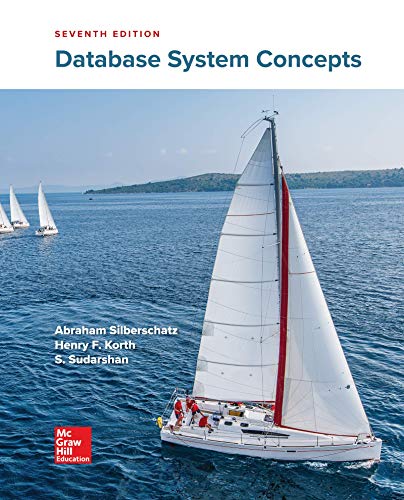
Write a program IN JAVA that replaces every “Java” word in a file into the word “HTML” and saves
the file in the same location on disk after printing both the old lines and new lines to the
output. The input file is passed to the program as a command line argument.
Sample file: input.txt
Welcome to Java!
This is a Java program.
I like
Use the following statements to create a file instance and make a scanner for it:
// Create a File instance
java.io.File file = new java.io.File("inputFileName.txt");
// Create a Scanner for the file
Scanner input = new Scanner(file);
// Read data from a file using input, e.g. input.next()
// input.hasNext() determines if there's more data in the file
Use the following statements to write to a file:
// Create a File instance
java.io.File file = new java.io.File("outputFileName.txt");
// Create the file
java.io.PrintWriter output = new java.io.PrintWriter(file);
// Write output to the file, this can be repeated
output.print(someString);
// Close the file
output.close();

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

- The next three questions concern a program that opens a scores file provided by the user, reads it one line at a time, adds the scores as floating point numbers to a list scores, and then computes the average score. The example file scores.csv looks like this: Student Name, Test Score Jinyue, 98.5 Adesh, 97.4 Pinn, 99.1 Arindaam, 97.2 The first part of the program opens the file and reads just the first line into the variable header. # Open the Filename and ignore the first line filename= input("Enter filename:") open (filename) fhand = header = Fill in the blank to complete the program fragment:arrow_forwardWrite a java program that Reads text from the user provided file using FileReader Writes output to a file using the FileWriter class Catches IOException using try and catch block and prints a customized error message.arrow_forwardIN JAVA Which XXX allows information to be written to a file using print( )? FileOutputStream foStream = null; PrintWriter outFS = null; foStream = new FileOutputStream("outfile.txt");XXXoutFS.println("Java is my favorite language!"); A. outFS = new PrintWriter("outfile.txt"); B. new PrintWriter(foStream); C. outFS = PrintWriter.open(foStream); D. outFS = new PrintWriter(foStream);arrow_forward
- Write a Java program that reads from a URL and searches for a given word in the URL and creates a statistic file as an output. The statistic file needs to include some information from the URL. URL address Number of words in the URL page Number of repetitions for a given word displays the number of times the word appears. You need to have two functions, one for reading from the URL and the other function for searching the word.arrow_forwardin java Integer cradleQuantity and string friendName are read from input. A FileOutputStream named fileStream is declared and the file named cradle.txt is opened. Then, a PrintWriter named cradleWriter is declared and associated with the file. Write the following to the opened file: "Remember:" "* * * *" cradleQuantity, followed by " cradles for " and friendName Another "* * * *" End each output with a newline. Finally, close the file. Ex: If the input is 12 Dax, then cradle.txt contains: Remember: * * * * 12 cradles for Dax * * * * Note: Data written to a file may be lost if the file is not closed. 