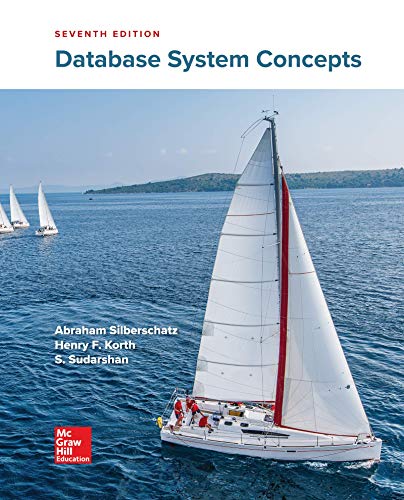
Write a function called word_length() that does not take any input parameter and returns
a 2-D list of length 10. This function repeatedly asks the user to enter words until they enter
the word "stop". Every unique word of length k, where 0 ≤ k ≤ 9, that the user enters should
be stored in the k'th element of the 2-D list. Words that have a length greater than 9 will be
ignored.
Sample run:
>>> the_list = user_words()
Enter a string, enter 'stop!' to quit: a
Enter a string, enter 'stop!' to quit: $
Enter a string, enter 'stop!' to quit: a
Enter a string, enter 'stop!' to quit: bbbb
Enter a string, enter 'stop!' to quit: ccc
Enter a string, enter 'stop!' to quit: dd
Enter a string, enter 'stop!' to quit: zzzzzzzzz
Enter a string, enter 'stop!' to quit: dd
Enter a string, enter 'stop!' to quit: efghi
Enter a string, enter 'stop!' to quit: abcdefghijk
Enter a string, enter 'stop!' to quit: $e#%^
Enter a string, enter 'stop!' to quit: *******
Enter a string, enter 'stop!' to quit:
Enter a string, enter 'stop!' to quit: bbbb
Enter a string, enter 'stop!' to quit: stop!
>>> print(the_list)
[[''], ['a', '$'], ['dd'], ['ccc'], ['bbbb'], ['efghi', '$e#%^'],
[], ['*******'], [], ['zzzzzzzzz']]
Note, both ‘a’ and ‘dd’ have been entered twice, only one copy of each is stored. The
input ‘abcdefghijk’ has more than 10 characters so it was not stored. Other than this,
the_list[0] contains a string (‘’) of length 0
the_list[1] contains all strings ('a', '$') of length 1
the_list[2] contains all strings ('dd') of length 2 and so on.

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- Can you use Python programming language to to this question? Thanksarrow_forwardplease code in pythonWrite a function that takes in a number and returns the sum of all primes less than or equal to that number. You'll need your answer to the previous problem to answer this.Example: sum_primes(11) == 2 + 3 + 5 + 7 + 11 == 28Bonus: Can you do this in one line with a list comprehension?arrow_forwardPython Complete the get_2_dimensional_list() function that takes two parameters: 1. An integer num_rows. 2. An integer num_columns. The function should return a 2D list with num_rows rows and num_columns columns. Each element is set to a random value between 1 and 99 included. If both parameters num_rows and num_columns are set 0, the function returns an empty list. You MUST use a for...in.range loop to create the 2D list. You do not need to import the random module as it has already been done for you. Some examples of the function being called are shown below. For example: Test random.seed (15) num_rows - 2 num columns 5 matrix = get_2_dimensional_list(num_rows, num_columns) print(matrix) random.seed (132) num_rows=2 num_columns 2 matrix = get_2_dimensional_list(num_rows, num_columns) print(matrix) Result [[27, 2, 67, 95, 5], [21, 31, 3, 8, 88]] [[53, 20], [53, 8]]arrow_forward
- I have given you the linear search code example on the video and I want you to go through the code and adjust the code. In the following code, list consists of number 4 twice however when you execute this code you find only the first 4. Please adjust your code to find both 4’s on the list def linear_search(list1,key): for i in range(len(list1)): if key==list1[i]: print("Element is found at index", i) break else: print("The element is not found") list1=[4,5,10,4,9,21] key=int(input("Enter a key number: ")) linear_search(list1,key)arrow_forwardWrite a function called mutate_list() that takes a 2D-list of integers as the input parameter and performs the following mutations: a. any even integer (non-zero) gets divided by 2, b. any odd integer less than 10 gets multiplied by 10, and c. any row (an element of the 2D-list) with less than 5 elements should be padded with zeroes to exactly 5 elements. Sample run, >>> print( mutate_list( [ [1, 2, 3], [20, 3, 40, 5, 60] ] ) ) [[10, 1, 30, 0, 0], [20, 30, 40, 50, 60]] >>> print( mutate_list( [[8,5,7,2],[13,12,9,17,5],[10,20],[1,2,3,4,5,6]] ) ) [[4, 50, 70, 1, 0], [13, 6, 90, 17, 50], [5, 10, 0, 0, 0], [10, 1, 30, 2, 50, 3]]arrow_forwardWrite a function called remove_odd that takes a list of numbers that have both even and odd numbers mixed.# Function should remove all the odd numbers and return a compact list which only contains the even numbers. Example1: Function Call:remove_odd ([21, 33, 44, 66, 11, 1, 88, 45, 10, 9])Output:[44, 66, 88, 10]arrow_forward
