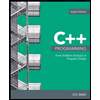
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN: 9781337102087
Author: D. S. Malik
Publisher: Cengage Learning
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Java
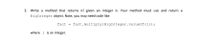
Transcribed Image Text:**Factorial Method Using BigInteger in Java**
**Objective:**
Develop a method to calculate the factorial of a number `n` using the `BigInteger` class to handle large numbers.
**Instructions:**
1. **Method Requirement:**
- Create a method that computes `n!`, where `n` is an integer.
- The method should utilize and return a `BigInteger` object.
2. **Implementation Guidance:**
- To handle multiplication for large numbers, use:
```java
fact = fact.multiply(BigInteger.valueOf(i));
```
In this code snippet, `fact` is a `BigInteger` object, and `i` represents an integer in the calculation process.
**Note:** The `BigInteger` class in Java allows operations on arbitrarily large integers, making it ideal for calculations that exceed the range of standard primitive data types like `int` or `long`.

Transcribed Image Text:Each of the following methods should be `static` and defined in a class called `Utilities`. Implement a second class called `Tester` that calls each of these methods.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a program named SalespersonDemo that instantiates objects using classes named Real EstateSalesperson and GirlScout. Demonstrate that each object can use a SalesSpeech() method appropriately. Also, use a MakeSale() method two or three times with each object, and display the final contents of each objects data fields. First, create an abstract class named Salesperson. Fields include first and last names; the Salesperson constructor requires both these values. Include properties for the fields. Include a method that returns a string that holds the Salespersons full name—the first and last names separated by a space. Then perform the following Create two child classes of Salesperson: Real EstateSalesperson and Girl Scout. The Real EstateSalesperson class contains fields for total value sold in dollars and total commission earned (both of which are initialized to 0), and a commission rate field required by the class constructor. The Girl Scout class includes a field to hold the number of boxes of cookies sold, which is initialized to 0. Include properties for every field. Create an interface named ISell able that contains two methods: SalesSpeech() and MakeSale(). In each Real EstateSalesperson and Girl Scout class, implement SalesSpeech() to display an appropriate one- or two-sentence sales speech that the objects of the class could use. In the Real Estatesalesperson class, implement the MakeSale() method to accept an integer dollar value for a house, add the value to the Real EstateSalespersons total value sold, and compute the total commission earned. In the Girl Scout class, implement the MakeSale() method to accept an integer representing the number of boxes of cookies sold and add it to the total field.arrow_forwardUsing classes, design an online address book to keep track of the names, addresses, phone numbers, and dates of birth of family members, close friends, and certain business associates. Your program should be able to handle a maximum of 500 entries. Define a class addressType that can store a street address, city, state, and ZIP code. Use the appropriate functions to print and store the address. Also, use constructors to automatically initialize the member variables. Define a class extPersonType using the class personType (as defined in Example 10-10, Chapter 10), the class dateType (as designed in this chapters Programming Exercise 2), and the class addressType. Add a member variable to this class to classify the person as a family member, friend, or business associate. Also, add a member variable to store the phone number. Add (or override) the functions to print and store the appropriate information. Use constructors to automatically initialize the member variables. Define the class addressBookType using the previously defined classes. An object of the type addressBookType should be able to process a maximum of 500 entries. The program should perform the following operations: Load the data into the address book from a disk. Sort the address book by last name. Search for a person by last name. Print the address, phone number, and date of birth (if it exists) of a given person. Print the names of the people whose birthdays are in a given month. Print the names of all the people between two last names. Depending on the users request, print the names of all family members, friends, or business associates.arrow_forwardChapter 10 defined the class circleType to implement the basic properties of a circle. (Add the function print to this class to output the radius, area, and circumference of a circle.) Now every cylinder has a base and height, where the base is a circle. Design a class cylinderType that can capture the properties of a cylinder and perform the usual operations on the cylinder. Derive this class from the class circleType designed in Chapter 10. Some of the operations that can be performed on a cylinder are as follows: calculate and print the volume, calculate and print the surface area, set the height, set the radius of the base, and set the center of the base. Also, write a program to test various operations on a cylinder.arrow_forward
- At most, a class can contain ____________ method(S). 0 1 2 any number ofarrow_forwarda. Write a FractionDemo program that instantiates several Fraction objects and demonstrates that their methods work correctly. Create a Fraction class with fields that hold a whole number, a numerator, and a denominator. In addition: Create properties for each field. The set access or for the denominator should not allow a 0 value; the value defaults to 1. Add three constructors. One takes three parameters for a whole number, numerator, and denominator. Another accepts two parameters for the numerator and denominator; when this constructor is used, the whole number value is 0. The last constructor is parameterless; it sets the whole number and numerator to 0 and the denominator to 1. (After construction, Fractions do not have to be reduced to proper form. For example, even though 3/9 could be reduced to 1/3, your constructors do not have to perform this task.) Add a Reduce() method that reduces a Fraction if it is in improper form. For example, 2/4 should be reduced to 1/2. Add an operator+() method that adds two Fractions. To add two fractions, first eliminate any whole number part of the value. For example, 2 1/4 becomes 9/4 and 1 3/5 becomes 8/5. Find a common denominator and convert the fractions to it. For example, when adding 9/4 and 8/5, you can convert them to 45/20 and 32/20. Then you can add the numerators, giving 77/20. Finally, call the Reduce() method to reduce the result, restoring any whole number value so the fractional part of the number is less than 1. For example, 77/20 becomes 3 17/20. Include a function that returns a string that contains a Fraction in the usual display format—the whole number, a space, the numerator, a slash (D, and a denominator. When the whole number is 0, just the Fraction part of the value should be displayed (for example, 1/2 instead of 0 1/2). If the numerator is 0, just the whole number should be displayed (for example, 2 instead of 2 0/3). b. Add an operator*() method to the Fraction class created in Exercise 11a so that it correctly multiplies two Fractions. The result should be in proper, reduced format. Demonstrate that the method works correctly in a program named FractionDemo2. c. Write a program named FractionDem03 that includes an array of four Fractions. Prompt the user for values for each. Display every possible combination of addition results and every possible combination of multiplication results for each Fraction pair (that is, each type will have 16 results).arrow_forwardCreate an application for Ninas Cookie Emporium named CookieDemo that declares and demonstrates objects of the CookieOrder class and its descendants. The CookieOrder class includes auto-implemented properties for an order number, recipients name, and cookie type (for example, chocolate chip), and fields for number of dozens ordered and price. When the field value for number of dozens ordered is set, the price field is set as $15 per dozen for the first two dozen and $13 per dozen for each dozen over two. Create a child class named Special Cookieorder, which includes a field with a description as to why the order is special (for example, gluten-free). Override the method that sets a CookieOrders price as described in part a, but also to include special handling, which is SIO for orders up to $40 and $8 for higher-priced orders. Create an application named CookieDem02 that demonstrates using several Special CookieOrder objects.arrow_forward
- Mark the following statements as true or false. The member variables of a class must be of the same type. (1) The member functions of a class must be public. (2) A class can have more than one constructor. (5) A class can have more than one destructor. (5) Both constructors and destructors can have parameters. (5)arrow_forwardA(n) ____________ is one instance or variable of a class.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
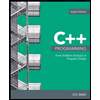
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
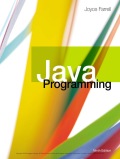
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
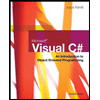
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
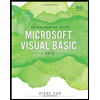
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
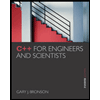
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr