[]
10
for sublist in 1st:
11
12
# write your code here (please read above for a suggested approach)
13
14
return even_lists
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- en "MyDoubleLinkedList" class: te a method called "PrintListInReverse" to print the list backward. example, Givenarrow_forwardChange this code to show strings that help you cast twelve dice. They should be shown one at a time like this:The first one was A. The second one was B. Afterward, the sum should be shown on a different line.arrow_forwardIn the Happy Valley School System, children are classified by age as follows: less than 2, ineligible2, toddler3-5, early childhood6-7, young reader8-10, elementary11 and 12, middle13, impossible14-16, high school17-18, scholargreater than 18, ineligible Given an int variable age , write a switch statement that prints out the appropriate label from the above list based on agearrow_forward
- Write the following method that returns the sum of all numbersin an ArrayList:public static double sum(ArrayList<Double> list)Write a test program that prompts the user to enter five numbers, stores them inan array list, and displays their sum.arrow_forwardFaceUp card game In this assignment we will implement a made-up card game we'll call FaceUp. When the game starts, you deal five cards face down. Your goal is to achieve as high a score as possible. Your score only includes cards that are face up. Red cards (hearts and diamonds) award positive points, while black cards (clubs and spades) award negative points. Cards 2-10 have points worth their face value. Cards Jack, Queen, and King have value 10, and Ace is 11. The game is played by flipping over cards, either from face-down to face-up or from face-up to face-down. As you play, you are told your total score (ie, total of the face-up cards) and the total score of the cards that are face down. The challenge is that you only get up to a fixed number of flips and then the game is over. Here is an example of the output from playing the game: FACE-DOWN | FACE-DOWN | FACE-DOWN | FACE-DOWN | FACE-DOWN Face up total: 0 Face down total: -5 Number of flips left: 5 Pick a card to flip between 1…arrow_forwardPrimeAA.java Write a program that will tell a user if their number is prime or not. Your code will need to run in a loop (possibly many loops) so that the user can continue to check numbers. A prime is a number that is only divisible by itself and the number 1. This means your code should loop through each value between 1 and the number entered to see if it’s a divisor. If you only check for a small handful of numbers (such as 2, 3, and 5), you will lose most of the credit for this project. Include a try/catch to catch input mismatches and include a custom exception to catch negative values. If the user enters 0, the program should end. Not only will you tell the user if their number is prime or not, you must also print the divisors to the screen (if they exist) on the same line as shown below AND give a count of how many divisors there are. See examples below. Your program should run the test case exactly as it appears below, and should work on any other case in general. Output…arrow_forward
- Practice / Frequency of Characters Write a method that returns the frequency of each characters of a given String parameter. If the given String is null, then return null If the given String is empty return an empty map Example input: output: empty map explanation: input is empty Example input: null output: null explanation: input is null. Since problem output is focused on the frequency we can comfortably use Map data structure. Because we can use characters as key and the occurrences of them as value. Example input: responsible output: {r=1, e=2, s=2, p=1, o=1, n=1, i=1, b=1, l=1} explanation: characters are keys and oc values ences arearrow_forwardComputer Science Part C: Interactive Driver Program Write an interactive driver program that creates a Course object (you can decide the name and roster/waitlist sizes). Then, use a loop to interactively allow the user to add students, drop students, or view the course. Display the result (success/failure) of each add/drop.arrow_forwardA for construct is an iterative loop that processes a list of items. As a consequence, it continues to process items as long as there are things to process. Is this a true or false statement?arrow_forward
- Java - Elements in a Rangearrow_forwardplease name this cards.py. You may use the following code in your program: import random SUITS = "♠ ♡ ♢ ♣".split()RANKS = "2 3 4 5 6 7 8 9 10 J Q K A".split() def create_deck(shuffle=False): """Create a new deck of 52 cards""" deck = [(s, r) for r in RANKS for s in SUITS] if shuffle: random.shuffle(deck) return deck This code creates a random deck of cards (each card is a tuple consisting of a rank and suit). You are to ask the user how many 5-card hands to play and will keep track of the following poker hands. You will need to reshuffle the deck as many times as necessary to get the required number of hands. An example run of the program is as follows. Enter the number of hands to deal: 10000Hand % of Hands Hands of Type2 of a kind 41.290% 4129 3 of a kind 1.970% 197 4 of a kind 0.010% 1 2 pair 4.860% 486 Full house 0.150% 15 Flush 0.220% 22 If you deal enough…arrow_forwardComplete the method “readdata”. In this method you are to read in at least 10 sets of student data into a linked list. The data to read in is: student id number, name, major (CIS or Math) and student GPA. You will enter data of your choice. You will need a loop to continue entering data until the user wishes to stop. Complete the method “printdata”. In this method, you are to print all of the data that was entered into the linked list in the method readdata. Complete the method “printstats”. In this method, you are to search the linked list and print the following: List of student’s id and names who are CIS majors List of student’s id and names who are Math majors List of student’s names along with their gpa who are honor students (gpa 3.5 or greater) All information for the CIS student with the highest gpa (you may assume that different gpa values have been entered for all students) CODE (student_list.java) You MUST use this code: package student_list;import…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
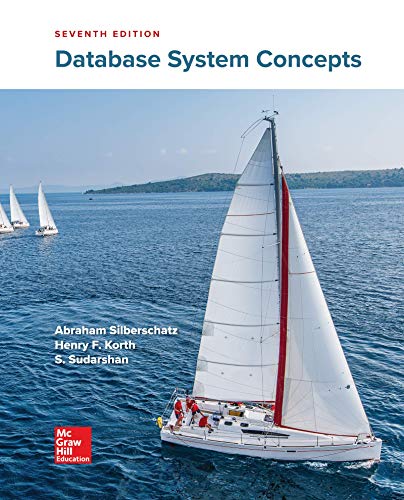
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
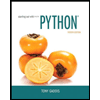
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
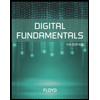
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
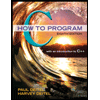
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
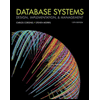
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
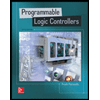
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education