Concept explainers
Sections 11.2–11.4
11.1 (The Triangle class) Design a class named Triangle that extends GeometricObject. The class contains:
■ Three double data fields named side1, side2, and side3 with default values 1.0 to denote three sides of a triangle.
■ A no-arg constructor that creates a default triangle.
■ A constructor that creates a triangle with the specified side1, side2, and side3.
■ The accessor methods for all three data fields.
■ A method named getArea() that returns the area of this triangle.
■ A method named getPerimeter() that returns the perimeter of this triangle.
■ A method named toString() that returns a string description for the triangle.
For the formula to compute the area of a triangle, see
return "Triangle: side1 = " + side1 + " side2 = " + side2 + " side3 = " + side3;
Draw the UML diagrams for the classes Triangle and GeometricObject and implement the classes. Write a test program that prompts the user to enter three sides of the triangle, a color, and a Boolean value to indicate whether the triangle is filled. The program should create a Triangle object with these sides and set the color and filled properties using the input. The program should display the area, perimeter, color, and true or false to indicate whether it is filled or not.

Program Plan:
- Include the required import statement.
- Define the main class.
- Define the main method using public static main.
- Declare the input scanner.
- Get the three sides of the triangle from the user.
- Create an object for the “Triangle” class.
- Get the color from the user and call the “setColor” method with the parameter “color”.
- Get the Boolean value for filled the triangle and call the “setFilled” method with the parameter “filled”.
- Display the output.
- Define the main method using public static main.
- Define the “GeometricObject” class.
- Declare the required variables.
- Define the default constructor and constructor for the class.
- Define the accessor and matator.
- The “isFilled” and “setColor” method will return the value to the main class.
- Define the derived class “Triangle” from the “GeometricObject” class.
- Declare the required variables.
- Define the default constructor and constructor for the class.
- Define the accessor.
- The “getArea()” method will calculate the area of triangle and then return the result.
- The “getPerimeter()” method will return the perimeter of the triangle.
- The “toString()” method will return the three sides of the triangle.
The below program is used to display the area, perimeter and sides of the triangle as follows:
Explanation of Solution
Program:
//import statement
import java.util.Scanner;
//class Excersise11_01
public class Exercise11_01
{
// main method
public static void main(String[] args)
{
// declare the scanner variable
Scanner input = new Scanner(System.in);
//get the input from the user
System.out.print("Enter three sides: ");
//declare the variables
double side1 = input.nextDouble();
double side2 = input.nextDouble();
double side3 = input.nextDouble();
//create an object for the "Triangle" class
Triangle triangle = new Triangle(side1, side2, side3);
//get the input from the user
System.out.print("Enter the color: ");
String color = input.next();
//call the "setColor" function
triangle.setColor(color);
//get the input from the user
System.out.print("Enter a boolean value for filled: ");
boolean filled = input.nextBoolean();
//call the "setFilled" function
triangle.setFilled(filled);
//print the output
System.out.println("The area is " + triangle.getArea());
System.out.println("The perimeter is "
+ triangle.getPerimeter());
System.out.println(triangle);
}
}
//definition of class "GeometricObject"
class GeometricObject
{
/* declare the required variables and initialize it */
private String color = "white";
private boolean filled;
private java.util.Date dateCreated;
//definition of default constructor
public GeometricObject()
{
//create an object
dateCreated = new java.util.Date();
}
//definition of constructor
public GeometricObject(String color, boolean filled)
{
//create an object
dateCreated = new java.util.Date();
//set the value
this.color = color;
this.filled = filled;
}
//definition of accessor
public String getColor()
{
//return the color
return color;
}
//definition of mutator
public void setColor (String color)
{
//set the color
this.color = color;
}
//definition of the "isFilled" method
public boolean isFilled()
{
