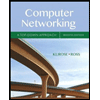
#include <iostream>
using namespace std;
void insertElement(int* LA, int ITEM, int N, int K)
{
int J=N; //initialize j to n
while(J>=K) //enters loop only if j less than or equal to k
{
LA[J+1]=LA[J]; //stores current value in next index
J=J-1; //decrement j
}
LA[K]=ITEM; //insert element at index k
N=N+1; //increment n by 1
//this is just used for printing and you can ignore if not required
for(int i=0;i<N;i++) //i from 0 to n
{
cout << LA[i] << " "; //print element at index i
}
}
int main()
{
int LA[6]={1,2,3,4,5}; //declare an array and initialize values to it
insertElement(LA,6,5,3); //call function to insert 6 at index 3
return 0;
}
note: Remove Funcation

Step by stepSolved in 3 steps with 1 images

- /* yahtC */ #include <stdio.h> #include <string.h> #include <stdlib.h> #include <time.h> void seed(int argc, char ** argv){ srand(time(NULL)); // Initialize random seed if (argc>1){ int i; if (1 == sscanf(argv[1],"%d",&i)){ srand(i); } } } void instructions(){ printf("\n\n\n\n" "\t**********************************************************************\n" "\t* Welcome to YahtC *\n" "\t**********************************************************************\n" "\tYahtC is a dice game (very) loosely modeled on Yahtzee\n" "\tBUT YahtC is not Yahtzee.\n\n" "\tRules:\n" "\t5 dice are rolled\n" "\tThe user selects which dice to roll again.\n" "\tThe user may choose to roll none or all 5 or any combination.\n" "\tAnd then the user selects which dice to roll, yet again.\n" "\tAfter this second reroll the turn is scored.\n\n" "\tScoring is as follows:\n" "\t\t*\t50 points \t5 of a kind scores 50 points.\n" "\t\t*\t45 points \tNo pairs (all unique) scores 45…arrow_forward// // main.c // Assignment1 // // Created by Hassan omer on 15/10/21. // #include <stdio.h> # include <stdlib.h> int input(); int multiples(); int cions(); void display_change(); int main() { int num; num = input(); multiples(num); cions(); display_change(); return 0; } int input(int num) { printf("enter 5-95 number\n"); scanf("%d",&num); return num; } int multiples(int num) { int sum5 =0; if (sum5 %5 != 0 ||sum5<5|| sum5 >95) { printf("invaild input %d",sum5); } cions(sum5); return sum5; } int cions(int sum05){ int cent50 = 0; int cent20 = 0; int cent10 = 0; int cent05 = 0; if (sum05 > 0) { if (sum05 >= 50){ sum05 -= 50; cent50++; } else if (sum05 >=20){ sum05 -= 20; cent20++; } else if (sum05 >= 10){ sum05 -=10;…arrow_forward#include <iostream>using namespace std; char* duplicateWithoutBlanks(char *word){int len = sizeof(word); char *new_str = new char[len + 1]; int k = 0; for (int i = 0; i < len; ++i){if (word[i] != ' ')new_str[k++] = word[i];} new_str[k] = '\0'; return new_str;} int main(){// Testing code char word[] = "Hello, World!"; char* result = duplicateWithoutBlanks(word); cout<< "word: "<< word<< "\nResult: "<< result; ; return 0;} What to change to out put the same word without blank?arrow_forward
- 3. int count(10); while(count >= 0) { count -= 2; std::cout << count << endl;arrow_forwardQuestion 37 public static void main(String[] args) { Dog[] dogs = { new Dog(), new Dog()}; for(int i = 0; i >>"+decision()); } class Counter { private static int count; public static void inc() { count++;} public static int getCount() {return count;} } class Dog extends Counter{ public Dog(){} public void wo(){inc();} } class Cat extends Counter{ public Cat(){} public void me(){inc();} } The Correct answer: Nothing is output O 2 woofs and 5 mews O 2 woofs and 3 mews O 5 woofs and 5 mews Oarrow_forward#include<stdio.h>#include<string.h>struct info{char names[100];int age;float wage;};int main(int argc, char* argv[]) {int line_count = 0;int index, i;struct info hr[10];if (argc != 2)printf("Invalid user_input!\n");else {FILE* contents = fopen (argv[1], "r");struct info in;if (contents != NULL) {printf("File has been opened successfully.\n\n");while (fscanf(contents, "%s %d %f\n",hr[line_count].names, &hr[line_count].age, &hr[line_count].wage) != EOF) {printf("%s %d %f\n", hr[line_count].names, hr[line_count].age, hr[line_count].wage);line_count++;}printf("\nTotal number of lines in document are: %d\n\n", line_count);fclose(contents);printf("Please enter a name: ");char user_input[100];fgets(user_input, 30, stdin);user_input[strlen(user_input)-1] = '\0';index = -1;for(i = 0; i < line_count; i++){if(strcmp(hr[i].names, user_input) == 0){index = i;break;}}if(index == -1)printf("Name %s not found\n", user_input);else{while(fread(&in, sizeof(struct info), 1,…arrow_forward
