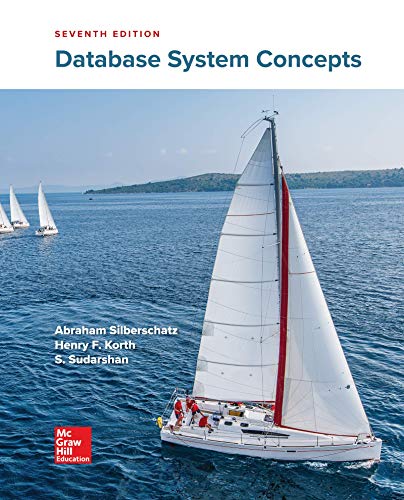
Write a Python code
This lab requires you to write a complete
Write a program that will allow a grocery store to keep track of the total number of bottles collected for seven days.
The program will calculate the total number of bottles returned for the week and the amount paid out (the total returned times .10 cents).
The output of the program should include the total number of bottles returned and the total paid out.
The program will ask the user if they have more data to enter and will end the program if they do not.
Step 1: In the pseudocode below, declare the following variables under the documentation for Step 1.
- A variable called totalBottles that is initialized to 0
- This variable will store the accumulated bottle values
- A variable called counter and that is initialized to 1
- This variable will control the loop
- A variable called todayBottles that is initialized to 0
- This variable will store the number of bottles returned on a day
- A variable called totalPayout that is initialized to 0
- This variable will store the calculated value of totalBottles times .10
- A variable called keepGoing that is initialized to "y"
- This variable will be used to run the program again
Step 2: In the pseudocode below, make calls to the following functions under the documentation for Step 2.
- A function call to getBottles that returns the total number of bottles for the week.
- A function called calcPayout that passes totalPayout and It returns the total payout for the week.
- A module called printInfo that passes totalBottles and
Step 3: In the pseudocode below, write a condition controlled while loop around your function calls using the keepGoing variable under the documentation for Step 3.
Complete Steps 1-3 below:
Module main ()
// Step 1: Declare variables below
___________________________________________________
___________________________________________________
___________________________________________________
___________________________________________________
___________________________________________________
// Step 3: Loop to run program again
While _________________________________
// Step 2: Call functions
totalBottles = _________________________________
totalPayout = _________________________________
________________________________________________
Display "Do you want to enter another week’s worth of data?"
Display "(Enter y or n)"
Input _________________________________
End While
End Module
Step 4: In the pseudocode below, write the missing lines, including:
- The 3 missing variable declarations and initializing them to 0
- The missing condition (Hint: should run seven iterations)
- The missing input variable
- The missing accumulator
- The increment statement for the counter
- The missing return statement
// getBottles function
Function getBottles()
NBR_OF_DAYS = 7
// Declare and initialize totalBottles, todayBottles, counter to 0
- ___________________________________
___________________________________
___________________________________
While b._______________________________
Display "Enter number of bottles returned for day #",
counter, ":"
Input c.______________________________
- ___________________________________
- ___________________________________
End While
Return f._______________________________
End Function
Step 5: In the pseudocode below, write the missing lines, including:
- The missing parameter list
- The missing calculation
- The missing return statement
// calcPayout function
Function Real calcPayout(a.______________________)
PAYOUT_PER_BOTTLE = .10
totalPayout = 0 // resets to 0 for multiple runs
- ______________________________
Return c._________________________
End Function
Step 6: In the pseudocode below, write the missing lines, including:
- The missing parameter list
- The missing display statement
- The missing display statement
// printInfo module
Module printInfo(a.______________________________________)
- _______________________________________________
- _______________________________________________
End Module

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Python Upvote for VERY QUICK answer. No need to comment the code. Thank you.arrow_forwardPython programing language 1. You just got a job working for the local Honda motorcycle shop. For every motorcycle you sell you get 10% commission. Using a while loop create the following output. Enter the sale amount: 9999Your commission is $999.90.Your paycheck will be $999.90.Did you make another sale: YEnter the sale amount: 4599Your commission is $459.90Your paycheck will be $1,459.80Did you make another sale: N 2. Write an application that uses a while loop to validate 3 test scores. What is the first test score: 101Please re-enter a number between 0 and 100What is the first test score: 100What is the second test score: 110Please re-enter a number between 0 and 100What is the second test score: 99What is the third test score: 87With an average score of 95.33% you are on track for an A in this course.arrow_forwardjava programming A lot of people have been in the real estate market this year and 2 measurements are used to measure land, acres and hectares. You are writing a program to convert acres to hectares for lots of land given in acres. You begin by asking the user how many conversions that they want to make for lots of land. Once you have a response, a for loop is entered for processing the lots of land. You will input the lot# and the acres for the lot and this information will be stored in parallel arrays. Use a value returning method to validate the value of the acres to make sure that it is not <1 acre. Print the lot#, and the acres within a 2nd for loop. Find the lot with the smallest acreage. Use a void method convertHectares that converts the sum of the acres of land processed by a factor of 2.471052, and then displays the Hectares. The following methods are required in this program: A value returning method to validate the acres to make sure that it is at least 1. A…arrow_forward
