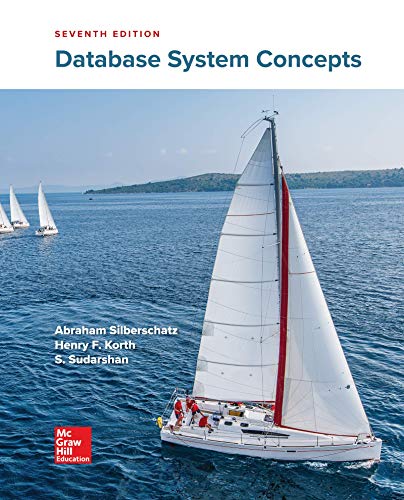
Python Question #6
Two programs are required and pseudocode is needed for each step
program6_1.py
Write a
Sample Output (see 6_1.png)
program6_2.py
This program reads the text file created in the program above and displays its data. Use f-strings to create a table of rows and columns with column headings as follows:
- PLAYER COLUMN: 10 characters wide and left-aligned.
- GOALS COLUMN: 6 characters wide and centered.
- ASSISTS COLUMN: 8 characters wide and centered.
- TOTAL COLUMN: 6 characters wide and centered.
After printing the stats for each player, the program should report the name of the top scorer and his total points (goals + assists).
Sample Output (see image 6_2.png)
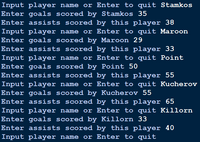
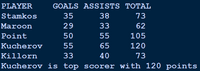

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 4 images

- Word Puzzle GameIn this assignment is only for individual. You are going to decode the scrambled word into correct orderin this game. One round of play is as follows:1. Computer reads a text file named words.txt2. Computer randomly picks one word in the file and randomly scrambles characters in the wordmany times to hide the word3. Computer displays the scrambled word to user with indexes and gives the user options to1. Swap two letters in word based on index given2. Solve the puzzle directly3. Quit the game4. If a player chooses to swap letters, the computer reads two indexes and swaps the letters. Thegame resume to step 3 with the newly guessed word. If the resulted word after swapping is thesecret word, the game is over.5. If a user chooses to solve directly, computer prompts the user to enter the guessed word. If it’scorrect, the game is over, otherwise goes to step 3.6. After game is over, display how many times the player has tried to solve the puzzle.The following is sample…arrow_forwardRange for loop should be used even the block code needs to access index. Group of answer choices True Falsearrow_forwardMenu option A -- Determine Hours to StudyThe program will READ in data from a text file named StudyHours.txt. The user corrects any bad data. The program updates the information in StudyHours.txt file. For example if the file contains a letter grade of K which is not a possible letter grade. You will create and submit a text file with a minimum of 5 additional records from example below. The file is named StudyHours.txt and contains the following format: first line full namesecond line number of creditsthird line grade desired for each class Example format StudyHours.txt fileAaron RODgers12ATom brady9Kphilip RiversapplecJoe Theismann15B The program determines the total weekly study hours (for all classes)All data must be displayed in proper case such as Wendy Payne, i.e. no names should be in all lower case or all upper case or a mix such as wendy or PaYNe. Use a function to convert to proper case.The program displays the student’s name, number of credits, expected total number of…arrow_forward
- /** * @file main.cpp * @brief This is the main file for the baseball champions program * @author Connor Melton * @date March 12, 2013 * */// INCLUDE THE HEADER FILES NEEDED FOR THE FUNCTIONS TO WORK#include "baseball.h" int main(){ ifstream teamFile; teamFile.open("./Teams.txt"); string teams[30]; int teams_count = 0; while (getline(teamFile, teams[teams_count])) { teams_count++; } teamFile.close(); ifstream winnersFile; winnersFile.open("./WorldSeriesWinners.txt"); // 1. Check if file opened successfully, if not exit the program // 2a. Declare an array of strings to store data for world series winners // 2. Load data from winnersFile to an array by calling loadWinnersFromFileToArray // 3. Close the file stream // Read the instructions on what else you need to finish this program string winners[65]; int winnersCount = 0; while (getline(winnersFile, winners[winnersCount])) { winnersCount++; }…arrow_forwardC Sharp Random Number File writer and Reader Create an application that writes a series of random numbers to a file. Each random number should be in the range of 1 through 100. The application should let the user specify how many randome numbers the file will hold and should use a SaveFileDialog control to let