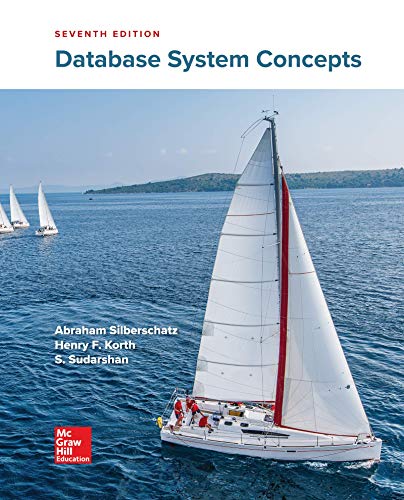
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Write a function that takes as input two sorted arrays, A and B. Assume array A has enough space at the end to hold array B. You may want to assert in your code that: 1) both input arrays A and B are sorted and 2) A has enough space at the end to hold B. Write the function code to merge B into A in sorted order. This is the function API:
void merge(int[] a, int[] b, int lastA, int lastB) {
SAVE
AI-Generated Solution
info
AI-generated content may present inaccurate or offensive content that does not represent bartleby’s views.
Unlock instant AI solutions
Tap the button
to generate a solution
to generate a solution
Click the button to generate
a solution
a solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write the function divideArray() in script.js that has a single numbers parameter containing an array of integers. The function should divide numbers into two arrays, evenNums for even numbers and oddNums for odd numbers. Then the function should sort the two arrays and output the array values to the console. Ex: The function call: let nums = [4, 2, 9, 1, 8]; divideArray(nums); produces the console output: Even numbers: 2 4 8 Odd numbers: 1 9 The program should output "None" if no even numbers exist or no odd numbers exist. Ex: The function call: let nums = [4, 2, 8]; divideArray(nums); produces the console output: Even numbers: 2 4 8 Odd numbers: None Hints: Use the push() method to add numbers to the evenNums and oddNums arrays. Supply the array sort() method a comparison function for sorting numbers correctly. To test your code in your web browser, call divideArray() from the JavaScript console.arrow_forward9. Write function getMoveRow to do the following a. Return type integer b. Parameter list i. 1-d character array (i.e. move), size 3 c. Convert the row portion of the player’s move to the associated integer index for the board array d. Example: i. move = ‘b2’ ii. 2 is the row iii. 2 is index 1 in the board array e. Return the row array index that corresponds to the player’s move f. Return a -1 if the row is not valid (i.e. INVALID) 10. Write function getMoveCol to do the following a. Return type integer b. Parameter list i. 1-d character array (i.e. move), size 3 c. Convert the column portion of the player’s move to the associated integer index for the board array d. Example: i. move = ‘b2’ ii. b is the column iii. b is index 1 in the board array e. Return the column array index that corresponds to the player’s move f. Return a -1 if the column is not valid (i.e. INVALID) I am getting an error when entering the code at the very end. please fix it. This is the code in C int…arrow_forwardIn C++, Can you please look at the code below and revise/fix so it will work according to instructions and criteria. Instruction 1) Write a function that copies a 1D array to a 2D array. The function’s prototype is bool copy1DTo2D(int d1[], int size, int d2[][NCOLS], int nrows); where size > 0 NCOLS > 0 nrows > 0 NCOLS is a global constant size = NCOLS * nrows the function returns true if the parameters and constants satisfy these conditions and false otherwise. the relation between 1d array indices and 2d array indices is 2d row index = 1d array index / NCOLS 2d column index = 1d array index modulus operator NCOLS 2) Write a function that copies a 2D array to a 1D array. The function’s prototype is bool copy2DTo1D(int d2[][NCOLS, int nrows, int d1[], int size); where size > 0 NCOLS > 0 nrows > 0 NCOLS is a global constant the function return true if the parameters and constants satisfy these conditions and false otherwise. the relation between 1d array indices and…arrow_forward
