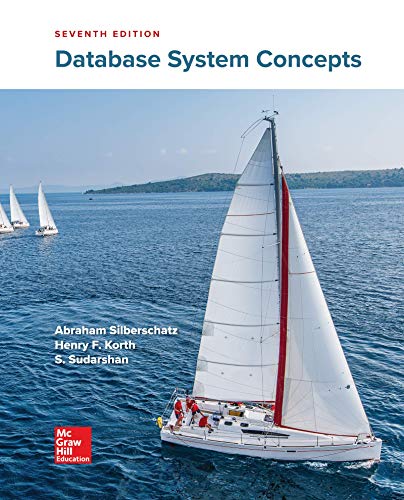
USING PYTHON
Design a class named Triangle that extends the GeometricObject class.
• Three float data fields named side1, side2, and side3 to denote the three sides of the triangle.
• A constructor that creates a triangle with the specified side1, side2, and side3 with default values 1.0.
• The accessor methods for all three data fields.
• A method named getArea() that returns the area of this triangle. • A method named getPerimeter() that returns the perimeter of this triangle.
• A method named __str__() that returns a string description for the triangle. For the formula to compute the area of a triangle, see Exercise 2.14. The __str__() method is implemented as follows: return "Triangle: side1 = " + str(side1) + " side2 = " + str(side2) + " side3 = " + str(side3)
Write a test program that prompts the user to enter the three sides of the triangle, a color, and 1 or 0 to indicate whether the triangle is filled. The program should create a Triangle object with these sides and set the color and filled properties using the input. The program should display the triangle’s area, perimeter, color, and True or False to indicate whether the triangle is filled or not.

Step by stepSolved in 3 steps with 4 images

- Write a program that will contain an array of person class objects. The program should include two classes: 1) One class will be the person class. 2) The second class will be the team class, which contain the main method and the array or array list. The person class should include the following data fields; Name Phone number Birth Date Jersey Number Be sure to include get and set methods for each data field.The team class should contain the data fields for the team, such as: Team name Coach name Conference name The program should include the following functionality. Add person objects to the array; Find a specific person object in the array; (find a person using any data field you choose such as name or jersey number) Output the contents of the array, including all data fields of each person object. (display roster)arrow_forwardHelp me solve this in Java, don't make it to complicated.arrow_forwardWritten in Python It should have an init method that takes two values and uses them to initialize the data members. It should have a get_age method. Docstrings for modules, functions, classes, and methodsarrow_forward
- Make C# (Sharp): console project named MyPlayList. Create an Album class with 4 fields to keep track of title, artist, genre, and copies sold. Each field should have a get/set property. Your Album class should have a constructor to initialize the title (other 3 variables should default to null or 0). In addition, create a public method in the Album class to calculate amount sold for the album (copies sold * cost_of_album). You determine the cost of each album. Also create a method in the Album class to display the information. Print out of information should look something like this: The album Tattoo You by the Rolling Stones, a Rock group, made $25,000,000. In the main method, create 3 instances of the Album class with different albums, use setters to set values of the artist, genre, and copies sold. And then use getters to display information about each on the console.arrow_forwardIn python and include doctring: First, write a class named Movie that has four data members: title, genre, director, and year. It should have: an init method that takes as arguments the title, genre, director, and year (in that order) and assigns them to the data members. The year is an integer and the others are strings. get methods for each of the data members (get_title, get_genre, get_director, and get_year). Next write a class named StreamingService that has two data members: name and catalog. the catalog is a dictionary of Movies, with the titles as the keys and the Movie objects as the corresponding values (you can assume there aren't any Movies with the same title). The StreamingService class should have: an init method that takes the name as an argument, and assigns it to the name data member. The catalog data member should be initialized to an empty dictionary. get methods for each of the data members (get_name and get_catalog). a method named add_movie that takes a Movie…arrow_forwardDescription# Write a class called Song in a file called Song.h with three fields: Title (string)Singer (string)Chart Position (int)# Place these method headers in the Song.h file 1. Getter and setter for each field2. Other methods1. Song(); // default constructor2. Song(std::string title,std:: string singer, int chartPosition);// custom constructor3. std::string toString(); // returns object as a string4. bool operator<(Song other)// overloads the < operator -returns true if the chart position of this song is less other’s5. friend std::ostream & operator<<(std::ostream&, Song* s); // overloads the << operator # Write the implementation of these methods in a file called Song.cpp # Write a driver called SongMain.cpp that does the followinga. Creates a dynamic array that reads in data from SongData.txt andb. Populates the array by constructing song objects from this data c. Uses the non-recursive selection sort method to sort the array according to chart position…arrow_forward
- What is the purpose of a constructor? It creates an array of data values associated with an object. It prints all of the information about our object. It returns all data members from an object back to the main method. It allows us to set our own default values when we create an object. in javaarrow_forwardFocus on classes, objects, methods and good programming styleYour task is to create a BankAccount class. Class name BankAccount Attributes __balance float float __pin integer integer Methods __init_()get_pin()check_pin()deposit()withdraw()get_balance() The bank account will be protected by a 4-digit pin number (i.e. between 1000 and 9999). The pin should be generated randomly when the account object is created. The initial balance should be 0.get_pin()should return the pin.check_pin(pin) should check the argument against the saved pin and return True if it matches, False if it does not.deposit(amount) should receive the amount as the argument, add the amount to the account and return the new balance.withraw(amount) should check if the amount can be withdrawn (not more than is in the account), If so, remove the argument amount from the account and return the new balance if the transaction was successful. Return False if it was not.get_balance()…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
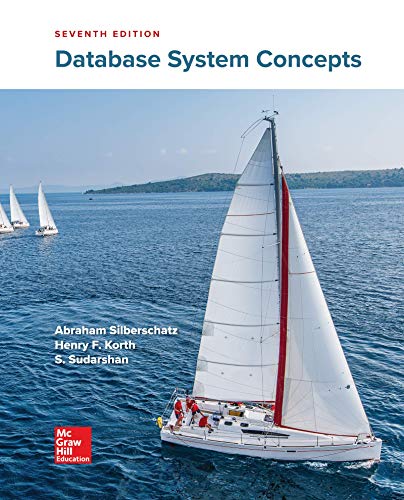
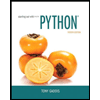
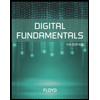
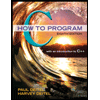
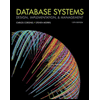
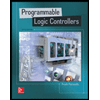