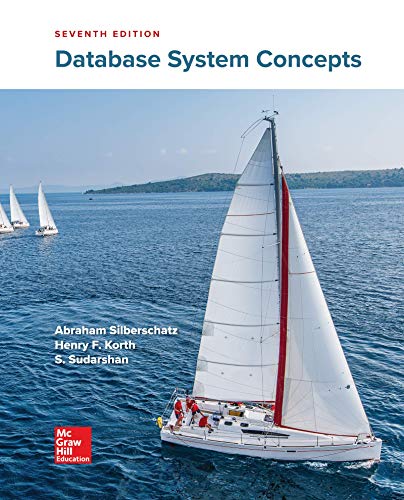
Concept explainers
NEED C++ HELP WITH WRITING
Write code to read a list of song durations and song names from input. For each line of input, set the duration and name of currSong.
Then add currSong to playlist.
Input first receives a song duration, then the name of that song (which you can assume is only one word long).
Input example: 424 Time
383 Money
-1
CODE AS IS NOW
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class Song {
public:
void SetDurationAndName(int songDuration, string songName) {
duration = songDuration;
name = songName;
}
void PrintSong() const {
cout << duration << " - " << name << endl;
}
int GetDuration() const { return duration; }
string GetName() const { return name; }
private:
int duration;
string name;
};
int main() {
vector<Song> playlist;
Song currSong;
int currDuration;
string currName;
unsigned int i;
cin >> currDuration;
while (currDuration >= 0) {
/* Your code goes here */
cin >> currDuration;
}
for (i = 0; i < playlist.size(); ++i) {
currSong = playlist.at(i);
currSong.PrintSong();
}
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Write a c++ function reportDuplicates() that takes a two dimensional integer array as a parameter and identifies the duplicate valuesin the array. The function then reports these to user. Sample input and corresponding output:How many rows? 5How many columns? 2Let’s populate the array:1 86 97 312 522 4Thank you, there are no duplicate elements!How many rows? 3How many columns? 4Let’s populate the array:3 7 5 76 9 7 38 5 12 6Thank you, 3 appears 2 times, 7 appears 3 times, 5 appears 2 times,and 6 appears 2 times.arrow_forwardWrite a program in C++ that repeatedly prompts the user to enter a capital for a state. Upon receiving the user input, the program reports whether the answer is correct. A sample run is shown below: What is the capital of Alabama? Montgomery [ENTER]Your answer is correct.What is the capital of Alaska? Anchorage [ENTER]The capital of Alaska is Juneau Assume that fifty states and their capitals are stored in a two-dimensional array, see below. The program prompts the user to enter ten states’ capitals and displays the total correct count.arrow_forwardC++arrow_forward
- 1. Write a program that defines a struct named Info. That Info struct should define two strings, a double and array of strings. In your main program, define a variable v using that Info struct and then initialize it to hold your first name, your last name, your lucky non-whole number (e.g. 26.5), your major, and a listing (an array) of your six most favorite college courses you have ever taken or plan to take. Then your program should access and print all the stored data held in the variable v. 2. print the data twice, once using the structure variable and do-while loop, and next using a pointer variable and a while-do loop.) Using the embed icon shown above show screenshots demoing the execution of your program.arrow_forwardGiven an array of integers of size 10. Write a C++ function that takes the array as a parameter and returns the numbers of elements in the array that are small. A number is called "small" if it is in the range 1..10 inclusive. int ele[10] = {12, 56, -18, 1, 6, 4, 92, 2, 34, 78};; Expected Output: 4arrow_forwardI need to write a program named Search.java that uses a function called search_string to check whether a string exists in the array or not. 1- The array of strings is iterated using a for loop and the value at every index is compared with the value to be searched in the array. 2- A boolean variable is set if any array value matches with the string. 3- At the end of the loop, this boolean variable is checked to determine if the array contains the string. It may be necessary to import java.util.Arrays then use Arrays.toString, String equals(). Output should be as picture showsarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
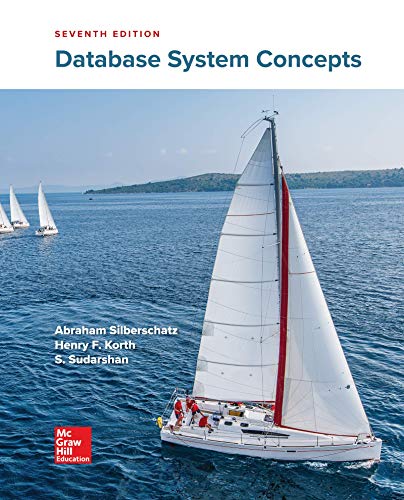
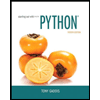
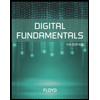
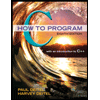
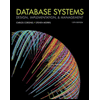
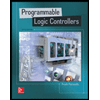