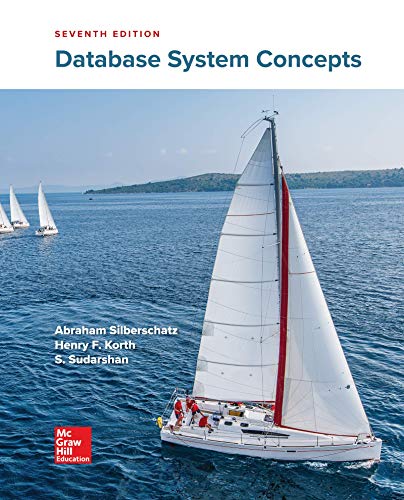
Write a python program that takes two lists, merges the
two lists, sorts the resulting list, and then finds the median of the
elements in the two lists.
**<font color='red'>[You cannot use python build-in sort() function, you can call your own sort function or copy your code from previous tasks instead]</font>**
=====================================================
**Sample Input 1**
list_one = \[1, 2, 1, 4\]
list_two = \[5, 4, 1\]
**Sample Output 1**
Sorted list = \[1, 1, 1, 2, 4, 4, 5\]
Median = 2
=====================================================
**Sample Input 2**
list_one = \[1, 7, 9, 10\]
list_two = \[2, 7, 6, 5\]
**Sample Output 2**
Sorted list = \[1, 2, 5, 6, 7, 7, 9, 10\]
Median = 6.5
this is my code but its not returning median
#todo
#You can use math functions like math.floor to help the calculation
import math
def task7(list_in_1, list_in_2):
# YOUR CODE HERE
list_in_1.extend(list_in_2)
sort_list=[]
while list_in_1:
min=list_in_1[0]
for x in list_in_1:
if x<min:
min=x
sort_list.append(min)
list_in_1.remove(min)
print("Sorted List ",sort_list)
if(len(sort_list))%2!=0:
median=int((len(sort_list)+1)/2-1)
return sort_list[median]
else:
median1=int(len(sort_list)/2-1)
median2=int(len(sort_list)/2)
return (sort_list[median1]+sort_list[median2])/2
n=int(input("no of elements in List1\n"))
l1=[]
print("Enter elements in List1")
for i in range(n):
i=int(input(""))
l1.append(i)
k=int(input("no of elements in list2\n"))
print("Enter elements of list2")
l2=[]
for j in range(k):
j=int(input(""))
l2.append(j)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- This is for Python version 3.8.5 and please include pseudocode. nums = [8, 15, 6, 17, 33, 20, 14, 9, 12]Write Python code that: uses a function to print the size of the list named nums uses a function to print the largest number in nums prints the element 14 by using its index makes a slice named subnums containing only 33, 20, and 14arrow_forwardin python pleasearrow_forwardUse Python when answering the question: Write a function primeFac that computes the prime factorization of anumber: it accepts a single argument, an integer greater than 1 it returns a list containing the prime factorization each number in the list is a prime number greater than 1 the product of the numbers in the list is the original number the factors are listed in non-decreasing order Output is in the attached picture:arrow_forward
- In Python IDLE: How would I write a function for the problem in the attached image?arrow_forwardPYTHON!!! Write a function that will filter course grades that are stored in a list, creating and returning a new list. Your implementation must have the following rules: - The function must accept two (and only two) parameters: The first is: the maximum integer value for any grade to remain in the list (any value above the maximum will be filtered and removed from the list). The second is: the list of grades (you can assume the list contains only integer values) - The function must return a new list and not modify the existing list. - The function must use a list comprehension to filter the existing list and create the new list that is returned. - DO NOT USE THE: built-in-"filter" function.arrow_forwardIn pythonarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
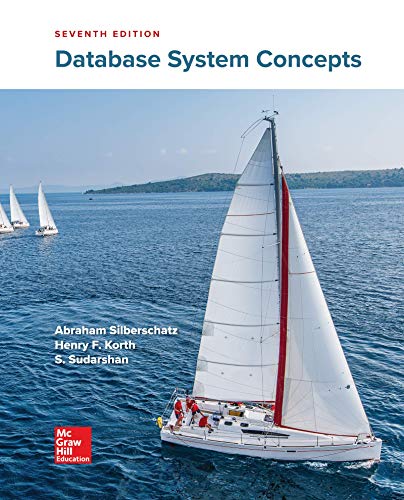
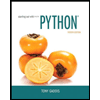
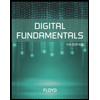
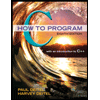
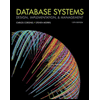
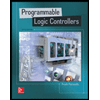