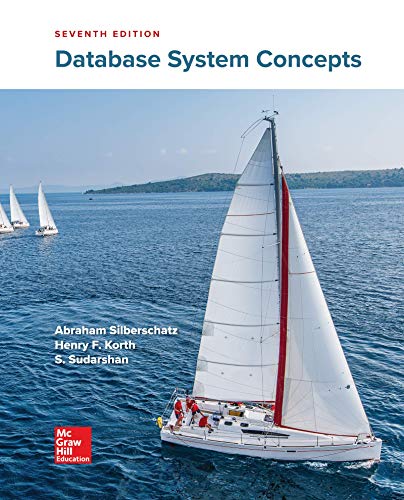
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
![COMP.1020 Computing II
UMASS - Lowell
#include <stdio.h>
char *reverse(char *word);
Write a function called reverse that accepts a c-string as an argument and reverses that argument in place
(meaning you should not create another array to store the result) returning the address of the first element
of the c-string as a character pointer when you are finished. For example, if your c-string contains the string
"Happy Birthday!" then after a call to the function your string would contain "lyadhtriB yppaH". For this
assignment you may not use the string.h library or any other library except stdio.h. You may assume the
following main program which would print the string forward, backward, and then forward again twice:
int main(int arge, char* argy [])
{
HW1 Spring 2023
char word [] "Happy Birthday!";
printf("%s\n", word);
reverse (word);
printf("%s\n", word);
printf("%s\n", reverse (word));
printf("%s\n", word);
return 0;
}
Place the code for your function in a file called reverse.c and submit only this file to me on blackboard. The
file should contain the definition for your function reverse and nothing else. I will use my own header file and
main program to test your code. Please keep in mind that this function should work for any valid C style
string and the fact that the above program works for "Happy Birthday!" is just one example. You should
try it with strings of even and odd lengths as well as very short strings.](https://content.bartleby.com/qna-images/question/17fb7c04-187c-4763-b6c5-1b444cd4b1ad/c5564dd9-dcd8-44c2-a9aa-ab05686efb76/yqf9pz9_thumbnail.jpeg)
Transcribed Image Text:COMP.1020 Computing II
UMASS - Lowell
#include <stdio.h>
char *reverse(char *word);
Write a function called reverse that accepts a c-string as an argument and reverses that argument in place
(meaning you should not create another array to store the result) returning the address of the first element
of the c-string as a character pointer when you are finished. For example, if your c-string contains the string
"Happy Birthday!" then after a call to the function your string would contain "lyadhtriB yppaH". For this
assignment you may not use the string.h library or any other library except stdio.h. You may assume the
following main program which would print the string forward, backward, and then forward again twice:
int main(int arge, char* argy [])
{
HW1 Spring 2023
char word [] "Happy Birthday!";
printf("%s\n", word);
reverse (word);
printf("%s\n", word);
printf("%s\n", reverse (word));
printf("%s\n", word);
return 0;
}
Place the code for your function in a file called reverse.c and submit only this file to me on blackboard. The
file should contain the definition for your function reverse and nothing else. I will use my own header file and
main program to test your code. Please keep in mind that this function should work for any valid C style
string and the fact that the above program works for "Happy Birthday!" is just one example. You should
try it with strings of even and odd lengths as well as very short strings.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- DO NOT COPY FROM OTHER WEBSITES Code with comments and output screenshot is must for an Upvote. Thank you!!!arrow_forwardC++ Programming: How would you write a function that takes a filename and an array of struct time, opens a file, reads each line in the file as the number of days, converts this to a struct time and stores this is in an array? Here is my code so far (p.s. the function i need help with is called void readData): #include<iostream>#include<string>#include<fstream>#include<cstdlib>#include<vector> using namespace std; struct time{// private:int years, months, days;//public: time(){}time(int days){}}; time getYearsMonthsDays(int days){time x;x.years = days/450;days = days%450;x.months = days/30;x.days = days%30;return x;} int getTotalDays(time x){int totalDays;totalDays = x.years*450 + x.months*30 + x.days;return totalDays;} void openofile(string ofilename,ofstream &fout){fout.open(ofilename.c_str());if(!fout){cout << "Error opening output file..." << endl;exit(1);}} void openifile(string ifilename,ifstream…arrow_forwardA C-string variable is an array, so we can use index operator [ ] to access individual character. True or false?arrow_forward
