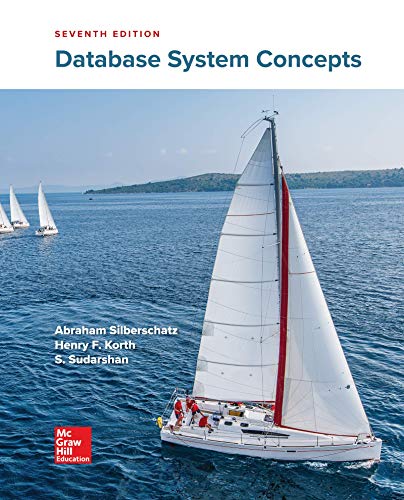
Using the provided MinHeap implementation & helper functions, build two sorted arrays as follows:
Option 1:
Given an unsorted array, build a sorted array in ascending order utilizing a minheap.
Hint: this one is quite easy.. Load a minheap with your unsorted array. From there you can get the min element from the minheap & build your sorted array.
Option 2:
Given your unsorted array loaded into a maxheap..
Using this max-heap, in which the root node contains the largest element, build a sorted array in ascending order. To achieve this you’ll ‘Float’ the max to the front and build the sorted list from the back.
The basic idea is to sort in place.
- Every time the
- Hence, the next place at the end of the array is not part of the heap anymore.
- At this index the n-th smallest number is placed.
Final Solution should look like:
Array to be sorted by MinHeap: [5, 3, 17, 10, 84, 19, 6, 22, 9, 7, 28]
Min Heap Sorted array: [3, 5, 6, 7, 9, 10, 17, 19, 22, 28, 84]
Array to be sorted by native Heapify: [5, 3, 17, 10, 84, 19, 6, 22, 9, 7, 28]
Heapify sorted array: [3, 5, 6, 7, 9, 10, 17, 19, 22, 28, 84]
package keeper;
class MinHeap {
private int[] Heap;
private int size;
private int maxsize;
// Initializing front
private static final int FRONT = 1;
// Ctor public MinHeap(int maxsize)
{
// This keyword refers to current object itself
this.maxsize = maxsize;
this.size = 0;
Heap = new int[this.maxsize + 1];
Heap[0] = Integer.MIN_VALUE; }
// Returning the position of
// the parent for the node currently
// at pos
private int parent(int pos) {
return pos / 2; }
// Returning the position of the
// left child for the node currently at pos
private int leftChild(int pos) {
return (2 * pos); }
// Returning the position of
// the right child for the node currently
// at pos
private int rightChild(int pos) {
return (2 * pos) + 1;
}
// Returning true if the passed
// node is a leaf node
private boolean isLeaf(int pos) {
if (pos > (size / 2.0)) {
return true;
}
return false;
}
// swap two nodes of the heap
private void swap(int fpos, int spos) {
int tmp;
tmp = Heap[fpos];
Heap[fpos] = Heap[spos];
Heap[spos] = tmp;
}
// heapify the node at pos. recursive call.
private void minHeapify(int pos) {
if (!isLeaf(pos)) {
int swapPos = pos;
// swap with the minimum of the two children to check if right child exists.
// Otherwise default value will be '0' and that will be swapped with parent node.
if (rightChild(pos) <= size)
swapPos = Heap[leftChild(pos)] < Heap[rightChild(pos)] ? leftChild(pos) : rightChild(pos);
else
swapPos = Heap[leftChild(pos)];
if (Heap[pos] > Heap[leftChild(pos)] || Heap[pos] > Heap[rightChild(pos)]) {
swap(pos, swapPos);
minHeapify(swapPos);
}
}
}
//insert a node into the heap public void insert(int element) {
if (size >= maxsize) { return;
} Heap[++size] = element;
int current = size;
while (Heap[current] < Heap[parent(current)]) {
swap(current, parent(current));
current = parent(current);
}
}
// To print the contents of the heap
public void print() {
for (int i = 1; i <= size / 2; i++) {
// Printing the parent and both children
System.out.print(
" PARENT : " + Heap[i] + " LEFT CHILD : " + Heap[2 * i] + " RIGHT CHILD :" + Heap[2 * i + 1]);
// formating
System.out.println();
}
}
// Remove and return the minimum
// element from the heap public int remove() {
int popped = Heap[FRONT];
Heap[FRONT] = Heap[size--];
minHeapify(FRONT);
return popped; }
// driver
public static void main(String[] arg) {
// Display message
System.out.println("The Min Heap is ");
// Creating object of class in main() method
MinHeap minHeap = new MinHeap(29);
// Inserting element to minHeap
// using insert() method
// Custom input entries
minHeap.insert(5);
minHeap.insert(3);
minHeap.insert(17);
minHeap.insert(10);
minHeap.insert(84);
minHeap.insert(19);
minHeap.insert(6);
minHeap.insert(22);
minHeap.insert(9);
minHeap.insert(7);
minHeap.insert(28);
// Print all elements of the heap
minHeap.print();
// Removing minimum value from above heap
// and printing it
System.out.println("The Min val is " + minHeap.remove());
System.out.println("The Min val is " + minHeap.remove());
System.out.println("The Min val is " + minHeap.remove());
System.out.println("The Min val is " + minHeap.remove());
System.out.println("The Min val is " + minHeap.remove());
System.out.println("The Min val is " + minHeap.remove());
System.out.println("The Min val is " + minHeap.remove());
System.out.println("The Min val is " + minHeap.remove());
System.out.println("The Min val is " + minHeap.remove());
System.out.println("The Min val is " + minHeap.remove());
System.out.println("The Min val is " + minHeap.remove());
}
}
Package keeper;
import java.util.Arrays;
public class heap HW{
public static void main (String args[]) {
int arr[] = {5, 3, 17, 10, 84, 19, 6, 22, 9, 7, 28 };
System.out.println("Array to be sorted by MinHeap: " + Arrays.toString(arr));
heapSortByMinHeap(arr);
System.out.println("");
System.out.println("Array to be sorted by native Heapify: " + Arrays.toString(arr));
heapSortByHeapify(arr);
}

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- Hello! I need some help with my Java homework. Please use Eclipse Please add comments to the to program so I can understand what the code is doing and learn Create a new Eclipse project named so as to include your name (eg smith15 or jones15). In this project, create a new package with the same name as the project. In this package, write a solution to the exercise noted below. Implement the following method that returns the maximum element in an array: public static <E extends Comparable<E>> E max(E[] list) Write a test program that generates 10 random integers, invokes this method to find the max, and then displays the random integers sorted smallest to largest and then prints the value returned from the method. Max sure the the last sorted and returned value as the same!arrow_forwardYou are working for a university to maintain a list of grades and some related statistics for a student. Class and Data members: Create a class called Student that stores a student’s grades (integers) in a vector (do not use an array). The class should have data members that store a student’s name and the course for which the grades are earned. Constructor(s): The class should have a 2-argument constructor that receives the student’s name and course as parameters and sets the appropriate data members to these values. Member Functions: The class should have functions as follows: Member functions to set and get the student’s name and course variables. A member function that adds a single grade to the vector. Only positive grades are allowed. Call this function AddGrade. A member function to sort the vector in ascending order. A member function to compute the average (x̄) of the grades in the vector. The formula for calculating an average is x̄ = ∑xi / n where xi is the value of each…arrow_forwardIn this assignment, you will decide how to keep the inventory in the text file. Then your program must read the inventory from the file into the array. Each product must have a record in the file with the name, regular price, and type. There are several options for storing records in the file. For example, • each value takes one line in the file (i.e., three lines for one product). Then you must take care of correct handling of the ends of the lines; • all values are in one line that can be read as a string. Then you must handle the parsing of the string; • all values are in one line separated by a delimiter. Then you must handle a line with delimiters. Assume that the inventory does not have more than 100 products. But the actual number is known only after the reading of the file. Once you can read data from the file into the array, you must add a new property to the product class – a static variable that holds the number of products in the inventory. Its value must grow as reading…arrow_forward
- In cell C18 type a VLOOKUP function to find the corresponding letter grade (from column D) for the name in A18. The table array parameter is the same as in B18. Type FALSE for the range lookup parameter. Copy the formula in C18 to C19:C22Notice this formula works correctly for all cells. Range lookup of FALSE means do an exact match on the lookup value whether or not the table array is sorted by its first column. In cell E11, type an IF function that compares the score in B11 with the minimum score to pass in A5. If the comparison value is true, display Pass. Otherwise, display Fail. Copy the formula in E11 to E12:E15. Did you use appropriate absolute and relative references so the formula copied properly? In cell F11, type an IF function that compares the grade in D11 with the letter F (type F). If these two are not equal, display Pass. Otherwise, display Fail. Copy the formula in F11 to F12:F15arrow_forwardGive me correct code. Downvote for incorrect and copied code. Changes in function..arrow_forwardStep 1: Read your files! You should now have 3 files. Read each of these files, in the order listed below. staticarray.h -- contains the class definition for StaticArray, which makes arrays behave a little more like Python lists O In particular, pay attention to the private variables. Note that the array has been defined with a MAX capacity but that is not the same as having MAX elements. Which variable indicates the actual number of elements in the array? staticarray.cpp -- contains function definitions for StaticArray. Right now, that's just the print() member function. 0 Pay attention to the print() function. How many elements is it printing? main.cpp -- client code to test your staticarray class. Note that the multi-line comment format (/* ... */) has been used to comment out everything in main below the first print statement. As you proceed through the lab, you will need to move the starting comment (/*) to a different location. Other than that, no changes ever need to be made to…arrow_forward
- What is the best way to implement a dynamic array if you don't know how many elements will be added? Create a new array with double the size any time an add operation would exceed the current array size. Create a new array with 1000 more elements any time an add operator would exceed the current size. Create an initial array with a size that uses all available memory Block (or throw an exception for) an add operation that would exceed the current maximum array size.arrow_forward1Code a JavaScript callback function for the Array.map method to ('Yu Yamaguchi') evaluate an array of integersidentify the integers that are Prime numbersreturn the Prime numbers in the new array 2 Code a JavaScript callback function for the Array.reduce method to evaluate an array of integersdetermine the smalles integerreturn the smalles integer Note: if there are identical integers, the function is to return the last one.arrow_forwardQuestion 1. Write a console application with several functions that deal with a two-dimensional array of positive integers: The number of rows and number of columns of the array should be 20. Fill the array with random numbers between 1 and 1000. Search for the prime numbers in the array using the following function in your code: bool isPrime(int n) for (int i = 2; iarrow_forward
- 4. Arrays. In order to test the functions in this question, you will need an array. We can create a three- dimensional test array as follows: testArray xijk - máx rij,k i=1 i=1 i=1 (b) Now suppose we want a function testFn2 which returns the d2 x d3 matrix {zj.k} where di Zj,k = Σ i=1arrow_forwarduse the array built to shuffle and deal two poker hands. Change the corresponding values for all Jacks through Aces to 11, 12, 13, 14 respectively. Shuffle the deck, then deal two hands. Comment your code and submit the Python code. i wrote a code for it but its not displaying properly, can someone tell me why? (black and white is the array given/ the picture of the python app is what i wrote) its only displaying the array given and not the bottom code i put, why?arrow_forwardPleas help! Thank you.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
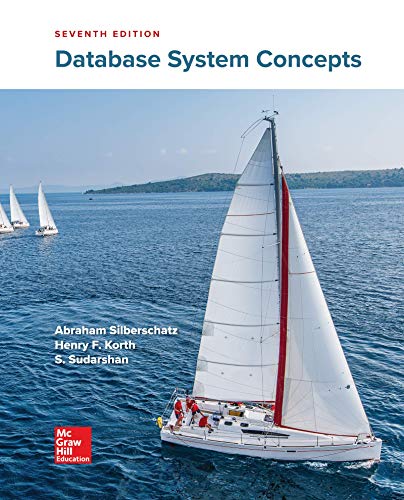
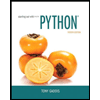
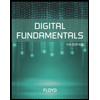
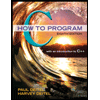
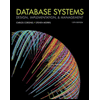
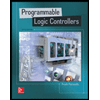