STARTING OUT WITH C++FROM CONTROL STRU
18th Edition
ISBN: 9781323815458
Author: GADDIS
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 10, Problem 3PC
Program Plan Intro
Word Counter
Program Plan:
- Include the required header files to the program.
- Define function prototype which is used in the program.
- Define the “main()” function.
- Declare the required variables.
- Get the input c-string from the user and call the function “word_count”.
- Print the c-string words count.
- Get the input string object from the user and call the function “word_count”.
- Print the string object words count.
- Define the “word_count” function.
- Declare the variable.
- The “while” loop is used to count the number of words in that string.
- The “while” loop is used to ignore the whitespaces.
- The “if” condition is used to count the words.
- The “while” loop is used to move to next word.
- Finally return the words count to the main function.
- Define the “word_count” function.
- Declare the variable.
- Get the length of the string.
- The “for” loop is used to count the number of words in that string object.
- The “if” condition is used to ignore the whitespaces. Otherwise it is used count the words.
- The “if” condition is used to determine the words.
- Finally return the words count to the main function.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Exercise 1:
Word Separator
Write a program that accepts as input a sentence in which all of the words are run together, but the first character of each word is uppercase. Convert the sentence to a string in which the words are separated by spaces and only the first word starts with an uppercase letter. For example the string StopAndSmellTheRoses. would be converted to “Stop and smell the roses.”
Exercise 2:
replaceSubstring Function
Write a function named replaceSubstring. The function should accept three string object arguments. Let’s call them string1, string2, and string3. It should search string1 for all occurrences of string2. When it finds an occurrence of string2, it should replace it with string3. For example, suppose the three arguments have the following values:
string1: “the dog jumped over the fence”
string2: “the”
string3: “that”
With these three arguments, the function would return a string object with the value
“that dog jumped over that fence.” Demonstrate the…
(C++) 9. True/False: the strcpy() function will make sure there is enough memory allocated in the destination string before copying C-strings
10. True/False: when creating a string object, you must dynamically allocate enough bytes to hold the string
11. Consider the following statement, assuming goAgain is a valid char. Rewrite it using toupper() or tolower()
if (goAgain == 'y' || goAgain == 'Y')
12. Write a C++ function which accepts a pointer to a C-string as its argument. It should return the number of words in the C-string. For example, for the C-string “The Giants won the pennant!” your function should return 5. You may assume the parameter passed is a pointer to a valid, null-terminated C-string with no newlines or tabs, exactly one space separates each word, and there is at least one word.
int wordCounter(char* str)
def swap_text(text):
Backstory:
Luffy wants to organize a surprise party for his friend Zoro and he wants to send a message to his friends, but he wants to encrypt the message so that Zoro cannot easily read it. The message is encrypted by exchanging pairs of characters.
Description: This function gets a text (string) and creates a new text by swapping each pair of characters, and returns a string with the modified text. For example, suppose the text has 6 characters, then it swaps the first with the second, the third with the fourth and the fifth with the sixth character.
Parameters: text is a string (its length could be 0)Return value: A string that is generated by swapping pairs of characters. Note that if the
Examples:
swap_text ("hello") swap_text ("Party for Zoro!") swap_text ("") def which_day(numbers):
→ 'ehllo'→ 'aPtr yof roZor!' → ''
length of the text is odd, the last character remains in the same position.
Chapter 10 Solutions
STARTING OUT WITH C++FROM CONTROL STRU
Ch. 10.2 - Write a short description of each of the following...Ch. 10.2 - Prob. 10.2CPCh. 10.2 - Write an if statement that will display the word...Ch. 10.2 - What is the output of the following statement?...Ch. 10.2 - Write a loop that asks the user Do you want to...Ch. 10.4 - Write a short description of each of the following...Ch. 10.4 - Prob. 10.7CPCh. 10.4 - Prob. 10.8CPCh. 10.4 - Prob. 10.9CPCh. 10.4 - When complete, the following program skeleton will...
