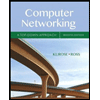
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
We will be looking at a problem known as the partition problem. The partition problem is to determine
if a given set can be partitioned into two subsets such that the sum of elements in both subsets is the
same. This is a variant of the 0-1 knapsack problem.
Instead of printing 'Set can be partitioned', use Backtracking so that the python program print the partitioned subsets.
Python program:
# Returns true if there exists a sublist of list `nums[0…n)` with the given sum
if a given set can be partitioned into two subsets such that the sum of elements in both subsets is the
same. This is a variant of the 0-1 knapsack problem.
Instead of printing 'Set can be partitioned', use Backtracking so that the python program print the partitioned subsets.
Python program:
# Returns true if there exists a sublist of list `nums[0…n)` with the given sum
def subsetSum(nums, total):
n = len(nums)
# `T[i][j]` stores true if subset with sum `j` can be attained
# using items up to first `i` items
T = [[False for x in range(total + 1)] for y in range(n + 1)]
# if the sum is zero
for i in range(n + 1):
T[i][0] = True
# do for i'th item
for i in range(1, n + 1):
# consider all sum from 1 to total
for j in range(1, total + 1):
# don't include the i'th element if `j-nums[i-1]` is negative
if nums[i - 1] > j:
T[i][j] = T[i - 1][j]
else:
# find the subset with sum `j` by excluding or including the i'th item
T[i][j] = T[i - 1][j] or T[i - 1][j - nums[i - 1]]
# return maximum value
return T[n][total]
# Returns true if given list `nums[0…n-1]` can be divided into two
# sublists with equal sum
def partition(nums):
total = sum(nums)
# return true if the sum is even and the list can be divided into
# two sublists with equal sum
return (total & 1) == 0 and subsetSum(nums, total // 2)
if __name__ == '__main__':
# Input: a set of items
nums = [7, 3, 1, 5, 4, 8]
if partition(nums):
print('Set can be partitioned')
else:
print('Set cannot be partitioned')
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

Knowledge Booster
Similar questions
- Use Javaarrow_forwardmplement a Java program that applies the Newton-Raphson's method xn+1 = xn – f(xn) / f '(xn) to search the roots for this polynomial function ax6 – bx5 + cx4 – dx3+ ex2 – fx + g = 0. Fill out a, b, c, d, e, f, and g using the first 7 digits of your ID, respectively. For example, if ID is 4759284, the polynomial function would be 4x6 – 7x5 + 5x4 – 9x3+ 2x2 – 8x + 4 = 0. The program terminates when the difference between the new solution and the previous one is smaller than 0.00001 within 2000 iterations. Otherwise, it shows Not Found as the final solution.arrow_forwardIn pythonarrow_forward
- In Python, Compute the: sum of squares total (SST), sum of squares regression (SSR), sum of squares error(SSE), and the r, R^2 values. You can use the sum() and mean() built-in functions. Here are the x and y values to use. x = [-5,-1,3,7,5,10] y = [-10,-3,5,8,7,10]arrow_forwardI have a block of Python. How do I modify it to solve this classical mechanics problem (questions are in the picture)? import matplotlib.pyplot as pltimport numpy as npimport os # This solves for x such that 1+tanh(x)=alpha *x# The program first prompts for alpha print("This find zeros of function 1+tanh(x)-alpha*x")alpha=float(input("Enter alpha: ")) # This defines a function F(x) and its derivative Fprime(x)def GetFFprime(x): F=1.0+np.tanh(x)-alpha*x Fprime=1.0/(np.cosh(x)*np.cosh(x)) -alpha return F,Fprime xguess=float(input("Guess a value of x: "))yarray=[] # Make arrays with no elementsxarray=[]xarray.append(xguess) # Add element with guess for first valueyarray.append(0.0)biggestx=xarray[0] offby=1.0E20 # used to check convergencenarray=0while offby>1.0E-7 and narray<10: yarray[narray],yprime=GetFFprime(xarray[narray]) print("x=",xarray[narray]," y=",yarray[narray]) offby=np.fabs(yarray[narray]) xarray.append(0.0) yarray.append(0.0)…arrow_forwardI need python solution please! Thank youarrow_forward
- In python, The function slice_end takes a string parameter, word, and a positive integer n; the function returns a slice of length n from the end of word. However, if word has length less than n, then the None object is returned instead. Hint: Notice that returning the empty string when n is zero might be a special case. You can use an if-elif-else decision structure and the slicing operator [ : ] to solve this problem. For example: Test Result word = "pineapple" n = 5 print(slice_end(word, n)) apple word = "too short" n = 20 print(slice_end(word, n)) None word = "llama" n = 0 print(slice_end(word, n)) word = "Saturn" n = 6 print(slice_end(word, n)) Saturnarrow_forwardI need the code from start to end with no errors and the explanation for the code ObjectivesJava refresher (including file I/O)Use recursionDescriptionFor this project, you get to write a maze solver. A maze is a two dimensional array of chars. Walls are represented as '#'s and ' ' are empty squares. The maze entrance is always in the first row, second column (and will always be an empty square). There will be zero or more exits along the outside perimeter. To be considered an exit, it must be reachable from the entrance. The entrance is not an exit.Here are some example mazes:mazeA7 9# # ###### # # ## # # #### # ## ##### ## ########## RequirementsWrite a MazeSolver class in Java. This program needs to prompt the user for a maze filename and then explore the maze. Display how many exits were found and the positions (not indices) of the valid exits. Your program can display the valid exits found in any order. See the examples below for exact output requirements. Also, record…arrow_forwardmake a C program that implements the "guess my number" game. The computer chooses a random number using the following random generator function srand(time(NULL)); int r = rand() % 100 + 1; that creates a random number between 1 and 100 and puts it in the variable r. (Note that you have to include <time.h>) Then it asks the user to make a guess. Each time the user makes a guess, the program tells the user if the entered number is larger or smaller than its number. The user then keeps guessing till he/she finds the number. If the user doesn't find the number after 10 guesses, a proper game over message will be shown and the actual guess is revealed. If the user makes a correct guess in its allowed 10 guesses, then a proper message will be shown and the number of guesses the user made to get the correct answer is also printed. After each correct guess or game over, the user decides to play again or quit and based on the user choice, the computer will make another guess and…arrow_forward
- Start with a pile of n stones and successively split a pile into two smaller piles until each pile has only one Each time a split happens, multiply the number of stones in each of the two smaller piles. (For example, if a pile has 15 stones and you split it into a pile of 7 and another pile of 8 stones, multiply 7 and 8.) The goal of this problem is to show that no matter how the pile of n stones are split, the sum of the products computed at each split is equal to n(n - 1)/2. Using strong mathematical induction, prove that no matter how the pile of n stones are split, the sum of the products computed at each split is equal to n(n - 1)/2.arrow_forwardThe following code is implementing a Treasure map use the A* algorithm to find the shortest path between two points in a map. It appears that the initial compare looks at the f_cost and decides that we are at the point in the map and exits the routine and prints only the starting point. I'm not sure how to fix it, any help would get appreciated. Example of the output from the following Python program: start place = (0, 0) Treasure location: (29, 86) Path to treasure:(0, 0) Python Code: import heapqimport random class Tile: def __init__(self, x, y): self.x = x self.y = y self.is_obstacle = False self.g_cost = 0 self.h_cost = 0 self.f_cost = 0 self.parent = None # Define comparison methods for Tile objects based on their f_cost def __lt__(self, other): return self.f_cost < other.f_cost def __eq__(self, other): return self.f_cost == other.f_cost class Map: def __init__(self, width, height):…arrow_forwardWrite a python code using IntegerRoot class that calculates integer nth root of a number m ifits nth root is an integer. For example: (m,n) where m = 1881676372240757194277820616164488626666147700309108518161,n = 3, then the integer nth root of m (i.e. the integer cubic root of m ) is: rgives an out put r = 12345678900987654321.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
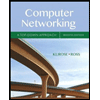
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
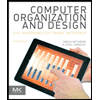
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
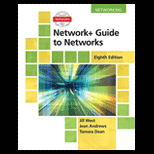
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
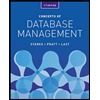
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
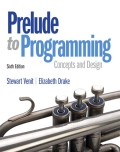
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
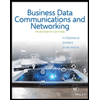
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY