Write a GUI-based program that allows the user to convert temperature values between degrees Fahrenheit and degrees Celsius. The interface should have labeled entry fields for these two values.
Write a GUI-based program that allows the user to convert temperature values between degrees Fahrenheit and degrees Celsius. The interface should have labeled entry fields for these two values.
Chapter16: Graphics
Section: Chapter Questions
Problem 8PE
Related questions
Question
Write a GUI-based program that allows the user to convert temperature values between degrees Fahrenheit and degrees Celsius. The interface should have labeled entry fields for these two values.
- These components should be arranged in a grid where the labels occupy the first row and the corresponding fields occupy the second row.
- At start-up, the Fahrenheit field should contain 32.0, and the Celsius field should contain 0.0.
- The third row in the window contains two command buttons, labeled >>>> and <<<<.
- When the user presses the first button, the program should use the data in the Celsius field to compute the Fahrenheit value, which should then be output to the Fahrenheit field.
- The second button should perform the inverse function.
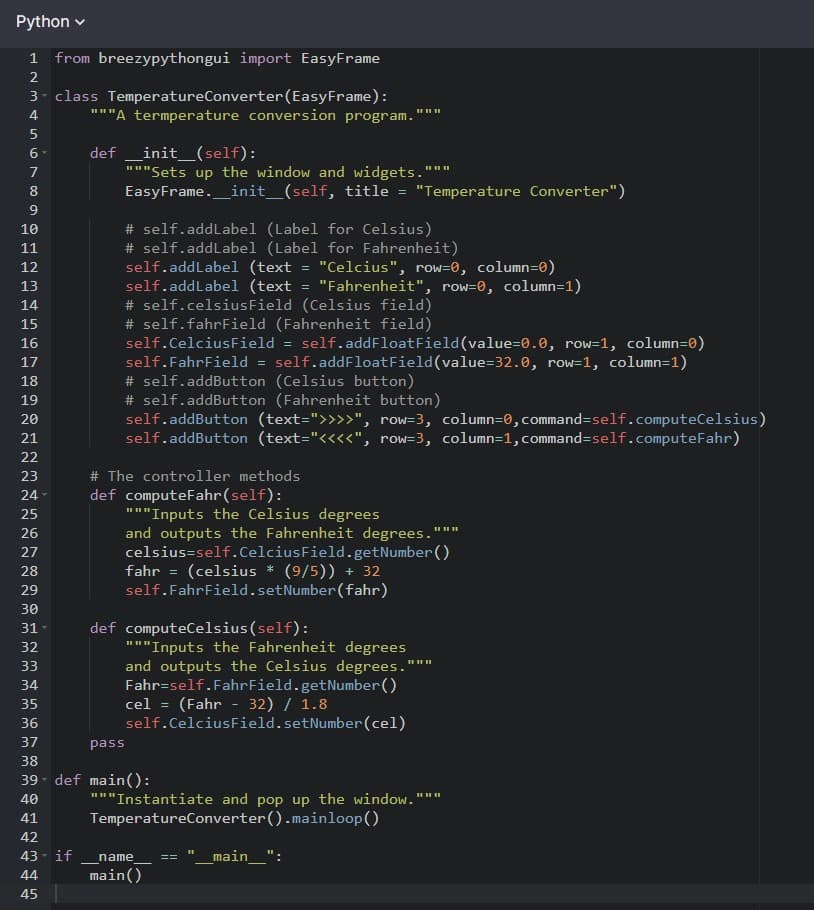
Transcribed Image Text:Python v
1 from breezypythongui import EasyFrame
2
3 class TemperatureConverter(EasyFrame):
"""A termperature conversion program.
4
5
init_(self):
"""Sets up the window and widgets.
EasyFrame._init_(self, title = "Temperature Converter")
def
II II II
9.
# self.addLabel (Label for Celsius)
# self.addLabel (Label for Fahrenheit)
self.addLabel (text
self.addLabel (text
# self.celsiusField (Celsius field)
# self.fahrField (Fahrenheit field)
10
11
"Celcius", row=0, column=0)
"Fahrenheit", row=0, column=1)
12
13
14
15
self.addFloatField(value=0.e, row=1, column=0)
self.addFloatField(value=32.0, row=1, column=1)
16
self.CelciusField
17
self.FahrField =
# self.addButton (Celsius button)
# self.addButton (Fahrenheit button)
self.addButton (text=">>>>", row=3, column=0, command=self.computeCelsius)
self.addButton (text="<<<<", row=3, column=1, command=self.computeFahr)
18
19
20
21
22
23
# The controller methods
def computeFahr(self):
"""Inputs the Celsius degrees
and outputs the Fahrenheit degrees.
celsius=self.CelciusField.getNumber()
fahr = (celsius * (9/5)) + 32
self.FahrField.setNumber (fahr)
24
II I
25
26
27
28
29
30
def computeCelsius(self):
"""Inputs the Fahrenheit degrees
and outputs the Celsius degrees.'
Fahr=self.FahrField.getNumber()
cel = (Fahr - 32) / 1.8
self.CelciusField.setNumber(cel)
31
32
33
34
35
36
37
pass
38
39 def main():
II II
"""Instantiate and pop up the window.""
TemperatureConverter().mainloop()
40
41
42
43 - if
main_'
name
44
main()
45
6700
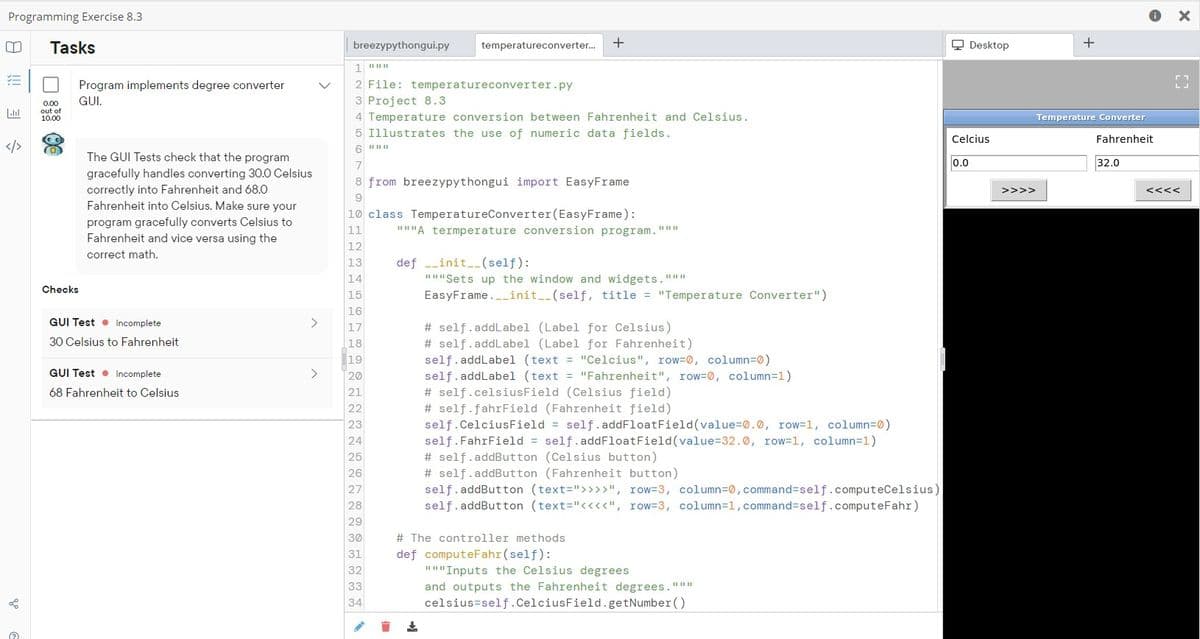
Transcribed Image Text:Programming Exercise 8.3
Tasks
breezypythongui.py
temperatureconverter.
Q Desktop
2 File: temperatureconverter.py
Program implements degree converter
GUI.
3 Project 8.3
0.00
out of
10.00
4 Temperature conversion between Fahrenheit and Celsius.
Temperature Converter
5 Illustrates the use of numeric data fields.
Celcius
Fahrenheit
</>
(0)
The GUI Tests check that the program
0.0
32.0
gracefully handles converting 30.0 Celsius
correctly into Fahrenheit and 68.0
Fahrenheit into Celsius. Make sure your
program gracefully converts Celsius to
Fahrenheit and vice versa using the
8 from breezypythongui import EasyFrame
>>>>
<<<<
9
10 class TemperatureConverter(EasyFrame):
" "A termperature conversion program."
11
12
correct math.
13
def --init_(self):
"I"Sets up the window and widgets."""
EasyFrame._init__(self, title = "Temperature Converter")
14
Checks
15
16
GUI Test • Incomplete
# self.addLabel (Label for Celsius)
# self.addLabel (Label for Fahrenheit)
self.addLabel (text = "Celcius", row=0, column=0)
self.addLabel (text = "Fahrenheit", row=0, column=1)
# self.celsiusField (Celsius field)
# self.fahrField (Fahrenheit field)
self.CelciusField = self. addFloatField(value=0.0, row=1, column=0)
self.FahrField = self.addFloatField(value=32.0, row=1, column=1)
# self.addButton (Celsius button)
# self.addButton (Fahrenheit button)
self. addButton (text=">>>>", row=3, column=0,command=self.computeCelsius)
self.addButton (text="<<<<", row=3, column=1, command3self.computeFahr)
17
30 Celsius to Fahrenheit
18
19
GUI Test • Incomplete
20
68 Fahrenheit to Celsius
21
22
23
24
25
26
27
28
29
30
# The controller methods
def computeFahr (self):
II I"Inputs the Celsius degrees
31
32
33
and outputs the Fahrenheit degrees."""
34
celsius=self.CelciusField.getNumber ()
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
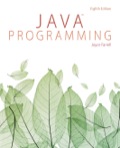
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
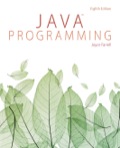
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT