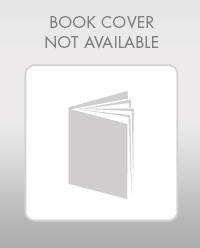
Indirect Sorting Through Pointers #1
Consider a company that needs to sort an array Person data[10] of structures of type Person by name.
struct Person
{
string name;
int age;
}
In real life the Person structures may have many members and occupy a large area of memory, making it computationally expensive to move Person objects around while sorting. You can define an auxiliary array Person *pData [10], setting each entry of pData[k] to point to the corresponding entry of data[k]. Write a

Want to see the full answer?
Check out a sample textbook solution
Chapter 10 Solutions
Starting Out With C++: Early Objects (10th Edition)
Additional Engineering Textbook Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Starting Out with Java: From Control Structures through Objects (6th Edition)
C++ How to Program (10th Edition)
Introduction To Programming Using Visual Basic (11th Edition)
Absolute Java (6th Edition)
Computer Systems: A Programmer's Perspective (3rd Edition)
- (Statistics) a. Write a C++ program that reads a list of double-precision grades from the keyboard into an array named grade. The grades are to be counted as they’re read, and entry is to be terminated when a negative value has been entered. After all grades have been input, your program should find and display the sum and average of the grades. The grades should then be listed with an asterisk (*) placed in front of each grade that’s below the average. b. Extend the program written for Exercise 1a to display each grade and its letter equivalent, using the following scale: Between90and100=AGreaterthanorequalto80andlessthan90=BGreaterthanorequalto70andlessthan80=CGreaterthanorequalto60andlessthan70=DLessthan60=Farrow_forwardCreat a code in c++ using s class compute array By using pointer notation, write a program that have three local arrays of same size and float type in the main () function. And consider that the values of first two are already initialized. To takethe addresses of all the arrays as arguments, write the function named ComputeArrays () that adds the contents of the first two arrays and before returning that store the results in the third array. The fourth argument to this function can store the size of the arrays.arrow_forwardTic-Tac-Toe) Write a program that allows two players to play the tic-tac-toe game. Your program must contain the class ticTacToe to implement a ticTacToe object. Include a 3-by-3 two-dimensional array, as a private member variable, to create the board. If needed, include additional member variables. Some of the operations on a ticTacToe object are printing the current board, getting a move, checking if a move is valid, and determining the winner after each move. Add additional operations as needed. (programming language C#)arrow_forward
- Revise the following Course class implementation in the following c++ code When adding a new student to the course, if the array capacity is exceeded, increase the array size by creating a new larger array and copying the contents of the current array to it. Implement the dropStudent function. Add a new function named clear() that removes all students from the course. Implement the destructor and copy constructor to perform a deep copy in the class. Write a test program that creates a course, adds three students, removes one, and displays the students in the course.arrow_forwardProject 1: MyString The purpose of this assignment is to practice: ▪Implementing a sequential abstract data type that uses a dynamic array structure ▪Analyzing and comparing algorithms for efficiency using Big-O notation This assignment reinforces the following competency: Apply the fundamentals of mathematics in computer science disciplines. For this assignment, implement a version of the string class. Design and implement a MyString class defined by the following data: ▪A char array reference (or pointer) for the array of characters that make up the string ▪An integer curr_length representing the number of characters in the string ▪(C++ only) An integer capacity that represents the size of the array Add the following methods to your class: ▪A constructor that initializes the array to null and the curr_length to 0 ▪A constructor that takes a String parameter and initializes the char array to the characters in the String. curr_length should be appropriately initialized. ▪a copy…arrow_forward5.12 LAB: User-Defined Functions: Adjust list by normalizing CORAL LANGUAGE ONLY PLEASE When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. Define a function named getMinimumInt that takes an integer array as a parameter and returns the smallest value. Then write a main program that reads five integers as input, stores the integers in an array, and calls getMinimumInt() with the array as an argument. The main program then outputs the normalized array by subtracting the returned smallest value from all the values in the array. Ex: If the input is: 30 50 10 70 65 function getMinimumInt() returns 10, and the program output is: 20 40 0 60 55 For coding simplicity, follow every output value by a space, even the last one. Your program should define and use a function:Function getMinimumInt(integer array(?) userVals) returns integer minInt…arrow_forward
- Part 1 Write a function that takes an integer array and the size of the array The array is supposed to have 'N' integers in it, where N-1 integers are repeated even number of times and one integer is repeated odd number of times For example array = {1,5,5, 1,2,5,2,2,5}. 1 and 5 are repeated even number of times whereas 2 is odd number times. The function should find the element which is repeated odd number of times and return it. In the case of the example shown above, the function should return 2. int FindSingleElement(int arr[], int size) { } int main(int argc, char *argv[]) { return 0; } THIS IN C++.arrow_forwardC code Blocks Write the complete function that receives a pointer to the array's first element as an argument and multiplies the third element of the array by 10. Define your function in the same way as the given function prototype. Take a look at the "For example" below to see how the function is used in the main function and the expected result. #include <stdio.h>#include <stdlib.h>void multiplyElement(int *ptr);int main(){ int array[5] = {1,2,3,4,5}; int *arrPtr = NULL; arrPtr = &array[0]; multiplyElement(arrPtr); for(int i = 0; i < 5; i++){ printf("%d ", array[i]); }return 0;}//Your answer starts here For example: Test Result int array[5] = {1,2,3,4,5}; int *arrPtr = NULL; arrPtr = &array[0]; multiplyElement(arrPtr); for(int i = 0; i < 5; i++){ printf("%d ", array[i]); } 1 2 30 4arrow_forwardArray Challenge 5. Write a program in C# to count a total number of duplicate elements in an array.Test Data :Input the number of elements to be stored in the array :3Input 3 elements in the array :element - 0 : 5element - 1 : 1element - 2 : 1Expected Output :Total number of duplicate elements found in the array is : 1arrow_forward
- Q 1) Write a class with name Array. This class has an array which should be initialized by user. Create a function with name sumFind in this class with working logic as sum of all elements of an array, after finding sum, if sum is even calculate factorial otherwise display only sum. ( Note::: subject::c# language )arrow_forward1- Define a struct type that contains a person’s name consisting of a first name and a second name, plus the person’s phone number. Use this struct in a program that will allow one or more names and corresponding numbers to be entered and will store the entries in an array of structures. The program should allow a second name to be entered and output all the numbers corresponding to the name, and optionally output all the names with their corresponding numbers. *'Keep the program as simple as possible using the basic logic of struct the following images attached are just for some reference of what simple basic logic means in my words & also supposed to be applied when solving **single pointers & 2d arrays can be usedarrow_forwardDevelop a function that accepts an array and returns true if the array contains any duplicate values or false if none of the values are repeated. Develop a function that returns true if the elements are in decreasing order and false otherwise. A “peak” is a value in an array that is preceded and followed by a strictly lower value. For example, in the array {2, 12, 9, 8, 5, 7, 3, 9} the values 12 and 7 are peaks. Develop a function that returns the number of peaks in an array of integers. Note that the first element does not have a preceding element and the last element is not followed by anything, so neither the first nor last elements can be peaksarrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
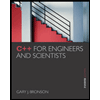
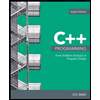