Ch. 10.5 - Write a short description of each of the following...Ch. 10.5 - Write a statement that will convert the string 10...Ch. 10.5 - Prob. 10.13CPCh. 10.5 - Write a statement that will convert the string...Ch. 10.5 - Write a statement that will convert the integer...Ch. 10.6 - Prob. 10.16CPCh. 10 - Prob. 1RQECh. 10 - Prob. 2RQECh. 10 - Prob. 3RQECh. 10 - Prob. 4RQECh. 10 - Prob. 5RQECh. 10 - Prob. 6RQECh. 10 - Prob. 7RQECh. 10 - Prob. 8RQECh. 10 - Prob. 9RQECh. 10 - Prob. 10RQECh. 10 - The __________ function returns true if the...Ch. 10 - Prob. 12RQECh. 10 - Prob. 13RQECh. 10 - The __________ function returns the lowercase...Ch. 10 - The _________ file must be included in a program...Ch. 10 - Prob. 16RQECh. 10 - Prob. 17RQECh. 10 - Prob. 18RQECh. 10 - Prob. 19RQECh. 10 - Prob. 20RQECh. 10 - Prob. 21RQECh. 10 - Prob. 22RQECh. 10 - Prob. 23RQECh. 10 - Prob. 24RQECh. 10 - The ________ function returns the value of a...Ch. 10 - Prob. 26RQECh. 10 - The following if statement determines whether...Ch. 10 - Assume input is a char array holding a C-string....Ch. 10 - Look at the following array definition: char...Ch. 10 - Prob. 30RQECh. 10 - Write a function that accepts a pointer to a...Ch. 10 - Prob. 32RQECh. 10 - Prob. 33RQECh. 10 - T F If touppers argument is already uppercase, it...Ch. 10 - T F If tolowers argument is already lowercase, it...Ch. 10 - T F The strlen function returns the size of the...Ch. 10 - Prob. 37RQECh. 10 - T F C-string-handling functions accept as...Ch. 10 - T F The strcat function checks to make sure the...Ch. 10 - Prob. 40RQECh. 10 - T F The strcpy function performs no bounds...Ch. 10 - T F There is no difference between 847 and 847.Ch. 10 - Prob. 43RQECh. 10 - char numeric[5]; int x = 123; numeri c = atoi(x);Ch. 10 - char string1[] = "Billy"; char string2[] = " Bob...Ch. 10 - Prob. 46RQECh. 10 - Prob. 1PCCh. 10 - Prob. 2PCCh. 10 - Prob. 3PCCh. 10 - Average Number of Letters Modify the program you...Ch. 10 - Prob. 5PCCh. 10 - Prob. 6PCCh. 10 - Name Arranger Write a program that asks for the...Ch. 10 - Prob. 8PCCh. 10 - Prob. 9PCCh. 10 - Prob. 10PCCh. 10 - Prob. 11PCCh. 10 - Password Verifier Imagine you are developing a...Ch. 10 - Prob. 13PCCh. 10 - Word Separator Write a program that accepts as...Ch. 10 - Character Analysis If you have downloaded this...Ch. 10 - Prob. 16PCCh. 10 - Prob. 17PCCh. 10 - Prob. 18PCCh. 10 - Check Writer Write a program that displays a...
Knowledge Booster
Similar questions
- IN C Programming Language...THANKS Write a function that replaces a given string with ‘*’ within a given text if that string is a full word, or the beginning or end of a word. Test your function with a suitable main.arrow_forward4. Complete the function show_upper. This function takes one parameter - a string (s). It should return a string made up of all the upper-case characters in s. For example, if s is “aBdDEfgHijK” then show_upper should return “BDEHK”. It should return the upper-case string - not print it. Do not change anything outside show_upper.arrow_forwardComplete the check_character() function which has 2 parameters: A string, and a specified index. The function checks the character at the specified index of the string parameter, and returns a string based on the type of character at that location indicating if the character is a letter, digit, whitespace, or unknown character. Ex: The function calls below with the given arguments will return the following strings: check_character('happy birthday', 2) returns "Character 'p' is a letter"check_character('happy birthday', 5) returns "Character ' ' is a white space"check_character('happy birthday 2 you', 15) returns "Character '2' is a digit"check_character('happy birthday!', 14) returns "Character '!' is unknown" use python please def check_character(word, index): # Type your code here. if __name__ == '__main__': print(check_character('happy birthday', 2)) print(check_character('happy birthday', 5)) print(check_character('happy birthday 2 you', 15))…arrow_forward
- Write a C++ program using C-Strings 4. This function returns the index in string s where the substring can first be found. For example if s is “Skyscraper” and substring is “ysc” the function would return 2. It should return -1 if the substring does not appear in the string. int findSubstring(char *s, char substring[]) 5. This function returns true if the argument string is a palindrome. It returns false if it is not. A palindrome is a string that is spelled the same as its reverse. For example “abba” is a palindrome. So is “hannah”, “abc cba”, and “radar”. bool isPalindrome(char *s) Note: do not get confused by white space characters. They should not get any special treatment. “abc ba” is not a palindrome. It is not identical to its reverse. 6) This function should reverse the words in a string. A word can be considered to be any characters, including punctuation, separated by spaces (only spaces, not tabs, \n etc.). So, for example, if s is “The Giants won the Pennant!”