the user specify the file's name and Location. Create another application that uses an OpenFileDialog control to let the user select the file that was created above. This application should read the numbers from the file, display the numbers in a ListBox control and display: the total of the numbers and the number of random numbers read from the file.arrow_forward>> classicVinyls.cpp For the following program, you will use the text file called “vinyls.txt” attached to this assignment. The file stores information about a collection of classic vinyls. The records in the file are like the ones on the following sample: Led_Zeppelin Led_Zeppelin 1969 1000.00 The_Prettiest_Star David_Bowie 1973 2000.00 Speedway Elvis_Presley 1968 5000.00 Spirit_in_the_Night Bruce_Springsteen 1973 5000.00 … Write a declaration for a structure named vinylRec that is to be used to store the records for the classic collection system. The fields in the record should include a title (string), an artist (string), the yearReleased (int), and an estimatedPrice(double). Create the following…arrow_forward
- BAGGINS Trucking maintains a trip file in which each record contains the following data:DRIVER nameMILES driven on tripHOURS driven on trip Design an application that inputs records from the file (until reaching "eof") and, for each record, displays the DRIVER's name and the average Miles-per-Hours (MPH) for the trip where MPH is calculated by dividing the MILES by the HOURS. The program should also accumulate a GRAND total of the MILES driven. At the end, the program should display the GRAND total of the MILES driven. For example, if the trip file contained the following:Smith, 300, 5Williams, 750, 10Jones, 880, 11 then the program should display:Smith averaged 60 mphWilliams averaged 75 mphJones averaged 80 mphGrand total of miles is 1,930 Pseudocode:arrow_forwardC++ Visual 2019 A file called num.txt. write a C++ program that opens a file, reads all the numbers from the file and calculates the following: The number of numbers in the file The sum of all the numbers in the file (a running total) The average of all the numbers in the file The program should display the number of numbers found in the file, the sum of the numbers and the average of the numbers. These are the numbers in the num.txt file :…arrow_forwardplease save number random and file and when print on monitor read from file and every num in single linearrow_forward
- python function that creates and saves data in a file. the saved data represents exam grades. in the function , you will create n random numbers in the range 1-100, where n is the number of students. The function can be called as follows: createFile(filename, n) main function, in which the user inputs the file name and the number of students, then the main calls function createfile. the main should preform validation for n (should be > 0), and the filename(should end with .txt)arrow_forwardC programarrow_forwardC lanaguge Managing Student Information! C LANGUAGE We're going to build a student information management system. The user should be shown a menu with four options: display student initials, display student GPAs, add students, or exit the program. If the user chooses to exit, the program should end. Otherwise, if the user chooses to display the student initials, the contents of the students.txt file should be read, and just the student number and initials should be displayed to the user in a tabular format. If the user chooses to display the student GPAs, the contents of the students.txt file should be read, and just the student number and GPAs should be displayed to the user in a tabular format. If the user chooses to add students, they should be prompted for the number of studnets they’d like to add. They should then be prompted that many times for a studnet number, a GPA, a first initial, and a last initial. Each of the new items should be saved to the students.txt file.…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
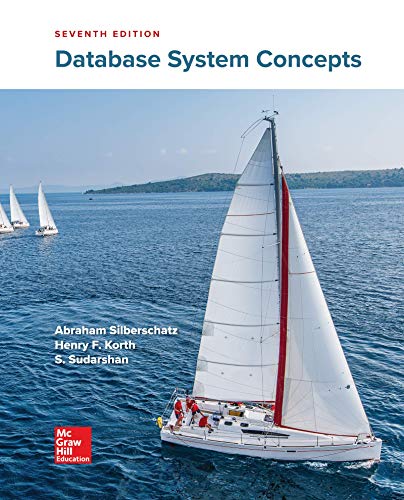
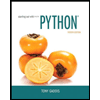
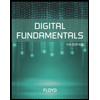
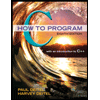
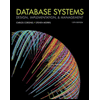
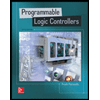