Write a static method named "bigBeforeSmall" that accepts an array of Strings as a parameter and rearranges its elements so that longer Strings appear before shorter Strings. For example, if the following array is passed to your method: String[] numbers = { "one", "twelve", "three", "four", "fourteen", "six" }; Then after the method has been called, one acceptable ordering of the elements would be: ["fourteen", "twelve", "three", "four", "one", "six" ] The exact order of the elements does not matter, so long as all longer Strings appear before all shorter Strings. Another acceptable order would be: ["fourteen", "twelve", "three", "four", "six", "one" ] Do not make any assumptions about the length of the array or the range of values it might contain. For example, the array might contain empty Strings (length zero), or all Strings could have the same length. You may assume that the array is not null (empty). Hint: This is actually a sorting problem. In BubbleSort you tested pairs of elements and swapped them if the left element is larger than the right element. In this problem, the swap happens when the left element has fewer characters than the right element. You also may not use any other data structures such as the ArrayList class from Chapter 10. DO NOT use Arrays.sort in your solution. You may use the following code to test your program: If your method is modeled after a bubble sort, expected output is: ["fourteen", "twelve", "three", "four", "one", "six" ] import java.util.*; public class BigOnesFirst { public static void main(String[] args) { String[] numbers = {"one", "twelve", "three", "four", "fourteen", "six"}; bigBeforeSmall(numbers); System.out.println(Arrays.toString(numbers)); } // *** Your method code goes here *** } // End of BigOnesFirst class
Write a static method named "bigBeforeSmall" that accepts an array of Strings as a parameter and rearranges its elements so that longer Strings appear before shorter Strings. For example, if the following array is passed to your method:
String[] numbers = { "one", "twelve", "three", "four", "fourteen", "six" };
Then after the method has been called, one acceptable ordering of the elements would be:
["fourteen", "twelve", "three", "four", "one", "six" ]
The exact order of the elements does not matter, so long as all longer Strings appear before all shorter Strings. Another acceptable order would be:
["fourteen", "twelve", "three", "four", "six", "one" ]
Do not make any assumptions about the length of the array or the range of values it might contain. For example, the array might contain empty Strings (length zero), or all Strings could have the same length. You may assume that the array is not null (empty).
Hint: This is actually a sorting problem. In BubbleSort you tested pairs of elements and swapped them if the left element is larger than the right element. In this problem, the swap happens when the left element has fewer characters than the right element.
You also may not use any other data structures such as the ArrayList class from Chapter 10.
DO NOT use Arrays.sort in your solution.
You may use the following code to test your program:
If your method is modeled after a bubble sort, expected output is:
["fourteen", "twelve", "three", "four", "one", "six" ]
import java.util.*;
public class BigOnesFirst {
public static void main(String[] args) {
String[] numbers = {"one", "twelve", "three", "four", "fourteen", "six"};
bigBeforeSmall(numbers);
System.out.println(Arrays.toString(numbers));
}
// *** Your method code goes here ***
} // End of BigOnesFirst class

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

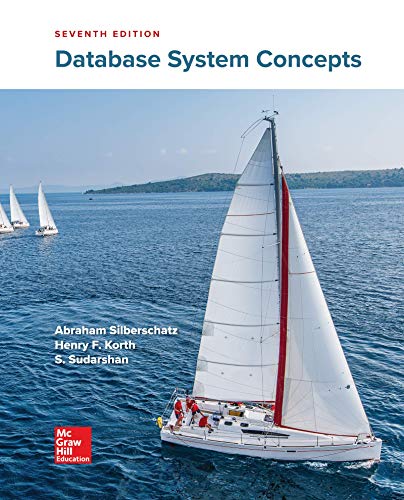
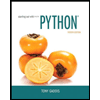
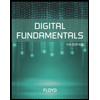
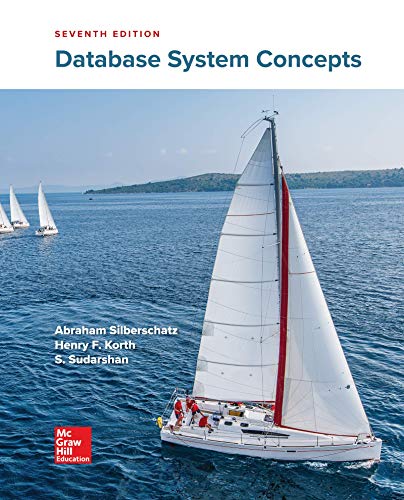
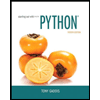
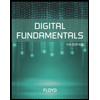
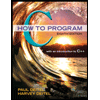
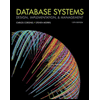
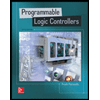