…arrow_forward10.17: Morse Code Converter C++Morse code is a code where each letter of the English alphabet, each digit, and various punctuation characters are represented by a series of dots and dashes. Table 10-8 from the textbook shows part of the code. Write a program that asks the user to enter a string, and then converts that string to Morse code. Note that Morse code represents both upper and lower case letters so that both 'A' and 'a' will be converted to ".-". Input Validation.None. Instructor Notes: Note: You can use the Morse table in the book or this site (space isn't listed, but it is just mapped to a space). You will most certainly need to create two parallel arrays to map the characters to the Morse code strings.arrow_forwardComputer Science ****Please Write a program CODE in C 2. Write a function which takes a string of any length and returns the number of times the letter a appears in the string. Write the main program, which prompts the user to enter a sentence (up to 100 characters) and uses the function to find the number of times a occurs in the sentence. Print the result.arrow_forward
- Write a function that counts the occurrences of a word in a string. Thefunction should return an integer. Do not assume that just one space separates words and a string can contain punctuation. Write the function sothat it works with either a String argument or a StringBuilder object.arrow_forwardC++ Code: DNA Sequence The main() function is already written for you. You will implement the function int numOccurrences(string& STR, string& sequence). Without even understanding what functions do in C++, all you need to know, at this point, is that you have access to the string STR of which you have to find the length of the largest consecutive occurrence in the string sequence. For example, if input sequence is: AGACGGGTTACCATGACTATCTATCTATCTATCTATCTATCTATCTATCACGTACGTACGTATCGAGATAGATAGATAGATAGATCCTCGACTTCGATCGCAATGAATGCCAATAGACAAAA then numOccurrences("AGAT", sequence) should return 5 numOccurrences("TATC", sequence) should return 8 if input sequence is: AACCCTGCGCGCGCGCGATCTATCTATCTATCTATCCAGCATTAGCTAGCATCAAGATAGATAGATGAATTTCGAAATGAATGAATGAATGAATGAATGAATG then numOccurrences("AATG", sequence) should return 7 numOccurrences("TATC", sequence) should return 4 if input sequence is:…arrow_forward//the language is c++ Implement function to tokenize a string. std::vector<std::string> tokenize(std::string& line) { } int main(){ std::string ts = "This is a string with no commas semicolons or fullstops"; std::vector<std::string> tok = tokenize(ts); for (auto x : tok) { std::cout<<x<<std::endl; } return 0; }arrow_forward
- Vowels and Consonants:Write a program with a function that accepts a string as an argument and returns thenumber of vowels that the string contains. The application should have another function that Programming Exercises 369 VideoNote The Vowels and Consonants problem 370 Chapter 9 More About Strings accepts a string as an argument and returns the number of consonants that the string contains. The application should let the user enter a string and should display the number of vowels and the number of consonants it contains. *python codimgarrow_forwardCode is in C++ Instructions Write a program that reads in a line consisting of a student’s name, Social Security number, user ID, and password. The program outputs the string in which all the digits of the Social Security number and all the characters in the password are replaced by x. (The Social Security number is in the form 000-00-0000, and the user ID and the password do not contain any spaces.) Your program should not use the operator [] to access a string element. Input is as follows highlighted in bold John Doe 333224444 DoeJ 123Password My problem is with my output, i am close with the code, but i have attached what happens on my output and i cannot figure out why? You can see how it prints out multiple times but i am lost? The terminal image is also attached. Thank you! Here is the code: #include <iostream> //include statement(s)#include <iomanip>#include <string> using namespace std; //using namespace statement(s) void getInfo(string info); //void…arrow_forwardC Programming Language Write a function that takes 2 strings, word1 and word2 as parameters. The function must return if,starting from word1, by only deleting some characters you can get word2.Example: word1: pineapple and word2:nap result: true; word1: orange, word2: one; results: true;word1: cyberspace; word2: peace, result: falsearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
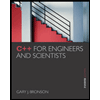
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
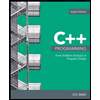
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning