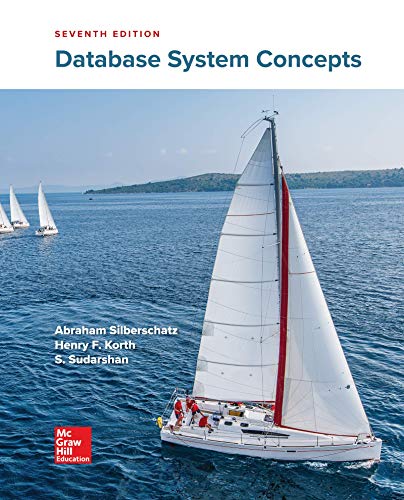
Using TKinter, display the Dictionary in the Dictionary Based Object streamed in question #4.
question #4:
import socket
import json
def receive_co2_data(host, port):
"""
Connects to a CO2 data server at the specified host and port,
receives and prints the CO2 data fields, and gracefully closes
the connection.
Args:
- host (str): The hostname or IP address of the server.
- port (int): The port number to connect to.
"""
# Initialize socket
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
try:
# Connect to server
client_socket.connect((host, port))
print(f"Connected to {host}:{port}")
# Receive and print the CO2 data fields
buffer = ""
while True:
data = client_socket.recv(1024).decode()
if not data:
break
# Buffer data and split into JSON-encoded objects
buffer += data
objects = buffer.split("\n")
buffer = objects.pop()
# Parse and print each object
for obj in objects:
try:
field_data = json.loads(obj)
print(field_data)
except json.JSONDecodeError:
pass
print("Connection closed")
except ConnectionRefusedError:
print(f"Error: connection to {host}:{port} refused")
except OSError as e:
print(f"Error: {e}")
finally:
# Close the socket
client_socket.close()
if __name__ == "__main__":
host = input("Enter server hostname or IP address: ")
port = int(input("Enter server port number: "))
receive_co2_data(host, port)

Step by stepSolved in 3 steps

- Please help me with this Please fix the full code: this code is supposed to work in a web browser (Client_side.js) const url = "http://localhost:3000/document"; let undoStack = [];let redoStack = []; function sendDataToServer(data) { fetch(url, { method: 'POST', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify({ content: data }) }) .then(response => response.json()) .then(data => console.log('Success:', data)) .catch((error) => console.error('Error:', error));} function formatText(command) { document.execCommand(command, false, null);} function changeColor() { const color = prompt("Enter text color code:"); document.execCommand("foreColor", false, color);} function changeFont() { const font = prompt("Enter font:"); document.execCommand("fontName", false, font);} function changeFontSize() { const size = prompt("Enter font size:"); document.execCommand("fontSize", false, size);}…arrow_forwardto create a SortedList object with three names and IP addresses, show the code.to create a SortedList object with three names and IP addresses, show the code.to create a SortedList object with three names and IP addresses, show the code.arrow_forwardQ: In a multiplayer game, players move characters around of a common scene. Game state is replicated on player machines and on a server, which contains the services that control the game as a whole, such as collision detection. Updates are multicast to all replicas. Consider the following conditions and answer each of the questions asked:i) Characters can launch projectiles at each other, and a hit weakens the unfortunate recipient for a limited time. Indicate what type of Update request is required here. Explain why yourchoice. Hint: consider the events 'throw', 'crash' and 'revive'.ii) The game incorporates magical objects that can be picked up by a player to help you. Indicate which type of order should be applied to the "object collection" operation. Explain the reason for the choice.arrow_forward
- Please help me with my code in python. Can you change the start_tag and end_tag to strip. Since we have not discussed anything about tag yet. Thank you def read_data(): with open("simple.xml", "r") as file: content = file.read() return content def extract_data(tag, string): data = [] start_tag = f"<{tag}>" end_tag = f"</{tag}>" while start_tag in string: start_index = string.find(start_tag) + len(start_tag) end_index = string.find(end_tag) value = string[start_index:end_index] data.append(value) string = string[end_index + len(end_tag):] return data def get_names(string): names = extract_data("name", string) return names def get_calories(string): calories = extract_data("calories", string) return calories def get_descriptions(string): descriptions = extract_data("description", string) return descriptions def get_prices(string): prices = extract_data("price", string) return prices def…arrow_forwardPart C Consider the standard web log file in assets/logdata.txt. This file records the access a user makes when visiting a web page (like this one!). Each line the log has the following items: • a host (e.g., '146.204.224.152') a user_name (e.g., 'feest6811' note: sometimes the user name is missing! In this case, use '-' as the value for the username.) • the time a request was made (e.g., '21/Jun/2019:15:45:24 -0700') • the post request type (e.g., 'POST /incentivize HTTP/1.1' note: not everything is a POST!) Your task is to convert this into a list of dictionaries, where each dictionary looks like the following: example_dict = {"host":"146.204.224.152", "user_name":"feest6811", "time":"21/Jun/2019:15:45:24 -0700", "request":"POST /incentivize HTTP/1.1"} In [ ]: import re def logs(): with open ("assets/logdata.txt", "r") as file: logdata = file.read() # YOUR CODE HERE raise NotImplementedError(() In [ ]: assert len(logs()) == 979 one_item={'host': '146.204.224.152', 'user_name':…arrow_forwardWhich of the following problems occur due to linear probing? O Primary clustering Secondary collision O Secondary hashing O Direct addressingarrow_forward
- Without using the built in java hash table Write a Java program to get input from the keyboard to enter an integer.Write a hash function to hash the integer.Store the integer in a DataItem object.Create a hash table to store the DataItem in the hash table at the hashed location.Write a function to search a hash function for an integer value and display it from the DataItemobject at the hashed locationarrow_forwardIn what situations is it helpful to have data stored in an array with several dimensions?arrow_forwardWrite a script that creates an inventory of what is present in a user-defined .aprx file. The aprx file path will be hardcoded at the top of the script. The inventory should consist of the following: 1) the full path of the .aprx file; 2) the number of maps and layouts; 3) for each map, the name of the map, the number of layers, whether a basemap is one of the layers or not, and the coordinate system of the map; and 4) for each layout, the name of the layout, the number of map frames, the number of text elements and the number of other layout elements. The inventory should be written as a text file I believe this needs to use the arcpy package in Pythonarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
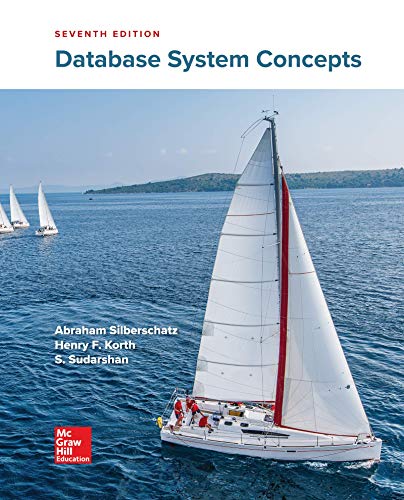
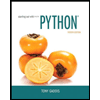
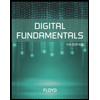
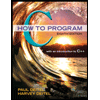
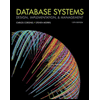
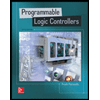