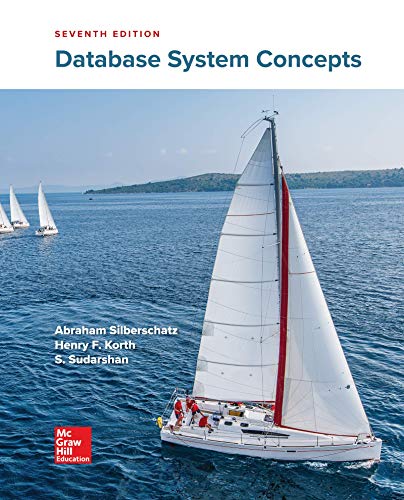
Write a
Ex: If the input is:
25 51 0 200 33 0 50
the output is:
25 0 33
The bounds are 0-50, so 51 and 200 are out of range and thus not output.
For coding simplicity, follow each output integer by a space, even the last one. Do not end with newline.
My Code:
def main():
inputs=[]
num_inputs = int(input('Enter number of inputs: '))
if num_inputs > 9:
print('Too many inputs')
else:
print("Enter", num_inputs," inputs in sorted order: ")
for i in range(num_inputs):
inputs.append(input())
print('The list of integers is: ', inputs)
middle_position=int(num_inputs/2)
print('The middle integer is: ',inputs[middle_position])
if __name__ == "__main__":
main()
This codes output is:
Traceback (most recent call last): File "main.py", line 19, in <module> main() File "main.py", line 4, in main num_inputs = int(input('Enter number of inputs: ')) ValueError: invalid literal for int() with base 10: '25 51 0 200 33'

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

- in java String inputWord is read from input. Write a while loop that iterates until inputWord is equal to "Finale". In each iteration of the loop: Read integer clothingNumber from input. If clothingNumber is less than 30, output the value of inputWord, followed by ": low on stock" and a newline. Otherwise, output the value of inputWord, followed by ": well stocked" and a newline. Read string inputWord from input. Click here for example 1 2 3 4 5 6 7 8 9 10 11 12 13 14 import java.util.Scanner; public class PairedDataProcessing { publicstaticvoidmain(String[] args) { Scannerscnr=newScanner(System.in); StringinputWord; intclothingNumber; inputWord=scnr.next(); /* Your code goes here */ } }arrow_forwardWrite an improved version of the chaos . py program from Chapter 1 thatallows a user to input two initial values and the number of iterations, and then prints a nicely formatted table showing how the values changeover time. For example, if the starting values were . 25 and . 26 with 10iterations, the table might look like this: index 0.25 0.26----------------------------1 0 . 731250 0 . 7503602 0 . 766441 0 . 7305473 0 . 698135 0 . 7677074 0 . 821896 0 . 6954995 0 . 570894 0 . 8259426 0 . 955399 0 . 5606717 0 . 166187 0 . 9606448 0 . 540418 0 . 1474479 0 . 968629 0 . 49025510 0.118509 0 . 974630arrow_forwardIn python, write a code that allows the user to input two non-negative number sequences in increasing order (the numbers entered are always getting bigger, and no number repeats), both terminated by a -1. The size of each sequence can vary (maybe sequence - 1 has four numbers, and sequence - 2 has seven). The output of this code should be a third sequence that is a combination of both sequences 1 and 2 and is sorted in non-decreasing order. Note: Non-decreasing order means there can be a repeated number in the third sequence (see example 1). A strictly increasing order sequence cannot have repeat numbers at all (see example 3). Remember, all input sequences must be in strictly increasing order! please do this using python, only using while loops. NO language of "break" or "len" please. Hint: we can use three separate lists to solve this code.arrow_forward
- Write a program that reads a list of words. Then, the program outputs those words and their frequencies (case insensitive). Ex: If the input is: hey Hi Mark hi mark the output is: hey 1 Hi 2 Mark 2 hi 2 mark 2 Hint: Use lower() to set each word to lowercase before comparing. My code: my_list = input()my_list_lower = my_list.lower()list = my_list_lower.split()for word in list: freq = list.count(word) print(word, freq)the program wants the lower case number count AND for the list of words to remain upper case if they were upper case. how do i make it do that?arrow_forwardWrite a program that takes in four positive integers and outputs the number of odd numbers. (Hint: use the modulo operator to determine if a number is odd) Ex: If the input is: 1 2 3 4 the output is: 2 Below is my code so far and I'm getting back unexpected EOF while parsing. I can't figure out what I'm doing wrong. odd_number=[]number_1=int(input())number_2=int(input())number_3=int(input())number_4=int(input())if number_1%2!=0: odd_number.append(number_1)else: passif number_2%2!