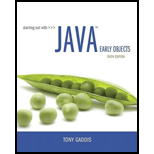
Starting Out with Java: Early Objects (6th Edition)
6th Edition
ISBN: 9780134462011
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 10, Problem 11PC
Program Plan Intro
- Define a class named “Employee”.
- Declare the required variables.
- Define a parameterize constructor to set “name” and “num”.
- Define a “setName()” method to set “name”.
- Define a “setEmpNum()” method to set “num”.
- Define a “setHrDate()” method to assign hire date
- Define a “getName()” method to return name.
- Define a “getEmpNum()” method to return employee number.
- Define a “getHrDate()” method to return hire date.
- Define a “isValidEmpNum()” method to return status.
- Define a “toString()” method to return string.
- Define a class named “InvalidEmpNum” that extends “Exception” class.
- Define a constructor with no parameters.
- Call “super()” method to print invalid number message.
- Define a class named “InvalidPayRate” that extends “Exception” class.
- Define a constructor with no parameters.
- Call “super()” method to print negative pay rate message.
- Define a class named “InvalidShift” that extends “Exception” class.
- Define a constructor with no parameters.
- Call “super()” method to print invalid shift number message.
- Define a class named “ProductionWorker” that extends “Exception” class.
- Define constant value for day and night shift.
- Declare required variables.
- Definition of parameterized constructor to set variables.
- Definition of constructor to initialize variables.
- Definition of “setShift()” method to set shift.
- Definition of “setPayRate()” method to set pay rate .
- Definition of “getShift()” method to return shift.
- Definition of “getPayRate()” method to return pay rate .
- Definition of “toString()” method to return string.
- Define a class named “WorkerDemo”.
- Define a “main()” method to check payroll exception.
- Create an object or reference variable.
- Test the exception.
- Declare the required variables.
- Define a parameterize constructor to set “name” and “num”.
- Define a “setName()” method to set “name”.
- Define a “setEmpNum()” method to set “num”.
- Define a “setHrDate()” method to assign hire date
- Define a “getName()” method to return name.
- Define a “getEmpNum()” method to return employee number.
- Define a “getHrDate()” method to return hire date.
- Define a “isValidEmpNum()” method to return status.
- Define a “toString()” method to return string.
- Define a constructor with no parameters.
- Call “super()” method to print invalid number message.
- Define a constructor with no parameters.
- Call “super()” method to print negative pay rate message.
- Define a constructor with no parameters.
- Call “super()” method to print invalid shift number message.
- Define constant value for day and night shift.
- Declare required variables.
- Definition of parameterized constructor to set variables.
- Definition of constructor to initialize variables.
- Definition of “setShift()” method to set shift.
- Definition of “setPayRate()” method to set pay rate .
- Definition of “getShift()” method to return shift.
- Definition of “getPayRate()” method to return pay rate .
- Definition of “toString()” method to return string.
- Define a “main()” method to check payroll exception.
- Create an object or reference variable.
- Test the exception.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
C++ Visual Studio 2019
Complete #13. Dependent #1 Employee and ProductionWorker classes showing below. Modify the Employee and ProductionWorker classes so they throw exceptions when the following errors occur:
The Employee class should throw an exception named InvalidEmployeeNumber when it receives an employee number that is less than 0 or greater than 9999.
The ProductionWorker class should throw an exception named InvalidShift when it receives an invalid shift.
The ProductionWorker class should throw an exception named InvalidPayRate when it receives a negative number for the hourly pay rate.
Write a driver program that demonstrates how each of these exception conditions works.
#1 Employee and ProductionWorker classes
#include <string>#include <iostream>#include <iomanip>using namespace std;
class Employee{private: string name; // Employee name string number; // Employee number string hireDate; // Hire date
public: // Default…
Instructions:
Exception handing
Add exception handling to this game (try, catch, throw). You are expected to use the standard exception classes to try functions, throw objects, and catch those objects when problems occur.
At a minimum, you should have your code throw an invalid_argument object when data from the user is wrong.