//return the value
return filled;
}
//definition of the "setFilled" method
public void setFilled(boolean filled)
{
//set the value
this.filled = filled;
}
//definition of the "getDateCreated" method
public java.util.Date getDateCreated()
{
//return the value
return dateCreated;
}
//definition of the "toString" method
public String toString()
{
//return the value
return "created on " + dateCreated + "\ncolor: " + color + " and filled: " + filled;
}
}
//definition of derived class "Triangle"
class Triangle extends GeometricObject
{
/* declare the required variables and initialize it */
private double side1 = 1.0, side2 = 1.0, side3 = 1.0;
/*definition of Constructor */
public Triangle()
{
}
/* definition of Constructor */
public Triangle(double side1, double side2, double side3)
{
this.side1 = side1;
this.side2 = side2;
this.side3 = side3;
}
//definition of accessor
public double getSide1()
{
//return the value
return side1;
}
//definition of accessor
public double getSide2()
{
//return the value
return side2;
}
//definition of accessor
public double getSide3()
{
//return the value
return side3;
}
/*override method of "getArea" in GeometricObject */
public double getArea()
{
//declare and calculate the value
double s = (side1 + side2 + side3) / 2;
//return the value
return Math.sqrt(s * (s - side1) * (s - side2) * (s - side3));
}
/*override method of "getPerimeter" in GeometricObject */
public double getPerimeter()
{
//return the value
return side1 + side2 + side3;
}
//definition of "toString" method
public String toString()
{
// return the three sides
return "Triangle: side1 = " + side1 + " side2 = " + side2 +" side3 = " + side3;
}
}
UML diagram:
Explanation:
The above UML diagram the “GeometricObject” is a parent class which contains “color”, “filled”, “dateCreated” variables and “GeometricObject()”, “GeometricObject(String color, boolean filled)”, “getColor()”, “getColor(String color)”, “isFilled()”, “setFilled(boolean filled)”, “getDateCreated()” and “toString()” methods.
The “Triangle” is the child class extended from “GeometricObject” class it contains “side1”, “side2” and “side3” variables and “Triangle()”, “Triangle(double side1, double side2, double side3)”, “getSide1()”, “getSide2()”, “getSide3()”, “getArea()”, “getPerimeter()” and “toString()” methods.
Enter three sides: 2
3
4
Enter the color: black
Enter a boolean value for filled: true
The area is 2.9047375096555625
The perimeter is 9.0
Triangle: side1 = 2.0 side2 = 3.0 side3 = 4.0
Want to see more full solutions like this?
Chapter 11 Solutions
INTRO TO JAVA PROGRAMMING: COMPREHENSIV
Additional Engineering Textbook Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
Digital Fundamentals (11th Edition)
Concepts of Programming Languages (11th Edition)
Starting out with Visual C# (4th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
- 23.6 LAB: BankAccount class (Use Python) Build a class called BankAccount that manages checking and savings accounts. The class has three attributes: a customer name, the customer's savings account balance, and the customer's checking account balance. Implement the following constructor and instance methods as listed below: Constructor with parameters (self, new_name, checking_balance, savings_balance) - set the customer name to parameter new_name, set the checking account balance to parameter checking_balance, and set the savings account balance to parameter savings_balance. deposit_checking(self, amount) - add parameter amount to the checking account balance (only if positive) deposit_savings(self, amount) - add parameter amount to the savings account balance (only if positive) withdraw_checking(self, amount) - subtract parameter amount from the checking account balance (only if positive) withdraw_savings(self, amount) - subtract parameter amount from the savings account…arrow_forward9.19 LAB: BankAccount class (EO) Build a class called BankAccount that manages checking and savings accounts. The class has three private member fields: a customer name (String), the customer's savings account balance (double), and the customer's checking account balance (double). Implement the following Constructor and instance methods: public BankAccount(String newName, double amt1, double amt2) - set the customer name to parameter newName, set the checking account balance to parameter amt1 and set the savings account balance to parameter amt2. (amt stands for amount) public void setName(String newName) - set