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 public class FileOutput { publicstaticvoidmain(String[] args) throwsIOException { Scannerscnr=newScanner(System.in); FileOutputStreamfileStream=null; PrintWritercradleWriter=null; intcradleQuantity; StringfriendName; cradleQuantity=scnr.nextInt(); friendName=scnr.next();…arrow_forwardJava Your program must read a file called personin.txt. Each line of the file will be a person's name, the time they arrived at the professor's office, and the amount of time they want to meet with the professor. These entries will be sorted by the time the person arrived. Your program must then print out a schedule for the day, printing each person's arrival, and printing when each person goes in to meet with the professor. You need to print the events in order of the time they happen. In other words, your output will be sorted by the arrival times and the times the person goes into the professor's office. In your output you need to print out a schedule. In the schedule, new students go to the end of the line. Whenever the professor is free, the professor will either meet with the first person in line, or meet with the first person in line if nobody is waiting. Assume no two people arrive at the same time. You should solve this problem using a stack and a queue. You can only…arrow_forward
- I need help with my code i need to ask the user for a filename. Display the oldest car for every manufacturer from that file. If two cars have the same year, compare based on the VIN. my code is not working properly, it is just dipslaying exactly what is in the file that the user inputs here is the code import java.io.BufferedReader;import java.io.FileReader;import java.util.Comparator;import java.io.File;import java.io.FileNotFoundException;import java.util.ArrayList;import java.util.Collections;import java.util.Scanner;class Car {private String makeModel;private int year;private String VIN;public Car(String makeModel, int year, String VIN) {this.makeModel = makeModel;this.year = year;this.VIN = VIN;}public String getMakeModel() {return makeModel;}public int getYear() {return year;}public String getVIN() {return VIN;}}class Assignment8_2 {private static int minIndex(ArrayList<Car> cars, int i, int j) {if (i == j)return i;int k = minIndex(cars, i + 1, j);if…arrow_forwardI am trying to figure out why the sum at the end of my Java code is not working correctly. It keeps showing as zero and I do not know what the issue is. Here is the program requirements Write a program in a single file that: Main: Creates 10 random doubles, all between 1 and 11, Calls a method that writes 10 random doubles to a text file, one number per line. Calls a method that reads the text file and displays all the doubles and their sum accurate to two decimal places. SAMPLE OUTPUT 10.62691196041722.7377903389094555.4279257388651281.37420580654725091.18587002624988364.1803912764852284.9109699989306755.7108582343439587.7908570073730523.1806714736219543 The total is 47.13 Here is my code: import java.util.*;import java.io.*; public class AssignmentTwo { public static void main(String[] args) {//main method double randNum [] = new double [10]; for(int i = 0; i< randNum.length; i++) { randNum[i] = (double)(Math.random()* (10) + 1);…arrow_forwardWrite a Java program to perform the following tasks:The program should ask the user for the name of an input file (scores.txt). Theinput file should contain 10 records. Each record in the input file shouldconsist of a name and score between 0 and 100 (inclusive). Eg: fred 95The program should open the input file as a text file (if the input file does notexist it should throw an exception) and read the contents line by line.Store each record in an array of a user-defined Score class. The Score classcan be kept very simple with 2 instance variables, a default constructor, getand set methods for the instance variables.Now process the array of Score class objects to determine:• The average of all scores• The largest score• The smallest scorearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
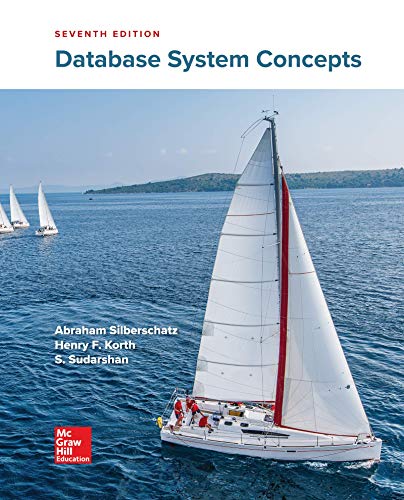
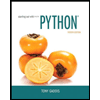
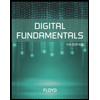
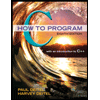
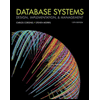
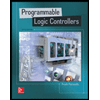