File GamePurse.h:
class GamePurse
{
// data
int purseAmount;
public:
// public functions
GamePurse(int);
void Win(int);
void Loose(int);
int GetAmount();
};
File GamePurse.cpp:
#include "GamePurse.h"
// constructor initilaizes the purseAmount variable
GamePurse::GamePurse(int amount){
purseAmount = amount;
}
// function definations
// add a winning amount to the purseAmount
void GamePurse:: Win(int amount){
purseAmount+= amount;
}
// deduct an amount from the purseAmount.
void GamePurse:: Loose(int amount){
purseAmount-= amount;
}
// return the value of purseAmount.
int GamePurse::GetAmount(){
return purseAmount;
}
File…
Create a tornadoException exception class.
The class should have two constructors, one of which should be the default function Object() { [native code] }.
If the default function Object() { [native code] } throws an error, the function should return "Tornado: Take cover immediately!"
The other function Object() { [native code] } takes a single int argument, say m.
If this function Object() { [native code] } throws an error, the function should return "Tornado: m miles distant; and approaching!"
Chapter 10 Solutions
Starting Out with Java: Early Objects (6th Edition)
Ch. 10.1 - Prob. 10.1CPCh. 10.1 - Prob. 10.2CPCh. 10.1 - Prob. 10.3CPCh. 10.1 - Prob. 10.4CPCh. 10.1 - Prob. 10.5CPCh. 10.1 - Prob. 10.6CPCh. 10.1 - Prob. 10.7CPCh. 10.1 - Prob. 10.8CPCh. 10.1 - Prob. 10.9CPCh. 10.1 - When does the code in a finally block execute?
Ch. 10.1 - What is the call stack? What is a stack trace?Ch. 10.1 - Prob. 10.12CPCh. 10.1 - Prob. 10.13CPCh. 10.1 - Prob. 10.14CPCh. 10.2 - What does the throw statement do?Ch. 10.2 - Prob. 10.16CPCh. 10.2 - Prob. 10.17CPCh. 10.2 - Prob. 10.18CPCh. 10.2 - Prob. 10.19CPCh. 10.3 - What is the difference between a text file and a...Ch. 10.3 - What classes do you use to write output to a...Ch. 10.3 - Prob. 10.22CPCh. 10.3 - What class do you use to work with random access...Ch. 10.3 - What are the two modes that a random access file...Ch. 10.3 - Prob. 10.25CPCh. 10 - Prob. 1MCCh. 10 - Prob. 2MCCh. 10 - Prob. 3MCCh. 10 - Prob. 4MCCh. 10 - FileNotFoundException inherits from __________. a....Ch. 10 - Prob. 6MCCh. 10 - Prob. 7MCCh. 10 - Prob. 8MCCh. 10 - Prob. 9MCCh. 10 - Prob. 10MCCh. 10 - Prob. 11MCCh. 10 - Prob. 12MCCh. 10 - Prob. 13MCCh. 10 - Prob. 14MCCh. 10 - Prob. 15MCCh. 10 - This is the process of converting an object to a...Ch. 10 - Prob. 17TFCh. 10 - Prob. 18TFCh. 10 - Prob. 19TFCh. 10 - True or False: You cannot have more than one catch...Ch. 10 - Prob. 21TFCh. 10 - Prob. 22TFCh. 10 - Prob. 23TFCh. 10 - Prob. 24TFCh. 10 - Find the error in each of the following code...Ch. 10 - // Assume inputFile references a Scanner object,...Ch. 10 - Prob. 3FTECh. 10 - Prob. 1AWCh. 10 - Prob. 2AWCh. 10 - Prob. 3AWCh. 10 - Prob. 4AWCh. 10 - Prob. 5AWCh. 10 - Prob. 6AWCh. 10 - The method getValueFromFile is public and returns...Ch. 10 - Prob. 8AWCh. 10 - Write a statement that creates an object that can...Ch. 10 - Assume that the reference variable r refers to a...Ch. 10 - Prob. 1SACh. 10 - Prob. 2SACh. 10 - Prob. 3SACh. 10 - Prob. 4SACh. 10 - Prob. 5SACh. 10 - Prob. 6SACh. 10 - What types of objects can be thrown?Ch. 10 - Prob. 8SACh. 10 - Prob. 9SACh. 10 - Prob. 10SACh. 10 - What is the difference between a text file and a...Ch. 10 - What is the difference between a sequential access...Ch. 10 - What happens when you serialize an object? What...Ch. 10 - TestScores Class Write a class named TestScores....Ch. 10 - Prob. 2PCCh. 10 - Prob. 3PCCh. 10 - Prob. 4PCCh. 10 - Prob. 5PCCh. 10 - FileArray Class Design a class that has a static...Ch. 10 - File Encryption Filter File encryption is the...Ch. 10 - File Decryption Filter Write a program that...Ch. 10 - TestScores Modification for Serialization Modify...Ch. 10 - Prob. 11PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Define an exception class called CoreBreachException. The class should have a default constructor. If an exception is thrown using this zero- argument constructor, getMessage should return "Core Breach! Evacuate Ship!" The class should also define a constructor having a single parameter of type String. If an exception is thrown using this constructor, getMessage should return the value that was used as an argument to the constructor.arrow_forwardWrite a Month class that holds information about the month. Write exception classes for the following error conditions:• A number less than 1 or greater than 12 is given for the month number.• An invalid string is given for the name of the month.Modify the Month class so that it throws the appropriate exception when either of these errors occurs. Demonstrate the classes in a program.arrow_forwardC++ D.S. Malik Exercise 14-4 Write a program that prompts the user to enter time in 12-hour notation. The program then outputs the time in 24-hour notation. Your program must contain three exception classes: invalidHr, invalidMin, and invalidSec. If the user enters an invalid value for hours, then the program should throw and catch an invalidHr object. Follow similar conventions for the invalid values of minutes and seconds.arrow_forward
- The following class maintains an account balance and returns aspecial error code.public class Account{private double balance;// returns new balance or -1 if errorpublic double deposit(double amount){if (amount > 0)balance += amount;elsereturn -1; // Code indicating errorreturn balance;}}Rewrite the class so that it throws appropriate exception instead ofreturning -1 as an error code. Write test code that attempts to depositinvalid amounts and catches the exceptions that are thrownarrow_forward(Please use JAVA) Create custom exception and implement exception handling Create the following classes based on the UML Class diagram: - Employee - InvalidUserDetailException - EmployeeValidation-uses the custom InvalidUserDetailException class. This class contains an instancemethod validateInput() which will validate the employee details (name, age & gender) based on the specified criteria. * If employee details are within the criteria, employee details will be outputted in the console. * If employee details are not within the criteria, InvalidUserDetailException will be thrown with a message informing user that employee detail inputted is invalid. Create Employee Main class which will contain the main() method main() method will allow user to input employee name, age and gender. Calls validateInput() to validate the employee details.arrow_forwardModify the attached code: Create a new class called CalculatorWithMod. This class should be a sub class of the base class Calculator. This class should also have an additional method for calculating the modulo. The modulo method should only be seen at the sub class, and not the base class! Include exception handling for instances when dividing by 0 or calculating the modulo with 0. You would need to use throw, try, and catch. The modulo (or "modulus" or "mod") is the remainder after dividing one number by another.Example: 20 mod 3 equals 2Because 20/3 = 6 with a remainder of 2 Refer also to the attached sample outputs:arrow_forward
- ArgumentException is an existing class that derives from Exception; you use it when one or more of a method’s arguments do not fall within an expected range. Write a C# application SwimmingWaterTemperature containing a variable that can hold a temperature expressed in degrees Fahrenheit. Within the class, create a method that accepts a parameter for a water temperature and returns true or false, indicating whether the water temperature is between 70 and 85 degrees and thus comfortable for swimming. If the temperature is not between 32 and 212 (the freezing and boiling points of water), it is invalid, and the method should throw an ArgumentException. In the Main() method, continuously prompt the user for data temperature, pass it to the method, and then display the following messages indicating whether the temperature is comfortable, not comfortable, or invalid: X degrees is comfortable for swimming. X degrees is not comfortable for swimming. Value does not fall within the expected…arrow_forwardArgumentException is an existing class that derives from Exception; you use it when one or more of a method’s arguments do not fall within an expected range. Write the application SwimmingWaterTemperature containing a variable that can hold a temperature expressed in degrees Fahrenheit. Within the class, create a method that accepts a parameter for a water temperature and returns true or false, indicating whether the water temperature is between 70 and 85 degrees and thus comfortable for swimming. If the temperature is not between 32 and 212 (the freezing and boiling points of water), it is invalid, and the method should throw an ArgumentException. In the Main() method, continuously prompt the user for data temperature, pass it to the method, and then display the following messages indicating whether the temperature is comfortable, not comfortable, or invalid: X degrees is comfortable for swimming. X degrees is not comfortable for swimming. Value does not fall within the expected…arrow_forwardArgumentException is an existing class that derives from Exception; you use it when one or more of a method’s arguments do not fall within an expected range. Write the application SwimmingWaterTemperature containing a variable that can hold a temperature expressed in degrees Fahrenheit. Within the class, create a method that accepts a parameter for a water temperature and returns true or false, indicating whether the water temperature is between 70 and 85 degrees and thus comfortable for swimming. If the temperature is not between 32 and 212 (the freezing and boiling points of water), it is invalid, and the method should throw an ArgumentException. In the Main() method, continuously prompt the user for data temperature, pass it to the method, and then display the following messages indicating whether the temperature is comfortable, not comfortable, or invalid: X degrees is comfortable for swimming. X degrees is not comfortable for swimming. Value does not fall within the expected…arrow_forward
- Develop an exception class named InvalidMonthException that extends the Exception class. Instances of the class will be thrown based on the following conditions: The value for a month number is not between 1 and 12 The value for a month name is not January, February, March, … December Develop a class named Month. The class should define an integer field named monthNumber that holds the number of a month. For example, January would be 1, February would be 2, and so forth. In addition, provide the following methods: A no-argument constructor that sets the monthNumberto 1. An overloaded constructor that accepts the number of the month as an argument. The constructor should set the monthNumberfield to the parameter value if the parameter contains the value 1 – 12. Otherwise, throw an InvalidMonthException exception back to the caller. The exception should note that the month number was incorrect. An overloaded constructor that accepts a string containing the name of the month,…arrow_forwardJava Problem:Create a Class named Bishop. Make sure the class cannot be inherited. If there have already been 5 objects initiated for this class, trying to initiate the 6th object, the constructor will throw a user-defined exception BishopCreationLimitExceeded. This class has an instance method named printObjectNumber which will print the object creation sequence number for the object for which you are calling the method as follows: This Bishop Object number is 3. Here, 3 is the object creation sequence number that is the third call to new Bishop() in your code that initiated the object. The BishopCreationLimitExceeded class sets the exception message (using super call in the constructor) as follows: The maximum number of bishop objects can be 5. You can define instance variables in the Bishop class if you need them.arrow_forwardQ1 (Creating Custom Exception Class) Write a Password class that stores a password string. Passwords must contain at least seven alpha-numeric characters, with at least one letter and one digit. Also write an InvalidPasswordException class that extends Exception. The Password constructor should throw an InvalidPasswordException, which should report an appropriate message based on a given string (e.g. if the string contains no digits, the exception should report this fact). Write a GUI program to prompt the user to enter a password and report an InvalidPasswordException if an invalid password is entered. Please teach and help me how to deisgn the program based on the two files given .Some sample screenshots are shown below: InvalidPasswordException.java public class InvalidPasswordException extends Exception{ public InvalidPasswordException (String errMsg){ super(errMsg); } } Password.java public class Password {private String passwordstr; public…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
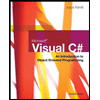
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,