the customer name public String getName() - return the customer name public void setChecking(double amt) - set the checking account balance to parameter amt public double getChecking() - return the checking account balance public void setSavings(double amt) - set the savings account balance to parameter amt public double getSavings() - return the savings account balance…arrow_forwarda package ParentPackage, define a class Code Tantra with the instance variables-student name, registration number, a float array of length 7 (to store the marks scored in 7 questions in TermEnd exam), total marks and percentage. Define a constructor that receives the name, registration number and an array of 7 marks and adds the top 5 marks and stores the sum in total marks in the same package, define a class Percentageclass with a method to calculate the percentage Define a subpackage ChildPackage" with a class GradeClass that inherits the PercentageClass and includes a method to print the count of S. A. B, C D E and 'F' grades, based on the percentage obtained(Java Code)arrow_forward
- 6.1 LAB: Pet information (derived classes)The base class Pet has protected fields petName, and petAge. The derived class Cat extends the Pet class and includes a private field for catBreed. Complete main() to: create a generic pet and print information using printInfo().create a Cat pet, use printInfo() to print information, and add a statement to print the cat's breed using the getBreed() method.Ex. If the input is: Dobby2Kreacher3Scottish Foldthe output is: Pet Information:Name: DobbyAge: 2Pet Information:Name: KreacherAge: 3Breed: Scottish Foldarrow_forwardCreate a class named Line: (a) Define private data members p1 and p2 as pointer to Point objects (the one we had in lectures), slope and length as double variables. (b) Define setter and getter functions. (c) Define a null-constructor that initializes numeric variables with zero and allocate dynamic memory for points and initialize them to [0,0] as well. (d) Overload a constructor that allocates memory for points, initilize them with given arguments, and calculate the slope and length. (e) Implement destructor, copy constructor and copy assignment operator. (f) Create a function called ”parallel” that takes too Line objects, returns true when given lines are parallel and returns false otherwise. (g) Overload the less than (<) and greater than (>) and equality (==) operators (compare the length). (h) Write a functions that reads lines in the format provided in the lines.txt from the file (without any change) and stores them in a vector named Lines. (i) Sort the objects of Lines…arrow_forwardGiven the provided class, please make the following modifications. class Employee: def __init__(self, name, number): self.name = name self.number = number class ProductionWorker(Employee): def __init__(self, name, number, shift_number, employee_number): Employee.__init__(self, name, number) self.shift_number = shift_number self.employee_number = employee_number # Create an instance of the ProductionWorker class and test itemployee1 = ProductionWorker("John Doe", 1234, 2, 5678)print(employee1.name)print(employee1.number)print(employee1.shift_number)print(employee1.employee_number) Write a class that creates an object of the ProductionWorker class and prompts the user to enter data for each of the object's data attributes. Store the data in the object, then use the object's accessor methods to retrieve it and display it on the screen.arrow_forward
- 7.23 LAB: Product class use Java. Given main(), define the Product class (in file Product.java) that will manage product inventory. Product class has three private member fields: a product code (String), the product's price (double), and the number count of product in inventory (int). Implement the following Constructor and member methods: public Product(String code, double price, int count) - set the member fields using the three parameters public void setCode(String code) - set the product code (i.e. SKU234) to parameter code public String getCode() - return the product code public void setPrice(double p) - set the price to parameter p public double getPrice() - return the price public void setCount(int num) - set the number of items in inventory to parameter num public int getCount() - return the count public void addInventory(int amt) - increase inventory by parameter amt public void sellInventory(int amt) - decrease inventory