- 8. Know how to do these, to trace functions like these and to debug functions like these: // recursive power , compute xn int exp(int x, int n){ if(n== return return *exp( ); } void main(){ int a,b; cin >>a>>b; cout>a; rev_print(a); }arrow_forwardinitial c++ file/starter code: #include <vector>#include <iostream>#include <algorithm> using namespace std; // The puzzle will always have exactly 20 columnsconst int numCols = 20; // Searches the entire puzzle, but may use helper functions to implement logicvoid searchPuzzle(const char puzzle[][numCols], const string wordBank[],vector <string> &discovered, int numRows, int numWords); // Printer function that outputs a vectorvoid printVector(const vector <string> &v);// Example of one potential helper function.// bool searchPuzzleToTheRight(const char puzzle[][numCols], const string &word,// int rowStart, int colStart) int main(){int numRows, numWords; // grab the array row dimension and amount of wordscin >> numRows >> numWords;// declare a 2D arraychar puzzle[numRows][numCols];// TODO: fill the 2D array via input// read the puzzle in from the input file using cin // create a 1D array for wodsstring wordBank[numWords];// TODO:…arrow_forwardC++ The binary search algorithm given in this chapter is nonrecursive. Write and implement a recursive version of the binary search algorithm. The program should prompt Y / y to continue searching and N / n to discontinue. If an item is found in the list display the following message: x found at position y else: x is not in the list Use the following list of integers when testing your program: 2, 6, 8, 13, 25, 33, 39, 42, 53, 58, 61, 68, 71, 84, 97arrow_forward
- #include<bits/stdc++.h>using namespace std;bool isPalindrome(string &s){ int start=0; int end=s.length()-1; while(start<=end) { if(s[start]!=s[end]) { return false; } start++; end--; } return true;}int main(){ string s; cout<<"ENTER STRING:"; getline(cin,s); int n=s.length(); bool flag=true; for(int i=1;i<n;i++) { string lowerHalf=s.substr(0,i); string upperHalf=s.substr(i,n-i); if(isPalindrome(lowerHalf) && isPalindrome(upperHalf)) { flag=false; cout<<"String A is:"<<lowerHalf<<"\n"; cout<<"String B is:"<<upperHalf<<"\n"; break; } } if(flag) { cout<<"NO\n"; } return 0;} change to stdio.h string.harrow_forward// Assume all libraries are included 3 void func (int a, int &b, int &c); // int main () { int i = 5, j = 4, k = 33; 4 7 %3D 8. func (i, j, k); func (j, i, k); cout << j « k << i <« endl; 10 11 12 13 return 0; 14 } // 15 16 void func (int a, int &b, int &c) { 17 18 = 2*c + b; 19 b a; 20 C = a + 3*b; } // 21 22 I|||arrow_forward#include <stdio.h>#include <limits.h> int findMissingUtil(int arr[], int low, int high, int diff){ if (high <= low)return INT_MAX; int mid = low + (high - low)/2; if (arr[mid+1] - arr[mid] != diff)return (arr[mid] + diff); if (mid > 0 && arr[mid] - arr[mid-1] != diff)return (arr[mid-1] + diff); if (arr[mid] == arr[0] + mid*diff)return findMissingUtil(arr, mid+1, high, diff); return findMissingUtil(arr, low, mid-1, diff);} int findMissing(int arr[], int n){int diff = (arr[n-1] - arr[0])/n; return findMissingUtil(arr, 0, n-1,diff);} int main(){int arr[] = {120001, 120013, 120025, 120037, 120049, 120061,120085,120097,120109,120121};int n = sizeof(arr)/sizeof(arr[0]);printf("The missing element is %d", findMissing(arr, n));return 0;} ______________________________________________________________________________ Convert the code above into Pseudocodearrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
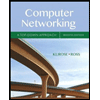
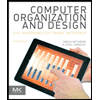
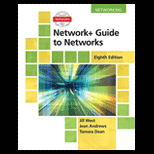
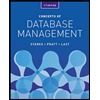
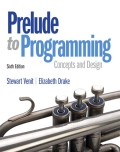
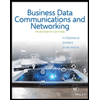