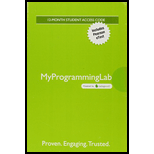
( HugeInteger Class) A machine with 32- bit integers can represent integers in the range of approximately-2 billion. This fixed-size restriction is rarely troublesome, but there are applications in which we would like to be able to use a much wider range of integers. This is what C++ was built to do, namely, create powerful new data types. Consider class HugeInteger of Figs. 10.17-10.19, which is similar to the HugeInteger class in Exercise 9.14. Study the class carefully, then respond to the following:
- Describe precisely how it operates.
- What restrictions does the class have?
- Overload the * multiplication operator.
- Overload the / division operator.
- Overload all the relational and equality operators.
[Note: We do not show an assignment operator or copy constructor for class HugeInteger, because the assignment operator and copy constructor provided by the compiler are capable of copying the entire array data member properly.]
- // Fig. 10.17: HugeInteger.h
- // HugeInteger Class definition.
- #ifndef HugeInteger H
- #define HugeInteger H
- #include
- #include
- #include
Fig.10.17 HugeInteger Class Definition. (Part 2of 2)
1 // Fig. 10.18; HugeInteger.Cpp
2 // HugeInteger member-function and friend-function definitions.
3 #include
4 #include “HugeInteger .h” // HugeInteger Class definition
5 using namespace;
6
7 // default constructors; conversion constructor that converts
8 // a long integer into a HugeInteger object
9 HugeInteger ::HugeInteger (long value) {
10 // place digits of argument into array
11 for (int j {digits -1} ; value != 0 && j >= 0; j--) {
12 integer [ j ] = value % 10;
13 value /= 10;
14 }
15 }
16
17 // Conversion constructor that converts a character string
18 // representing a large integer into a HugeInteger object
19 HugeInteger ::HugeInteger (const string&number) {
20 // place digits of argument into array
21 int length { number, size () };
22
23 for (int j {digits -length}, k {0}; j < digits; ++j, ++k) {
24 if (isdigit (number [k])) { // ensure that character is a digit
25 integer [j] = number [k] − ‘0’;
26 }
27 }
30 // addition operator; HugeInteger + HugeInteger
31 // HugeInteger HugeInteger :: operator+(const HugeInteger & op2) const {
32 HugeInteger temp; // temporary result
33 int carry= 0;
34
35 for (int I (digits-1) ; I >=0; i--) {
36 temp.integer [i] = integer [i] op2.integer [i]
+ carry:
37
38 //determine whether to carry a 1
39 If (temp.integer[i] > 9) {
40 temp.integer [i] %-10; //reduce to
41 carry= 0;
42 }
43 else { // no carry
44 carry = 0;
45 }
46 }
47
48 return temp; // return copy of temporary object
49 ]
50
51 // addition operator; HugeInteger + int
52 HugeInteger HugeInteger ::operators+(int op2) const {
53 // convert op2 to a HugeInteger , then invoke
54 // operator + for two HugeInteger objects
55 return *this + HugeInteger (op2);
56 }
57
58 //addition operator ;
59 // HugeInteger + string that represents large integervalue
60 HugeInteger HugeInteger ::operator+(const string&op2) const {
61 // convert op2 to a HugeInteger , then invoke
62 //operator + for two HugeInteger objects
63 return *this +HugeInteger (op2);
64 }
65
66 // overloaded output operator
67 ostream & operator <<(ostream & output, const HugeInteger & num ) {
68 int I ;
69
70 // skip leading zeros
71 for (I =0; (I < HugeInteger ::digits) && (0== num.integer [i] ) ; ++i) { }
72
73 if (I ==HugeInteger ::digits) {
74 output << 0;
75 }
76 else {
77 for (; I < HugeInteger :: digits; ++i) [
78 output << num.integer [i]
79 ]
80 ]
81
82 return output
83 }
- // Fig. 10.19: Fig10_19.cpp
- // HugeInteger test program.
- #include < iostream>
- #include “ HugeInteger.h”
- Using namespace std;
- Int main () {
- HugeInteger n1{7654321};
- HUgeInteger n2{7891234};
- HugeInteger n3{“999999999999999999999999999999999”};
- HUgeInteger n4{“1”};
- HugeInteger n5;
- Cout << “n1 is “ << n1 << “\nn2 is “ << n2
- << “nn3 is “ <<n3 << nn4 is “ <<n4
- << ‘\nn5 is “ << n5 << “\n\n”;
- N5 = n1 + n2 ;
- Cout << n1 << “ + ” << n2 << “ = ” << n5 << “\n\n”;
- Cout << n3 << “ + “ << n4 << “\n= “ << (n3 + n4) << “\n\n;
- n5 = n1 + n2;
- Cout << n1<< “+” << 9<< “ = “ << n5 << “\n\n”;
- n5 = n2 + “10000” ;
- Cout << n2 << “ +” << “10000” << “ + “ << n5 << end ] :
- }
N1 is 7654321 N2 is 7891234 N3 is 99999999999999999999999999999 N4 is 1 N5 is 0 765431+7891324=15545555 99999999999999999999999999999+1 =100000000000000000000000000000 7654321+9 = 7654330 7891234+ 10000 =7901234 |

Want to see the full answer?
Check out a sample textbook solution
Chapter 10 Solutions
Mylab Programming With Pearson Etext -- Access Code Card -- For C++ How To Program (early Objects Version)
Additional Engineering Textbook Solutions
Programming in C
Management Information Systems: Managing The Digital Firm (16th Edition)
Database System Concepts
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Concepts Of Programming Languages
- -Program C 2. Not my Favorites by CodeChum Admin We've already done looping through a series of numbers and printing out its squares, so how about we level it up and go with cubes this time? I have a condition though; I don't want to be associated with numbers that are divisible by 3 or 5 so if an integer is divisible by either of the two, don't make their cube values appear on the screen, please. Care to fulfill my request? Instructions: Print out the cube values of the numbers ranging from 1 to 1000 (inclusive). However, if the number is divisible by either 3 or 5, do not include them in printing and proceed with the next numbers. Tip: When skipping through special values, make use of the keyword continue and put them inside a conditional statement. Output Multiple lines containing an integer. 1 8 64 343 512 . . .arrow_forward1. Write C Statements for the followings:arrow_forwardWhat’s the output of the following programs?arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
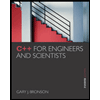
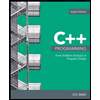