by parameter amt Ex. If a new Product object is…arrow_forwardhighlight the correct answer and provide proper explanation 6. A class should take any number as an argument but no other data types. Which code does this? a. public class xyz <T> b. public class xyz <T extends Number> c. public class xyz <T implements Number> d. public class xyz <T extends Integer> 7. Log4j.properties is set to 'warn'. Which would show in the log file? a. Info b. Debug c. Trace d. Error 8. Which would lock an object so that it could only be used by a single thread? a. join() b. yield() c. synchronized() d. start() 9. Which of the following is an example of a dependency between 2 objects? a. Vehicle and car b. Person and employee c. Pilot and plane d. Generic database reader and Oracle database readerarrow_forward13.5 LAB: Zip code and population (class templates) Define a class StatePair with two template types (T1 and T2), constructors, mutators, accessors, and a PrintInfo() method. Three vectors have been pre-filled with StatePair data in main(): * vector> zipCodeState: ZIP code - state abbreviation pairs * vector> abbrevState: state abbreviation - state name pairs * vector> statePopulation: state name - population pairs Complete main() to use an input ZIP code to retrieve the correct state abbreviation from the vector zipCodeState. Then use the state abbreviation to retrieve the state name from the vector abbrevState. Lastly, use the state name to retrieve the correct state name/population pair from the vector statePopulation and output the pair. Ex: If the input is: 21044 the output is: Maryland: 6079602 Main.cpp: #include #include #include #include #include "StatePair.h" using namespace std; int main() { ifstream inFS; // File input stream int zip; int…arrow_forward
- 13.5 LAB: Zip code and population (class templates) Define a class StatePair with two template types (T1 and T2), constructors, mutators, accessors, and a PrintInfo() method. Three vectors have been pre-filled with StatePair data in main(): * vector> zipCodeState: ZIP code - state abbreviation pairs * vector> abbrevState: state abbreviation - state name pairs * vector> statePopulation: state name - population pairs Complete main() to use an input ZIP code to retrieve the correct state abbreviation from the vector zipCodeState. Then use the state abbreviation to retrieve the state name from the vector abbrevState. Lastly, use the state name to retrieve the correct state name/population pair from the vector statePopulation and output the pair. Ex: If the input is: 21044 the output is: Maryland: 6079602 I put the TODO in bold which are the ones I need help with. main.cpp: #include #include #include #include #include "StatePair.h" using namespace std; int main()…arrow_forwardCreate a class called Line with the followings:1. Private members: p1 and p2 as pointer to Point objects (code provided below), slope and length as double variables2. Define setter and getter functions.3. Define a default constructor that allocate dynamic memory for points andset everything to 0.4. Overload a constructor that allocates memory for points, initilize themwith given arguments, and calculate the slope and length.5. Overload a destructor, a copy constructor and a copy assignment operator.6. Create a function called ”parallel” that return true when given lines areparallel and returns false otherwise7. Overload the less than (<) and greater than (>) and equality (==) oper-ators (compare the length)8. Write a functions that reads lines in the format provided in the lines.txtfrom the file and stores them in a vector named Lines.9. Sort the objects of Lines vector in descending order.10. Extend the functionality of cin and cout for this class11. Write a separate file to…arrow_forward[2/25, 05:28] Vishal Singh Rawat: Kindly store employee details using class with python program [2/25, 05:28] Vishal Singh Rawat: There are 4 employees Details of all employee: Name: John , Designation: Manager , Salary: 80000 Name: Mike , Designation: Team Leader , Salary: 50000 Name: Derek , Designation: Programmer , Salary: 30000 Designation: Assistant , Salary: 25000 Name: Rajarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
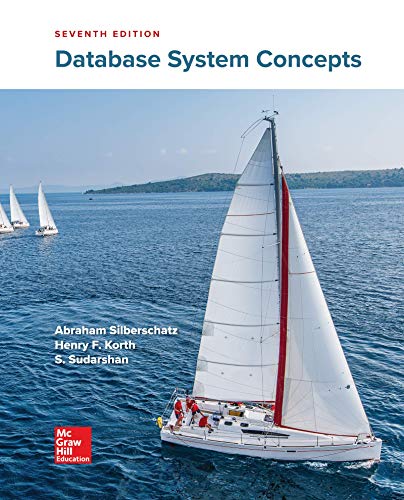
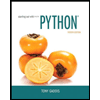
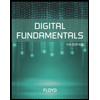
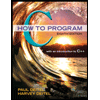
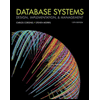
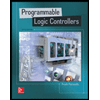