- The Lottery Problem: • The lottery draws a number that is the winning number • The number should be between 0 and 999 • Have someone play • The person tells how many tickets they want • The person always does “quick pick” (let the computer pick the number for them) and gets one number between 0 and 999 for each ticket bought • The program reports how many tickets win of the ones bought • NOTE: A ticket “wins” if it matches the winning number previously chosen • Write working code with at least one function and one sub other than “main”arrow_forward//PROBLEM 1/* This program should play a game with the user asking her to guess a target number (randomly generated integer between 1 and 10). The user gets 3 attempts. If she gets it wrong, the program tells her to go up or down, depending on whether her number was less or more then the target. The output may look like this, for example: ============= Guess the number between 1 and 10 in THREE attempts. Enter your first guess: 4 Nope. Go up. Enter your second guess: 6 Nope. Go down. Enter your third and final guess: 5 You got it! CONGRATULATIONS! ============= or like this: ============= Guess the number between 1 and 10 in THREE attempts. Enter your first guess: 4 Nope. Go up. Enter your second guess: 6 Nope. Go up. Enter your third and final guess: 8 GAME OVER! You lost! The number was 9arrow_forward: Write a python program to simulate an online store. The program should begin by displaying a list of products and their prices. There should be a minimum of 4 products offered. The program should ask the user to choose a product and then ask the user to enter the quantity they require of that product. The program should then allow the user to keep choosing more products and quantities until they enter something to indicate they want to end the program (e.g. a given number or ‘q’ or ‘exit’). The program should then tell the user the total amount for the products they have selected.arrow_forward
- 4.3-4a. IPv4/IPv6 co-existence: tunneling (a). Consider the mixed IPv4/IPv6 network shown b where an IPv4 tunnel exists between IPv6 routers B and E. Suppose that IPv6 router A sends a datagram to IPv6 router F. IPv6 datagrams are shown in blue; the IPv4 datagram is in red (cont. the encapsulated IPv6 datagram in blue). A IPv6 (a) B IPv6/v4 IPv4 (b) At point (a), the IP version field in the datagram is: At point (a), the source IP address is that of host: D IPv4 Perform the matching below to indicate the datagram field value and type at point (a). At point (a), the destination IP address is that of host: At point (a), the number of bits in the destination IP address is: E IPv6/v4 IPv6 (c) [Choose ] [Choose ] [Choose ] [Choose ]arrow_forwarduse java programingarrow_forwardValidating User Input Summary In this lab, you will make additions to a Java program that is provided. The program is a guessing game. A random number between 1 and 10 is generated in the program. The user enters a number between 1 and 10, trying to guess the correct number. If the user guesses correctly, the program congratulates the user, and then the loop that controls guessing numbers exits; otherwise, the program asks the user if he or she wants to guess again. If the user enters a "Y", he or she can guess again. If the user enters "N", the loop exits. You can see that the "Y" or an "N" is the sentinel value that controls the loop. Note that the entire program has been written for you. You need to add code that validates correct input, which is "Y" or "N", when the user is asked if he or she wants to guess a number, and a number in the range of 1 through 10 when the user is asked to guess a number. Instructions Ensure the file named GuessNumber.java is open. Write loops…arrow_forward
- C Language - Write a program that takes in three integers and outputs the largest value. If the input integers are the same, output the integers' value.arrow_forwardUsing a Sentinel Value to Control a while Loop Summary In this lab, you write a while loop that uses a sentinel value to control a loop in a Java program that has been provided. You also write the statements that make up the body of the loop. The source code file already contains the necessary variable declarations and output statements. Each theater patron enters a value from 0 to 4 indicating the number of stars the patron awards to the Guide’s featured movie of the week. The program executes continuously until the theater manager enters a negative number to quit. At the end of the program, you should display the average star rating for the movie. Instructions Ensure the file named MovieGuide.java is open. Write the while loop using a sentinel value to control the loop, and write the statements that make up the body of the loop to calculate the average star rating. Execute the program by clicking Run. Input the following: 0, 3, 4, 4, 1, 1, 2, -1 Check that the average…arrow_forwardflow chart and dimple program. Python thank youarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
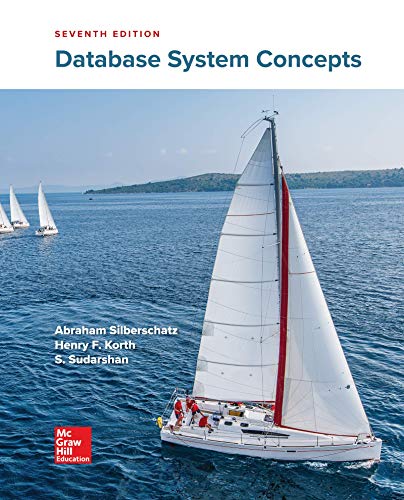
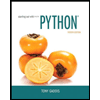
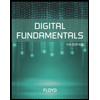
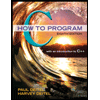
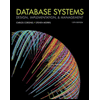
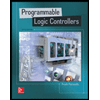