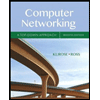
By implementing the concepts of Classes and Objects, write a C++ program that determines the slope of a line from the coordinates, P(x,y), of the 2 points. The coordinates of the 2 points: P1 (x1, y1) and P2 (x2, y2) are to he entered from the keyboard. The slope, m, of the line is computed as: m=(y2-y1/x2-x1)
Accomplish the following for the given class diagram:
1.) Create the class implementation using C++
2.) Using the constructor, create 2 objects, P1 and P2, from class Point and store them in the heap memory.
3.) Implement the functions of the 2 objects.
NOTE: The member function setXY is used to enter the values of x and y from the keyboard. Sample input/output display:
Enter the coordinates of the 1st point (x1 y1): 1 1
Enter the coordinates of the 2nd point (x2 y2): 5 4
The slope of the line, m=0.75
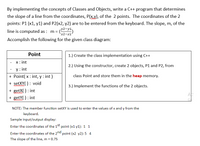

Step by stepSolved in 4 steps with 3 images

- I need help with the exercise problem 10-16 from C++ Programming II by D.S. Malik. The instruction was: Write the definition of a class swimmingPool, to implement the properties of a swimming pool. Your class should have the instance variables to store the length (in feet), width (in feet), depth (in feet), the rate (in gallons per minute) at which the water is filling the pool, and the rate (in gallons per minute) at which the water is draining from the pool. Add appropriate constructors to initialize the instance variables. Also, add member functions to do the following: determine the amount of water needed to fill an empty or partially filled pool, determine the time needed to completely or partially fill or empty the pool, and add or drain water for a specific amount of time, if the water in the pool exceeds the total capacity of the pool, output "Pool overflow" to indicate that the water has breached capacity. The header file for the swimmingPool class has been provided for…arrow_forwardWrite a C++ Program using classes, functions (recursive and otherwise), arrays and other C++ commands for a friendly game of BlackJack (no bets), according to the rules in Wikipedia. The program would ask if the user wants to play a game and draw the cards. Depending on the drawn cards, the game would progres according to the rules. When the game ends, the program asks if the user wants to play again. You can use a Joker of any card type as a Wild Card valued up to the highest rated card in the deck needed to complete the card hand, if applicable; if not, the player decides the value of the Joker for their card hard (user's hand or program' hand). The game will keep running as long as the user states so.arrow_forwardWRITE CODE IN C LANGUANGE On their way down the river, Jojo and Lili saw two frogs each in position X1 and X2. The two frogs are seen jumping happily towards the same direction that is come to Jojo. After watching the two frogs, Joio and Lili saw that the speed the two frogs are different. The first frog to start jumping from position X1 has a speed of v1, while the second frog that starts to jump from position X2 has a speed of V2. Joig guess that "YES" both frogs will be in the same position in a certain time T, while Bibi guessing "NO" the two frogs will never be in the same position in time. Help Joio and Lili calculate the frog's movements to determine whether the guess they are rightarrow_forward
- The Java code must have the structure as shoen in the picture: Develop with Java programming language a calculator (console application) that takes a number, a basic math operator ( + , - , * , / , % ), and a second number all from user input, and have it print the result of the mathematical operation. The mathematical operations should be wrapped inside of functions. Note: Do please use the provided source code template to implement your solution. The purpose of this problem is not only to assess the students' ability to build a valid implementation but also to assess the ability to read the Java code. Input: On single line a number, a basic math operator ( + , - , * , / , % ), and a second number all from user input. Output: On new line result of the mathematical operation. In the case of "Division by zero" you must printout the text message "Error". Example 1: 4 + 812 Example 2: 4 * 832 Example 3: 4 % 20 Example 4 4/0 Error Example 5: 4-10 -6arrow_forwardCreate a C++ address book using the following information: The address should contain a Person class. string firstName; string middleName; string lastName; Each person’s detailed address should be provided as follows: int block; int unit; int floor; string street; string city; string country; int postalCode; Write appropriate getters/ setters and test your implementation.arrow_forwardWrite a C++ Program using classes, functions (recursive and otherwise), arrays and other C++ commands for a friendly game of BlackJack (no bets), according to the rules in Wikipedia. The program would ask if the user wants to play a game and draw the cards. Depending on the drawn cards, the game would progres according to the rules. When the game ends, the program asks if the user wants to play again. You can use a Joker of any card type as a Wild Card valued up to the highest rated card in the deck needed to complete the card hand, if applicable; if not, the player decides the value of the Joker for their card hard (user s hand or program' hand). The game will keep running as long as the user states so.arrow_forward
- Write a C++ program for the following. In each plastic container of Pez candy, the colors are stored in random order. Your little brother only likes the yellow ones, so he painstakingly takes out all of the candies, one at a time, eats the yellow ones, and keeps the others in order so he can return them to the container in exactly the same order as before, minus the yellow candies, of course. Write a program to simulate this process. You must use Nyhoff's stack class; other solutions including the use of the STL stack class are unacceptable. Assume that the candies are denoted as Y for yellow, B for blue, R for red, O for orange. Your program is to query the user for ten letters (representing ten Pez candies), and after using appropriate stack operations, display the contents of the container minus the yellow candies. The order in which the letters are entered is the order that they were removed from the Pez container. When your answer is displayed the results must be in the same…arrow_forwardWrite a Java function name fracpart() that returns the fractional part of any number passed to the function. For example, if the number 256.979 is passed to fracpart(), the number .979 should be returned. Have the function fracpart() call the function whole(). The number returned can then be determined as the number passed to fracpart() less the returned value when the same argumetn is passed to whole. The completed program should consist of main () followed by fracpart() followed by whole(). how can i execute this in c++arrow_forwardImplement a function alterCase in Python that converts a word to "alterCase". Given a word, using any mix of upper and lower case letters, the function then returns the same word, except that the first letter is upper case, the second is lower case, and then cases of letters alternate throughout the rest of the word. >>> alterCase('apple')'ApPlE'arrow_forward
- javascript Need help defining a function frequencyAnalysis that accepts a string of lower-case letters as a parameter. frequencyAnalysis should return an object containing the amount of times each letter appeared in the string. example frequencyAnalysis('abca'); // => {a: 2, b: 1, c: 1}arrow_forwardCreate a social network, Chirper, that lets the user add new Chirps and like existing Chirps. It's lonely because only one person can add and like messages -- so there's really not much "social" about it. At least you can practice using objects, references, and function overloading in C++! Here's an example of how this program will run You can "chirp" a new message to Chirper, or "like" an existing chirp, or "exit". What do you want to do? chirp What's your message? This is my first chirp! Chirper has 1 chirps: 1. This is my first chirp! (0 likes) You can "chirp" a new message to Chirper, or "like" an existing chirp, or "exit". What do you want to do? like Which index do you want to like? 1 Chirper has 1 chirps: 1. This is my first chirp! (1 likes) You can "chirp" a new message to Chirper, or "like" an existing chirp, or "exit". What do you want to do? chirp What's your message? Second chirp is the best chirp. Chirper has 2 chirps: 1. This is my first chirp! (1 likes) 2. Second chirp…arrow_forwardIn JAVA A function foo takes three integers as input arguments, i.e., foo(int a, int b, int c). The three inputs represent the three sides of a triangle in centimeter. The function is expected to return the type of a triangle: “equilateral”, “isosceles”, or “scalene”. Assume the domains of the three variables are 1 ≤ a ≤ 100, 50 ≤ b ≤ 150 and 100 ≤ c ≤ 200, respectively. A test case is in a tuple format <a, b, c, expected_output> with test inputs and the expected output. S1 is a set of test cases for the “Boundary Value Analysis” approach. S1 = S2 is a set of test cases for the “Robustness testing” approach. S2 – S1 = S3 is a set of tests cases for the “Robust Worst-Case testing” approach. Are there any types of triangles that S3 cannot reveal? If yes, what are they? If no, why?arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
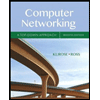
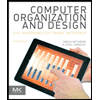
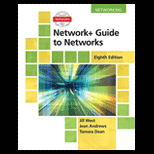
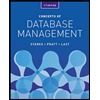
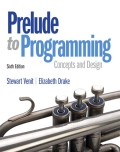
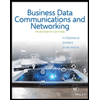