- Write a function that accepts (but does not read) a 2D array of marks and length (the number of students). The function returns a one-dimensional array with the total marks of the students and the class average. Write the declarations, and show the call to the function from function main. Assume all input and output take place in function main, not in this function, and you do not have to write the code to read in the data. Show the main program and the function call. const int NUM_STUDENTS = 35; const int NUM_ASG = 5; //Purpose: To calculate the total marks for 35 students and the class average //PreCondition: marks is a 2D array already filled with 5 marks for each student // length is the actual number of students with marks //Post Condition: total is a 1D array which is the total of the 5 marks for each student avg is the class average of the total array (for length number of students) void TotalAverage(const double marks[][NUM_ASG], int length,…arrow_forwarda. Problem 1. Create an array of 30 random numbers that range between 1 and 100. Then, write a function that will receive a number from the user and determine if that number exists in the array or not. For instance, assume the array is: [2, 93, 14, 89, 12, 3, 81, 15, 14, 89, 52, 96, 71, 82, 5, 2, 41, 23, 52, 59, 44, 44, 88, 39, 49, 50, 97, 45, 48, 36] Now, assume the user enters 89, the program should output true. But, if the user enters 77, the program should output false. Approach: We will be implementing this method in two different ways. Both will be recursive. First, implement a method called findA (x,A), where x is the number we are looking for and A is an array. In the body of the function, compare x with the FIRST item that is in the array. If this first item is equal to X, return true. If not, remove the first item from A and call findA (x,A) on the revised list. If you call find on an empty list, you will want to return false. Writing any explicit loop in your code results a…arrow_forwardWrite a function that accepts (but does not read) a 2D array of marks and length (the number of students). The function returns a one-dimensional array with the total marks of the students and the class average. Write the declarations, and show the call to the function from function main. Assume all input and output take place in function main, not in this function, and you do not have to write the code to read in the data. Show the main program and the function call. const int NUM_STUDENTS = 35; const int NUM_ASG = 5; //Purpose: To calculate the total marks for 35 students and the class average //PreCondition: marks is a 2D array already filled with 5 marks for each student // length is the actual number of students with marks //Post Condition: total is a 1D array which is the total of the 5 marks for each student avg is the class average of the total array (for length number of students) void TotalAverage(const double marks[][NUM_ASG], int length,…arrow_forward
- 2. Fill in the for-loop below with the correct parameters to make room for the new value. saved Let's say that you have to write a function to insert an element into an ordered array. It takes an array A, a size, an index and a value. The value needs to be inserted at Aſindex] without losing values that are already in the array. // Assume that the index is found to be within array bounds and there is room to insert. for (int i = ?; ?; ?) { A[i]=A[i-1]; } A[index]=value; size++; O 0; i 0; - O size; i> index; -- O size-1; i>= index; i--arrow_forwardWrite a void function that reads a file “a:data.txt” with up to 35 student ids, names and final marks into 3 arrays. When the student name is "quit" stop processing or if 35 array elements have been read then stop processing . The function should return the length of the actual number of students as well as the 3 arrays. In main, show all declarations and show the actual call. You can use the following prototype and data file: (note that in this case length would be 4) const int SIZE = 35; void ReadData( string name[],int id[], int mark[], int& length); data.txt Bob 123456 89 Suki 234567 77 Felix 345678 55 Julie 456789 67 quit in c++ please use basic coding I'm not advancedarrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
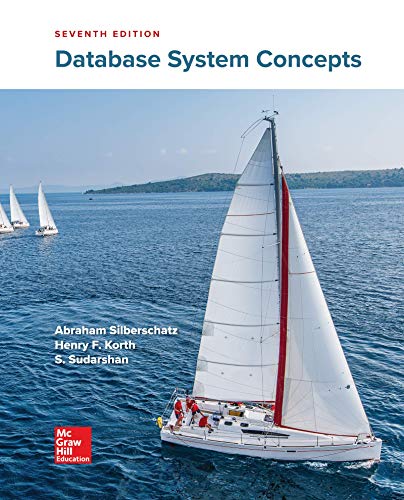
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
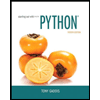
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
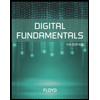
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
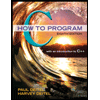
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
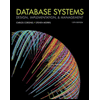
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
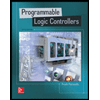
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education