- Write a function named "changeCase" that takes an array of characters terminating by NULL character (C-string) and a boolean flag of toUpper. If the toUpper flag is true, it will go through the array and convert all lowercase characters to uppercase. Otherwise, it will convert all uppercase to lowercase. For example, if the array is {'H', 'e', 'l', 'l', 'o', '\0'} and the flag is true, then the array will become {'H', 'E', 'L', 'L', 'O', '\0'}. And if the flag is false, the array will become{'h', 'e', 'l', 'l', 'o', '\0'}arrow_forwardIn C++, define an integer vector and ask the user to give you values for the vector. Because you used a vector, so you don't need to know the size. user should be able to enter a number until he wants(you can use while loop for termination. for example if the user enters any negative number, but until he enters a positive number, your program should read and push it inside of the vector). the calculate the average of all numbers he entered.arrow_forwardWrite a function named rockPaperScissors. It should have one input:- An array with 2 elements, each representing a player in the game of Rock Paper Scissors.It should return one output- A scalar with the result of the game.For the purposes of this program, assume the following numerical code:- 1 is rock- 2 is paper- 3 is scissorsIn the game of Rock, Paper, Scissors, rock beats scissors, paper beats rock, and scissors bears paper. Ifplayers play the same object, the result is a tie.Your output should be:- The index of the winning player if there is a winner.- 0 if there is a tie- -1 if a player played an invalid object (a number that is not 1, 2, or 3)For example, if we called our function:result = rockPaperScissors([1 2]);result would be 2, as paper beats rock.If we called our functionresult = rockPaperScissors([3 2]);result would be 1 because scissors beats papearrow_forward
- python function!: a function called popular_color with one parameter, of type dict[str, str] of names and favorite colors. The return type is a str and it is the most commonly occuring color. If two colors are tied for popularity, the first color shown will be picked.arrow_forwardWhich of the following are true? O The C-library has many functions that are useful in manipulating strings. V If a and b are two different C-string variables, the value in a can be assigned the value in b with the following C-statement: a = b; %3D O The length of the char array holding the string must be at least one character longer than the string itself. O An array identifier cannot be used as a pointer. V The identifier, name, of a C-string is also a pointer to a char data type. The ending character in a C-string must be the newline character, '\n'. O The name of a 1-D array is a pointer to the data type of the array, while the name of a 2-D array is a pointer to a 1-D array of the data type.arrow_forwardplease use python.arrow_forward
- Please help in C++arrow_forwardhappy Eidarrow_forwardin c++ Write a function named “countTheSameAsLast” that accepts an array of pointers to Name objects and its array size. It will go through the array and return how many elements (int) in the array are the same as the last element and whether the first and the last elements are the same or not (bool). Please note that the objects pointed by elements in the array can be either Word or Item objects. Please show how you test with the list of mixed objects.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
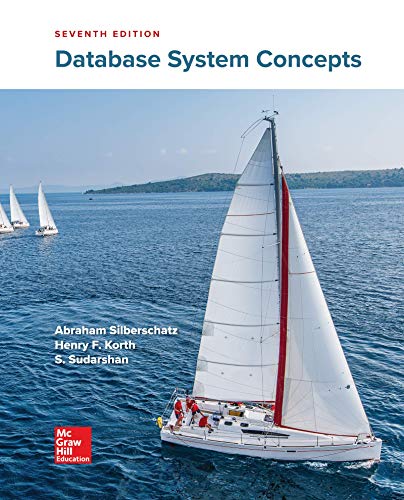
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
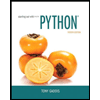
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
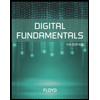
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
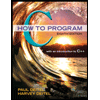
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
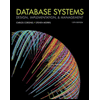
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
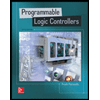
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education