=0: odd_number.append(number_2)else: passif number_3%2!=0: odd_number.append(number_3)else: passif number_4%2!=0: odd_number.append(number_4)else: passprint(len(odd_number)arrow_forwardWrite a program to understand customer waiting time. A list provides the number of customers that arrive every minute for 10 minutes. The program should output the length of the line at each minute, assuming one clerk serves each customer and takes 1 minute to complete service. If the input is 2 0 0 2 1 0 0 0 1 0, the output should be 2 1 0 2 2 1 0 0 1 0 (At minute 0, 2 customers arrived in line. At minute 1, 1 of those has been served, reducing the line to 1. At minute 2, that customer was served, reducing the line to 0). Output a space after each output integer, including the last (followed by newline). Hints: Every minute, if the lineLength wasn't 0, you can decrement lineLength because the clerk would have finished serving one customer. Every minute, if new customers arrived, just add them to lineLength for that minute.arrow_forward
- Write a program that reads a list of integers, and outputs whether the list contains all even numbers, odd numbers, or neither. The input begins with an integer indicating the number of integers that follow. Ex: If the input is: 5 2 4 6 8 10 the output is: all even Ex: If the input is: 5 1 3 5 7 9 the output is: all odd Ex: If the input is: 5 1 2 3 4 5 the output is: not even or odd Your program must define and call the following two functions. is_list_even() returns true if all integers in the list are even and false otherwise. is_list_odd() returns true if all integers in the list are odd and false otherwise.def is_list_even(my_list)def is_list_odd(my_list) if __name__ == '__main__': both def and if need to be included and functions called from both defs def is_list_even(my_list):for i in range(len(my_list)):if my_list[i] % 2 != 0:return False or Truedef is_list_odd(my_list):for i in range(len(my_list)):if my_list[i] % 2 == 0:return False or Trueif is_list_odd == True:print("all…arrow_forwardWrite a program that first gets a list of integers from input. The input begins with an integer indicating the number of integers that follow. Then, get the last value from the input, and output all integers less than or equal to that value. Ex: If the input is: 5 50 60 140 200 75 100 the output is: 50 60 75 The 5 indicates that there are five integers in the list, namely 50, 60, 140, 200, and 75. The 100 indicates that the program should output all integers less than or equal to 100, so the program outputs 50, 60, and 75. For coding simplicity, follow every output value by a space, including the last one. Such functionality is common on sites like Amazon, where a user can filter results. Write your code to define and use two functions:vector<int> GetUserValues()void OutputIntsLessThanOrEqualToThreshold(vector<int> userValues, int upperThreshold) #include <iostream>#include <vector> using namespace std; /* Define your function here */ int main() {/* Type your…arrow_forwardWrite a program that takes in an integer in the range 11-100 as input. The output is a countdown starting from the integer, and stopping when both output digits are identical. Additionally, output the distance between the starting and ending numbers (ex: 93 - 88 = 5) Note: End with a newline. Ex: If the input is: 93 the output is: 93 92 91 90 89 88 5 Ex: If the input is: 11 the output is: 11 0 Ex: If the input is: 9 or any value not between 11 and 100 (inclusive), the output is: Input must be 11-100 For coding simplicity, follow each output number by a space, even the last one. Use a while loop. Compare the digits; do not write a large if-else for all possible same-digit numbers (11, 22, 33, …, 99), as that approach would be cumbersome for larger ranges. Hint: To practice incremental development, start by writing code that just outputs the countdown until matching digits and submit to get most of the points. Then, update your code to output the distance.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
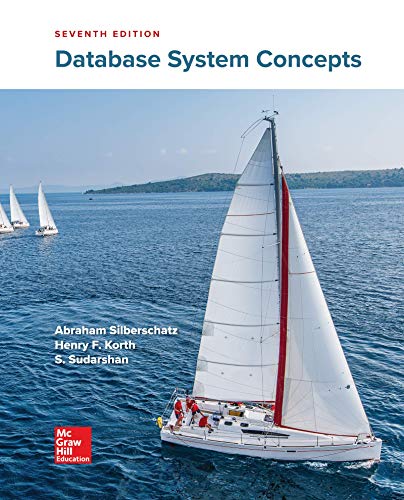
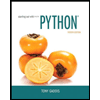
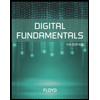
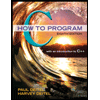
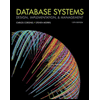
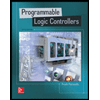