- Written in python with docstrings if applicable. Thanksarrow_forwardWrite a function little_sum (number_list, limit) that returns the sum of only the numbers in number_list that are less than or equal to the given limit. In this question you must use a for loop and you are not allowed to use the sum function. For example: Test Result numbers = [1, 2, 3, 4, 5, 6] total = little_sum(numbers, 3) print(total) values = [6, 3, 4, 1, 5, 2] 10 total = little_sum(values, 4) print(total)arrow_forwardhave been given a singly linked list of integers. Write a function that returns the index/position of integer data denoted by 'N' (if it exists). Return -1 otherwise. Note :Assume that the Indexing for the singly linked list always starts from 0.Input format :The first line contains an Integer 'T' which denotes the number of test cases. The first line of each test case or query contains the elements of the singly linked list separated by a single space. The second line contains the integer value 'N'. It denotes the data to be searched in the given singly linked list. Remember/Consider :While specifying the list elements for input, -1 indicates the end of the singly linked list and hence -1 would never be a list element. Output format :For each test case, return the index/position of 'N' in the singly linked list. Return -1, otherwise. Output for every test case will be printed in a separate line. Note:You do not need to print anything; it has already been taken care of. Just implement…arrow_forward
- Write a function called find_duplicates which accepts one list as a parameter. This function needs to sort through the list passed to it and find values that are duplicated in the list. The duplicated values should be compiled into another list which the function will return. No matter how many times a word is duplicated, it should only be added once to the duplicates list. NB: Only write the function. Do not call it. For example: Test Result random_words = ("remember","snakes","nappy","rough","dusty","judicious","brainy","shop","light","straw","quickest", "adventurous","yielding","grandiose","replace","fat","wipe","happy","brainy","shop","light","straw", "quickest","adventurous","yielding","grandiose","motion","gaudy","precede","medical","park","flowers", "noiseless","blade","hanging","whistle","event","slip") print(find_duplicates(sorted(random_words))) ['adventurous', 'brainy', 'grandiose', 'light', 'quickest', 'shop', 'straw', 'yielding']…arrow_forwardWrite a function called get_palindromes() that takes a list of words as input. The function should return a new list containing every word in the input list that is a palindrome (a palindrome is a word that reads the same forwards and backwards, like "noon" or "eve"). The output list should be a list of tuples, where each tuple consists of a palindrome and its length. NOTE: You must use a list comprehension to solve this exercise. Your function must be defined as a *single line* of code - CodeRunner will count the number of "new lines" inside your function definition, and there must be only one new line (separating the function header from the function body). The code editor below should show only two lines: one for the function header and one for the function body. Delete any blank lines within the function definition and do not add a new line at the end of the function body (i.e. at the end of the return statement)! For example: Test words = ["noon", "bob", "ann"] result =…arrow_forwardIn Python IDLE: How would I write a function for the problem in the attached image?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
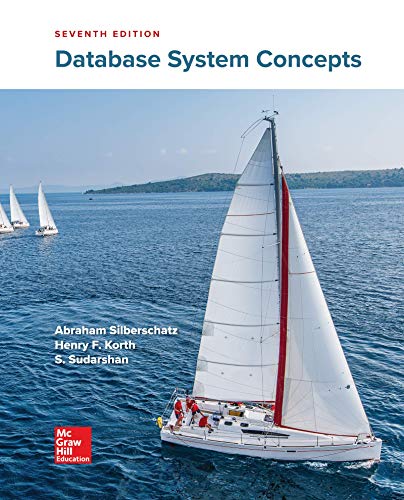
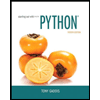
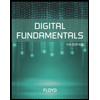
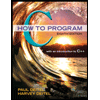
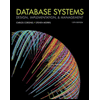
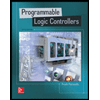