What does the following code output? tinclude void pointerUpd (double* upt); int main() { double* pp: double b[6] = { 1.01, 2.02, 3.03, 4.04, 5.05 }; pp = 6 (b[4]); double *dp = pp; dp++; std::cout << *dp << std::endl:
![What does the following code output?
#include <iostream>
void pointerUpd (double* upt);
int main() {
double* pp;
double b[6] = { 1.01, 2.02, 3.03, 4.04, 5.05 };
pp = & (b[4]);
double *dp = pp;
dp++;
std::cout « *dp << std::endl;
std::cout <« *pp « std::endl;
pointerUpd (pp);
std::cout <« *dp << std::endl;
std::cout << *pp << std::endl;
void pointerUpd (double* upt) {
* (++upt) = 6.06;
upt = new double;
*upt = 1.01;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0dc061a5-6ce8-4788-a9b1-d5cfea3c8a74%2Fc8b4480e-eea9-4159-8b2b-c1658c0b1359%2F1zjqza_processed.png&w=3840&q=75)

The C++ code given in the problem:
#include <iostream>
void pointerUpd(double* upt);
int main()
{
double* pp;
double b[6]={1.01,2.02,3.03,4.04,5.05};
pp=&(b[4]);
double *dp=pp;
dp++;
std::cout<< *dp <<std::endl;
std::cout<< *pp <<std::endl;
pointerUpd(pp);
std::cout<< *dp <<std::endl;
std::cout<< *pp <<std::endl;
}
void pointerUpd(double* upt){
*(++upt)=6.06;
upt=new double;
*upt=1.01;
}
From the code given in the problem:
pp is a pointer of type double.
b is an array having capacity 6 but 5 elements are present
pp is storing the address of the last element of the array i.e., (b[4]=5.05).
dp is another pointer of type double which pointing to pp.
dp is incremented and as dp is pointing to pp and pp was storing the last element of the array.
So, dp is now pointing to the sixth element or b[5] which by default stores the value of 0 as it was empty.
So, *dp=b[5]=0
and *pp=b[4]=5.05.
pointerUpd function is called and pointer pp is passed as parameter.
By using pointerUpd function, the last value of the array i.e., b[5] is changed to 6.06.
As dp is pointing to b[5] and pp is pointing to b[4] so after the execution of the pointerUpd function,
*dp=b[5]=6.06
and *pp=b[4]=5.05.
Step by step
Solved in 3 steps with 2 images

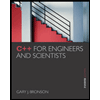
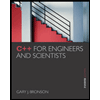